5 Tips to Boost Your Django App Performance and Keep Your Users Happy
Django is an incredibly powerful web framework, but it is important to keep your app running smoothly and quickly. Poor performance can lead to user frustration, so here are 5 tips to help you boost the performance of your Django app and keep your users happy.
1. Optimize Your Database Queries
The most important way to improve the performance of your Django app is to optimize your database queries. One way to do this is to use the select_related
method to reduce the number of queries that Django needs to make. This method will return related objects in a single query, instead of making multiple queries. For example:
# without select_related
blog_posts = BlogPost.objects.all()
for post in blog_posts:
comments = post.comments.all()
# with select_related
blog_posts = BlogPost.objects.select_related('comments').all()
for post in blog_posts:
comments = post.comments.all()
2. Use Caching
Caching is a great way to improve the performance of your Django app. Django has an excellent caching framework that allows you to easily cache data in memory, on disk, or in a database. For example, you can use the @cache_page
decorator to cache the results of a view:
@cache_page(60 * 15)
def my_view(request):
# ...
3. Use Template Caching
Another way to improve performance is to use template caching. Django has an excellent template caching framework that allows you to cache the results of a template for a given time period. For example, you can use the @cache_page
decorator to cache the results of a template:
@cache_page(60 * 15)
def my_template(request):
rendered_template = render_to_string('my_template.html', {'foo': 'bar'})
return HttpResponse(rendered_template)
4. Use a Web Server
Using a web server to serve your Django app can drastically improve performance. Popular web servers such as Nginx and Apache are designed to handle large amounts of traffic and can handle requests more efficiently than Django.
5. Use a CDN
Using a Content Delivery Network (CDN) is another great way to improve performance. A CDN is a network of servers located around the world that can cache static files and serve them to users quickly. Popular CDNs include CloudFlare and Amazon CloudFront.
By following these 5 tips, you can dramatically improve the performance of your Django app and keep your users happy.
Recent Posts
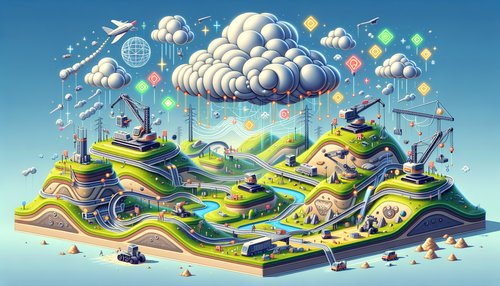
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
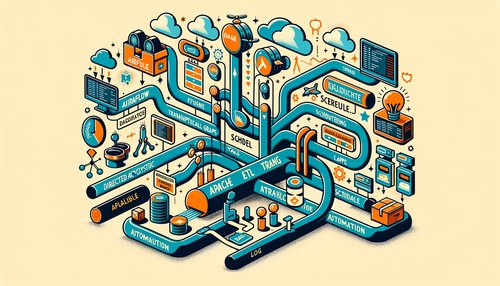
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow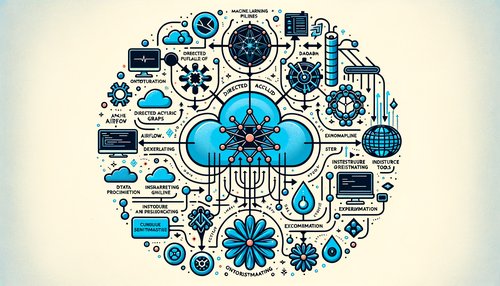
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow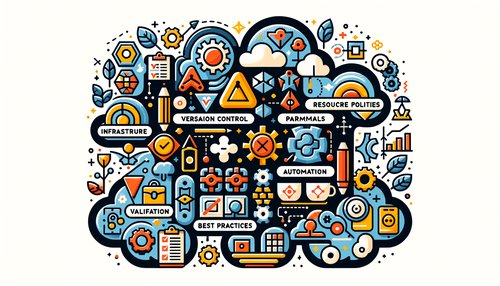
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
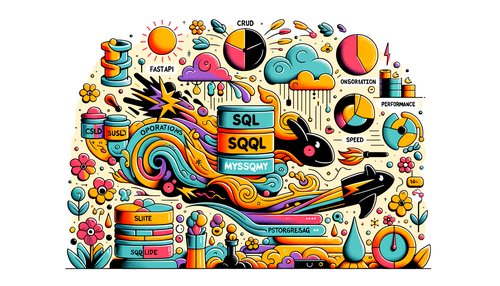