Beginner's Blueprint: Navigating Your First Steps in Secure App Development with FastAPI
Welcome to the exciting world of app development with FastAPI! If you're taking your first steps into this domain, you've chosen a powerful and efficient tool to build your applications. However, with great power comes great responsibility, especially when it comes to security. This post is designed to be your go-to guide, helping you navigate through the basics of secure app development with FastAPI. We'll cover essential concepts, practical tips, and some best practices to ensure your application is not only fast and efficient but also secure from the get-go.
Understanding FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. The key features that make FastAPI stand out are its speed, ease of use, and robustness. It's designed to be easy to use, with a simple syntax that's highly expressive. But before diving into the development, it's crucial to grasp the importance of securing your application.
Why Security Matters
In the digital age, security is paramount. A single vulnerability in your application can lead to data breaches, unauthorized access, and various cyber attacks. These incidents can tarnish your reputation, lead to financial losses, and even legal consequences. Hence, embedding security into your app development process from the start is not just recommended; it's essential.
Setting Up a Secure Foundation
Starting with a secure foundation is critical. Here are some steps to ensure this:
- Use a Virtual Environment: Always work within a virtual environment to manage dependencies safely and avoid conflicts between packages.
- Dependency Management: Regularly update your dependencies to mitigate vulnerabilities from outdated packages.
- Environment Variables: Store sensitive information like database URLs, secret keys, etc., in environment variables, not in your code.
Authentication and Authorization
Controlling who gets access to what is a fundamental aspect of securing your application. FastAPI provides tools to help with this:
- OAuth2: Implement OAuth2 with Password (and hashing), including token expiry, to manage user authentication securely.
- Permissions: Use FastAPI's dependency system to create reusable dependencies that control access at the operation level.
Remember, proper authentication and authorization mechanisms are your first line of defense against unauthorized access.
Data Validation and Serialization
Validating incoming data is crucial to protect your application from malicious input. FastAPI makes validation straightforward:
- Pydantic Models: Use Pydantic models to define the expected data shape, automatically validating data against it.
- SQL Injection Protection: FastAPI's ORM tools (like SQLAlchemy) automatically protect against SQL injection attacks when used correctly.
HTTPS Everywhere
Securing your data in transit is just as important as securing it at rest. Always use HTTPS to encrypt data between your server and your clients. This prevents man-in-the-middle attacks and ensures that sensitive information is transmitted securely.
Rate Limiting
Protect your API from abuse and overuse by implementing rate limiting. This prevents bad actors from overwhelming your server with excessive requests. FastAPI doesn't include rate limiting out of the box, but you can integrate it with tools like Traefik or use third-party libraries.
Monitoring and Logging
Keeping an eye on your application is vital. Implement logging to track down issues and monitor your application's health. FastAPI supports logging, but it's up to you to configure it to suit your needs. Monitor logs for unusual activity that could indicate a security issue.
Conclusion
Securing your FastAPI application is an ongoing process that begins the moment you start coding. By setting up a secure foundation, implementing robust authentication and authorization, validating and serializing data correctly, enforcing HTTPS, rate limiting, and monitoring your application, you can build a secure and reliable application. Remember, security is not a one-time task but a continuous effort. Keep learning, updating, and testing your application to stay ahead of potential threats.
Embarking on your journey in secure app development with FastAPI is exciting. With this beginner's blueprint, you have the knowledge to start building secure, efficient, and powerful applications. Happy coding, and remember, security first!
Recent Posts
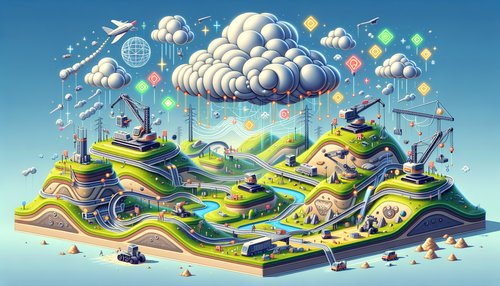
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
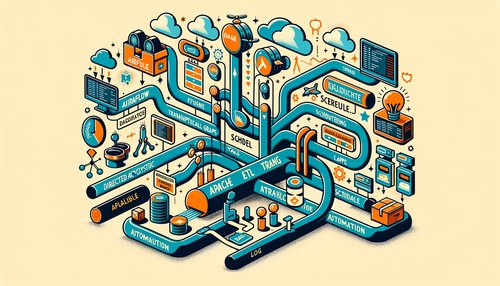
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow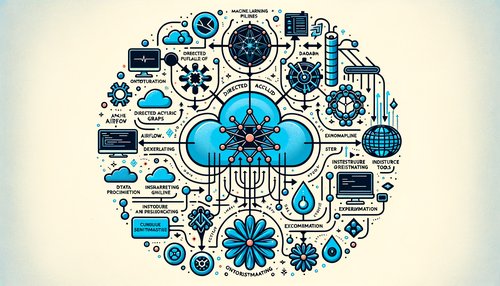
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow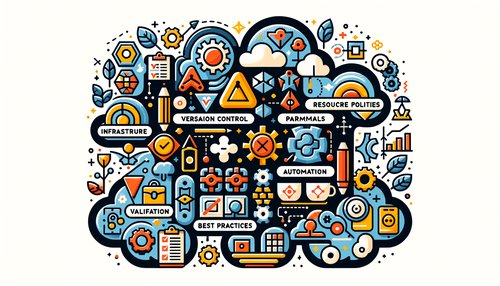
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
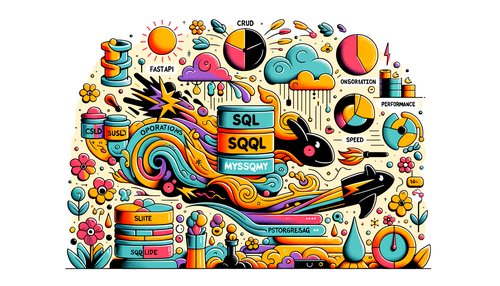