Mastering Dynamic Forms: The Ultimate Guide to Elevating Your Web Projects with React Hook Form
Welcome to the comprehensive guide on elevating your web projects by mastering dynamic forms using React Hook Form. In the ever-evolving landscape of web development, the ability to create efficient, scalable, and user-friendly forms is crucial. Whether you're building a simple contact form or a complex multi-step application process, React Hook Form offers a powerful and flexible solution that can significantly enhance your project's functionality and user experience. In this guide, we'll dive deep into the world of dynamic forms, exploring the features, benefits, and practical applications of React Hook Form. Get ready to transform your web projects with the insights and tips you'll find here!
Understanding React Hook Form
Before we delve into the specifics of dynamic forms, let's first understand what React Hook Form is and why it's a game-changer for developers. React Hook Form is a minimal library that simplifies the process of working with forms in React applications. It leverages React hooks to create a seamless, performant way to build and manage forms without the cumbersome boilerplate code that often accompanies form handling. The key features that set React Hook Form apart include its performance optimization, easy validation, and minimal re-renders, making it an optimal choice for developers looking to create sophisticated form-based interfaces efficiently.
Getting Started with React Hook Form
Integrating React Hook Form into your project is straightforward. You begin by installing the library via npm or yarn, and then you can immediately start using it within your components. A basic implementation involves using the useForm
hook to create form controls, which can then be easily connected to your form elements. This section will guide you through the initial setup and show you how to create a simple form with validation, providing a solid foundation for more complex scenarios.
Installation
npm install react-hook-form
Basic Form Example
import React from 'react';
import { useForm } from 'react-hook-form';
function BasicForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = data => console.log(data);
return (
);
}
Creating Dynamic Forms
One of the most powerful features of React Hook Form is its ability to handle dynamic forms with ease. Dynamic forms are forms that change based on user input, such as adding or removing fields on the fly. This functionality is crucial for creating interactive, user-friendly interfaces. In this section, we'll explore how to leverage React Hook Form to implement dynamic form fields, including practical examples and best practices for managing complex form structures.
Example: Adding and Removing Fields
Let's consider a scenario where you need to allow users to add multiple email addresses within a form. React Hook Form simplifies this process by using the useFieldArray
hook, which provides methods for adding, removing, and updating array-based fields. Here's how you can implement this functionality:
import React from 'react';
import { useForm, useFieldArray } from 'react-hook-form';
function DynamicForm() {
const { register, control, handleSubmit } = useForm();
const { fields, append, remove } = useFieldArray({
control,
name: "emails"
});
const onSubmit = data => console.log(data);
return (
);
}
Advanced Techniques and Best Practices
As you become more familiar with React Hook Form, you can explore advanced techniques and best practices to further enhance your form handling. This includes efficient data validation using external libraries like Yup, optimizing form performance by minimizing re-renders, and implementing complex form patterns such as conditional fields and multi-step forms. By mastering these advanced concepts, you can take your web projects to the next level, creating highly interactive and user-friendly forms that stand out.
Conclusion
In this guide, we've covered the essentials of mastering dynamic forms with React Hook Form, from understanding the basics to implementing advanced techniques. React Hook Form is a powerful tool that can dramatically improve the way you handle forms in your web projects, offering efficiency, flexibility, and a great user experience. By following the insights and tips provided in this guide, you're now well-equipped to elevate your web projects with sophisticated, dynamic forms. Remember, the key to mastering any technology is practice and experimentation, so don't hesitate to explore further and push the boundaries of what you can achieve with React Hook Form.
Happy coding!
Recent Posts
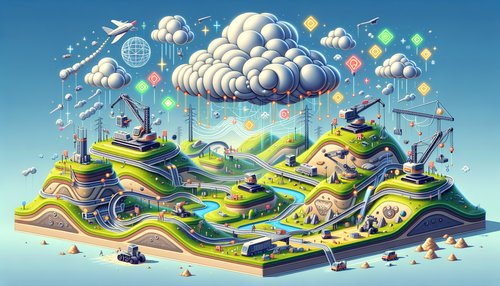
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
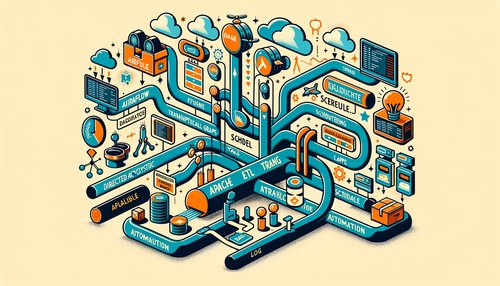
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow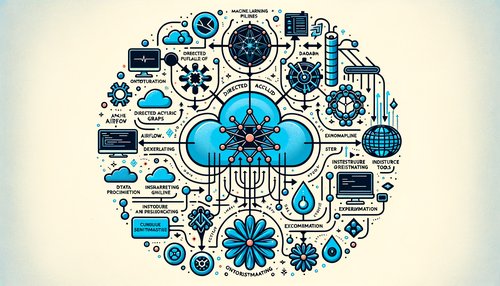
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow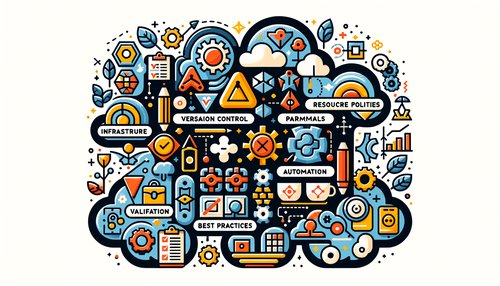
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
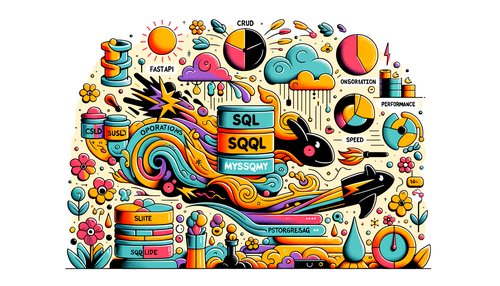