Mastering FastAPI: A Deep Dive into Leveraging Header Parameters for Enhanced User Guides
Welcome to an in-depth exploration of how FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ types, enables developers to leverage header parameters to create more robust and user-friendly APIs. Whether you're a seasoned developer or new to the world of API development, understanding how to effectively use header parameters in FastAPI can significantly enhance the functionality and user experience of your applications. This blog post will guide you through the essentials of header parameters in FastAPI, providing practical tips, examples, and insights to help you master their use in your projects.
Understanding Header Parameters in FastAPI
Before diving into the specifics of leveraging header parameters, it's crucial to understand what they are and why they're important. Header parameters are components of the HTTP request header that can be used to pass additional information about the request or the client itself to the server. They play a vital role in controlling the behavior of both the request and the response, influencing aspects such as content type, authentication, caching, and more.
In FastAPI, header parameters are defined using Pydantic models or standard Python data types, and they are easy to declare and utilize within your API endpoints. This flexibility and ease of use make FastAPI an attractive choice for developers looking to build efficient and scalable web applications.
Declaring Header Parameters in FastAPI
Declaring header parameters in FastAPI is straightforward. You can define them in your route functions using the Header
class from FastAPI. This class allows you to set default values, make the header required or optional, and include additional validation constraints. Here's a simple example:
from fastapi import FastAPI, Header app = FastAPI() @app.get("/items/") async def read_items(user_agent: str = Header(None)): return {"User-Agent": user_agent}
In this example, the user_agent
header parameter is extracted from the request header and returned in the response. This demonstrates how easy it is to access header information in FastAPI.
Practical Uses of Header Parameters
Header parameters can be used for a variety of purposes in web development. Some common use cases include:
- Authentication: API keys and JWT tokens can be passed in headers to authenticate requests.
- Content Negotiation: The
Accept
header can be used to specify the desired response format (e.g., JSON, XML). - Rate Limiting: Custom headers can be used to communicate rate limiting policies and remaining quotas to clients.
- Localization: Headers like
Accept-Language
can tailor responses based on the user's language preference.
These examples illustrate the versatility of header parameters in enhancing API functionality and improving the user experience.
Best Practices for Using Header Parameters
While header parameters are powerful tools, it's important to use them wisely. Here are some best practices to consider:
- Be mindful of security: Avoid passing sensitive information in headers unless it's encrypted or secured.
- Use standard headers when possible: Adhering to standard headers for common tasks ensures compatibility and predictability.
- Document your headers: Clearly document the headers your API uses, including required formats and example values, to facilitate easier integration for developers.
Conclusion
Leveraging header parameters in FastAPI allows developers to build more flexible, efficient, and user-friendly APIs. By understanding how to declare and use header parameters, implementing practical use cases, and following best practices, you can enhance the capabilities of your web applications. Remember, the key to mastering FastAPI and its features lies in experimentation and continuous learning. So, dive in, start coding, and unlock the full potential of header parameters in your next project!
Whether you're developing complex APIs or simple web services, incorporating header parameters effectively can lead to more secure, scalable, and sophisticated applications. Embrace the power of FastAPI and its support for header parameters to elevate your web development skills to new heights.
Recent Posts
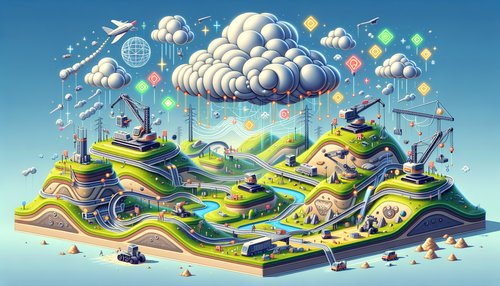
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
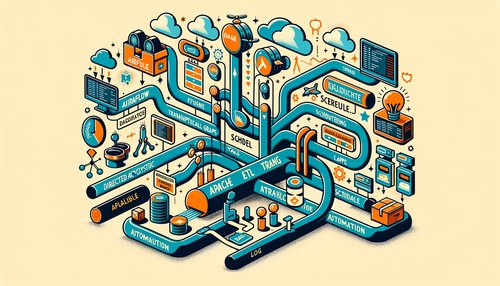
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow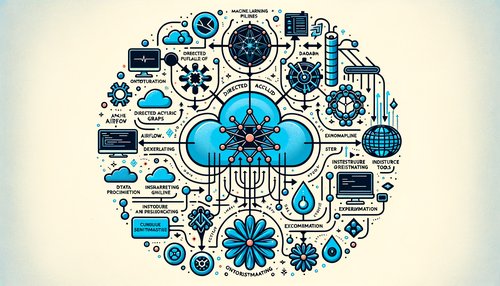
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow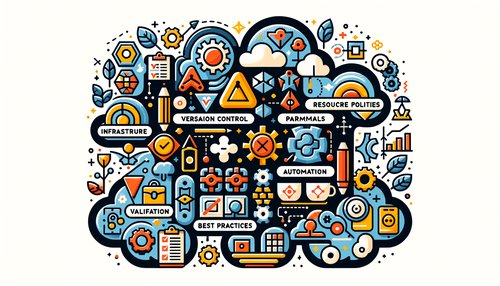
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
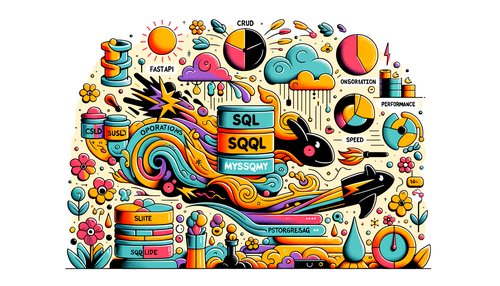