Mastering Form Data with FastAPI: A User's Ultimate Guide to Efficient Web Development
Welcome to the world of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. The speed of FastAPI, intuitive design, and scalability has made it a popular choice among developers for creating robust APIs efficiently. In this comprehensive guide, we delve into the specifics of handling form data with FastAPI, ensuring your web development process is not just efficient, but seamless and powerful. From understanding the basics of form data and FastAPI to implementing advanced techniques for data validation and file handling, this guide has got you covered.
Understanding Form Data in Web Development
Form data represents the information that users input into forms on web pages. It's a critical component of modern web applications, allowing users to register, login, submit personal details, and much more. Handling form data accurately and securely is essential for any web application's functionality and user experience. FastAPI provides a straightforward and effective way to handle form data, leveraging Python's type hints for validation and error handling right out of the box.
Getting Started with FastAPI and Form Data
Before diving into the specifics, ensure you have FastAPI and Uvicorn (an ASGI server) installed in your environment. FastAPI's design allows you to define your form fields similarly to how you define query parameters and request bodies, making it incredibly intuitive for developers who are already familiar with the framework.
pip install fastapi uvicorn
Here's a simple example to get us started:
from fastapi import FastAPI, Form
app = FastAPI()
@app.post("/login/")
async def login(username: str = Form(...), password: str = Form(...)):
return {"username": username}
This example demonstrates how to create a simple login form with FastAPI, capturing the username and password from form data.
Advanced Form Data Handling
Moving beyond the basics, FastAPI allows for more complex data handling scenarios, including validations, pre-processing of form data, and file uploads. Utilizing Pydantic models, FastAPI can validate form data against a predefined schema, ensuring the data integrity and security of your application.
For example, to validate that a password meets certain criteria (e.g., minimum length), you could use the following approach:
from pydantic import BaseModel, Field
class LoginForm(BaseModel):
username: str
password: str = Field(..., min_length=8)
@app.post("/login/")
async def login(form_data: LoginForm = Form(...)):
return {"username": form_data.username}
This leverages Pydantic's Field
function to specify that the password must be at least 8 characters long, adding an extra layer of validation to your form handling.
Handling File Uploads
File uploads are another aspect of form data that FastAPI simplifies. By combining form field definitions with FastAPI's File
and UploadFile
types, you can easily handle file uploads in your applications.
from fastapi import FastAPI, File, UploadFile
@app.post("/uploadfile/")
async def create_upload_file(file: UploadFile = File(...)):
return {"filename": file.filename}
This snippet creates an endpoint that accepts file uploads, providing a straightforward way to handle files in your FastAPI applications.
Conclusion
Handling form data efficiently is crucial for the development of robust web applications. FastAPI provides developers with the tools and flexibility needed to manage form data effectively, including built-in validation, file handling, and more. By leveraging FastAPI's capabilities, developers can ensure that their applications are not only functional but also secure and user-friendly. Remember, the key to mastering form data with FastAPI lies in understanding the basics, applying best practices, and continually experimenting with the framework's advanced features. So, take this guide as a starting point and dive deeper into the fascinating world of efficient web development with FastAPI.
As you embark on your journey to master form data handling with FastAPI, keep experimenting, keep learning, and most importantly, keep building amazing applications.
Recent Posts
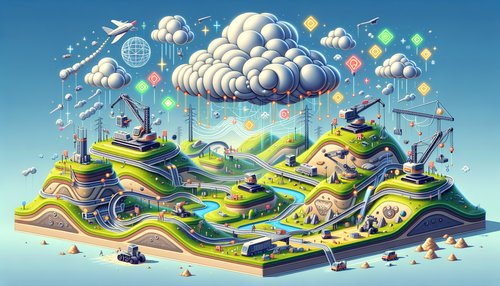
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
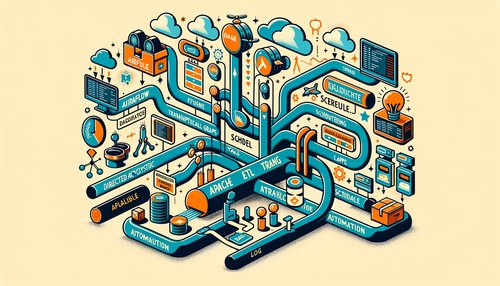
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow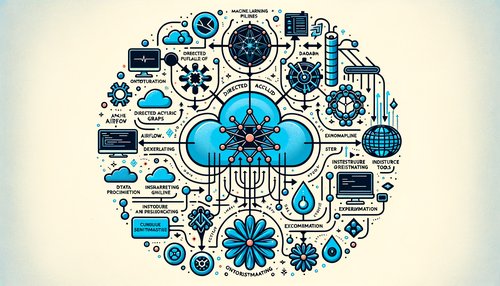
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow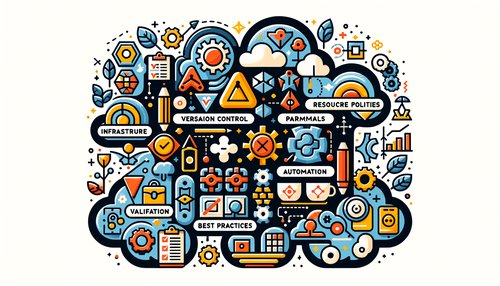
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
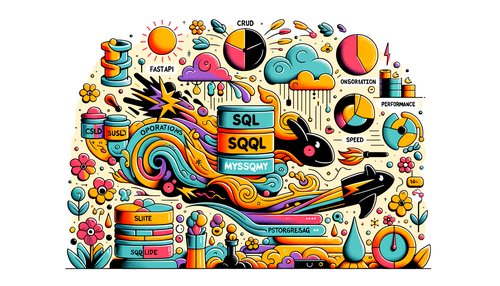