Mastering the Web: Unleashing the Full Power of Django REST API for Seamless Application Integration
When it comes to web development, the ability to seamlessly integrate various applications is paramount. This is where Django, a high-level Python web framework, shines, especially with its powerful toolkit for building Web APIs - Django REST framework (DRF).
The Django REST framework is a flexible toolkit that helps you build Web APIs that can cater to a multitude of clients, including browsers and mobile devices. By mastering Django REST API, developers can create robust, scalable, and secure web applications. Let's dive into the core aspects of utilizing Django REST framework to its full potential.
Setting Up Your Environment
Before you begin, ensure you have Python and Django installed on your system. Then, install Django REST framework:
pip install djangorestframework
Add 'rest_framework' to your Django project's settings 'INSTALLED_APPS' like so:
INSTALLED_APPS = [
...
'rest_framework',
]
Creating a Simple API
Let's create a basic API for managing a list of items. First, define your model in models.py
:
from django.db import models
class Item(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
Next, create a serializer for your model in serializers.py
:
from rest_framework import serializers
from .models import Item
class ItemSerializer(serializers.ModelSerializer):
class Meta:
model = Item
fields = ['id', 'name', 'description']
Now, set up your views in views.py
:
from rest_framework import generics
from .models import Item
from .serializers import ItemSerializer
class ItemListCreate(generics.ListCreateAPIView):
queryset = Item.objects.all()
serializer_class = ItemSerializer
Finally, wire up the URLs in urls.py
:
from django.urls import path
from .views import ItemListCreate
urlpatterns = [
path('items/', ItemListCreate.as_view(), name='item-list-create'),
]
At this point, you have a basic API endpoint that allows you to list and create items. If you run your server and navigate to /items/
, you should see your API in action.
Authentication & Permissions
Security is crucial for any API. Django REST framework offers various authentication and permission classes that you can use to protect your API. For example, to add Token Authentication, you would update your settings:
INSTALLED_APPS = [
...
'rest_framework.authtoken'
]
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.TokenAuthentication',
],
}
And then apply permissions in your views:
from rest_framework.permissions import IsAuthenticated
class ItemListCreate(generics.ListCreateAPIView):
permission_classes = [IsAuthenticated]
queryset = Item.objects.all()
serializer_class = ItemSerializer
With these settings, only authenticated users with valid tokens can access the API.
Optimizations and Best Practices
As your API grows, you'll want to ensure it remains efficient and maintainable. Here are some quick tips:
- Use viewsets and routers for concise code and automatic URL routing.
- Throttle requests to prevent abuse of your API.
- Employ caching to improve performance.
- Version your API to handle changes gracefully.
Conclusion
Django REST framework is a powerful tool that can help you create APIs that stand at the heart of modern web applications. By following the guidelines above, you can harness the full potential of Django REST API for seamless application integration. The combination of Django's robustness and REST framework's flexibility creates a web development powerhouse for both simple and complex applications alike.
Remember, the key to mastering Django REST API is practice and continuous learning. So dive in, build something amazing, and keep iterating. The web awaits your creations!
Recent Posts
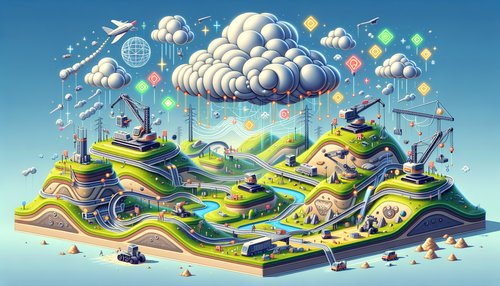
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
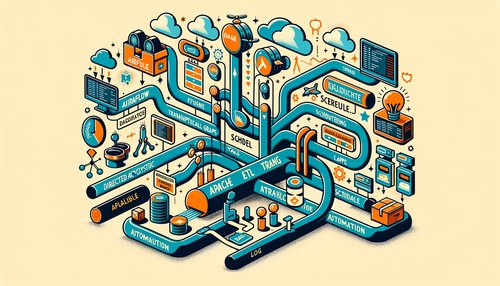
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow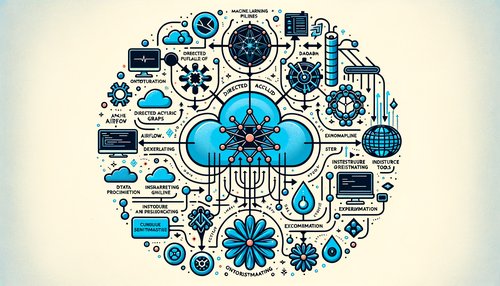
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow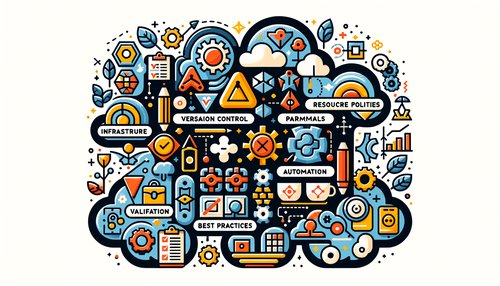
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
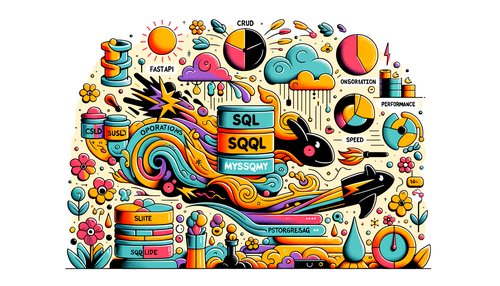