The Difference Between React and Angular
React and Angular are two of the most popular front-end web development frameworks. Although they both share similarities, they have some key differences that set them apart. In this blog post, we’ll go over the main differences between React and Angular and provide some code snippets to illustrate the differences.
React
React is a JavaScript library created by Facebook in 2013. It is primarily used for building user interfaces (UIs). React is a component-based framework, meaning it’s built around individual, reusable components. Each component has its own state, and can be reused in different places throughout the app.
React is lightweight and fast, and it uses a “virtual DOM” (Document Object Model). This means that React only updates the parts of the DOM that have changed, which makes it faster and more efficient than other frameworks.
Here’s a code snippet to show how you might create a simple React component:
class MyComponent extends React.Component {
render() {
return (
<div>
<h1>My Component</h1>
</div>
);
}
}
Angular
Angular is a TypeScript-based open-source web application framework. It was developed by Google and released in 2009. Angular is a full-featured framework, meaning it has everything you need to build a complete web application.
Angular uses a hierarchical structure of components, which makes it easier to create complex applications. It also supports two-way data binding, which makes it easier to keep your UI and data in sync.
Here’s a code snippet to show how you might create a simple Angular component:
@Component({
selector: 'my-component',
template: `<h1>My Component</h1>`
})
export class MyComponent { }
Conclusion
As you can see, React and Angular have some key differences. React is a lightweight library, while Angular is a full-featured framework. React uses components and a virtual DOM, while Angular uses hierarchical components and two-way data binding.
Ultimately, the decision of which framework to use will depend on the needs of your project. If you’re looking for a lightweight solution, React might be the right choice. If you need a full-featured framework, Angular might be a better option.
Recent Posts
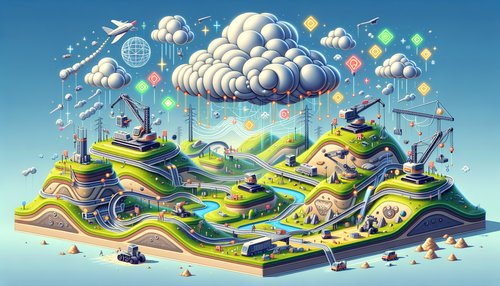
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
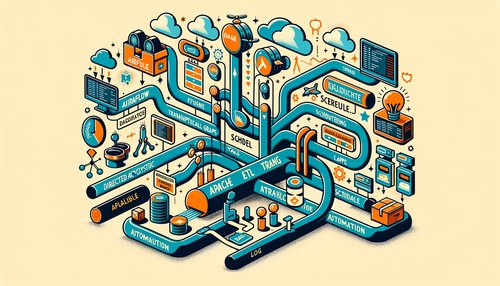
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow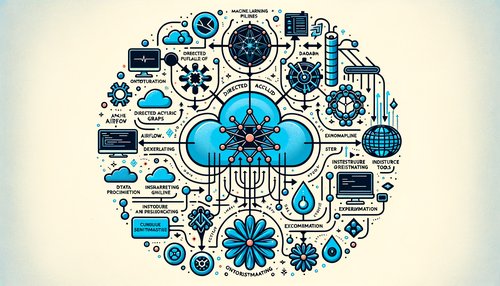
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow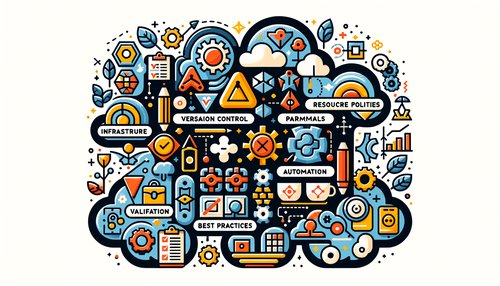
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
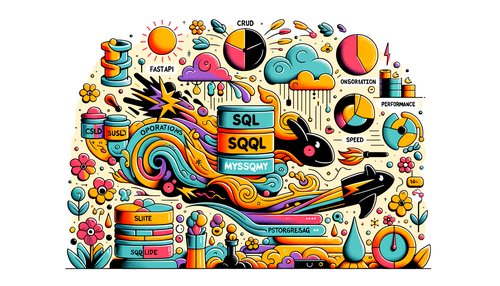