Mastering the Art of Time with Pandas: A Deep Dive into Windowing Operations
Welcome to a journey through the intricate and powerful world of windowing operations with Pandas, the go-to Python library for data manipulation and analysis. If you've ever found yourself wrestling with time series data or seeking ways to analyze trends over intervals, you're in the right place. This blog post will guide you through the art of leveraging windowing operations in Pandas to extract insights from your data efficiently and effectively. From understanding the basics to exploring advanced techniques, we'll cover everything you need to transform your data analysis skills. Get ready to unlock new potentials in your datasets!
What Are Windowing Operations?
Before diving into the technicalities, let's demystify what windowing operations are. In essence, windowing operations allow you to perform calculations over a sliding window of data points, making them indispensable for time series analysis. These operations can be categorized into two main types: rolling and expanding windows. Rolling windows move with your data, considering a fixed-size segment at a time, while expanding windows grow as your data does, including all previous data points in the calculation. Understanding these operations is key to mastering time series analysis in Pandas.
Getting Started with Rolling Windows
Rolling windows are your first step into the world of windowing operations. Using the .rolling()
method in Pandas, you can easily apply functions like mean, median, sum, and more over a specified period. This is particularly useful for smoothing out short-term fluctuations and highlighting longer-term trends in your data.
import pandas as pd
# Sample time series data
data = pd.Series([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Apply a rolling window of size 3
rolling_window = data.rolling(window=3)
rolling_mean = rolling_window.mean()
print(rolling_mean)
This simple example illustrates how to calculate the rolling mean over a window of three observations. Experimenting with different window sizes and functions can provide deeper insights into your data's behavior.
Expanding Your Horizons with Expanding Windows
While rolling windows offer a snapshot of your data over fixed intervals, expanding windows provide a cumulative perspective. The .expanding()
method in Pandas enables you to apply functions that consider all preceding data points. This approach is useful for understanding the overall direction and stability of your data over time.
import pandas as pd
# Sample time series data
data = pd.Series([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Apply an expanding window
expanding_window = data.expanding()
expanding_sum = expanding_window.sum()
print(expanding_sum)
This example demonstrates how to calculate the cumulative sum of a dataset. Expanding windows can also be used to compute metrics like cumulative averages, variances, and more, providing a broad view of your data's trends.
Advanced Techniques: Exponential Weighted Windows
For those looking to delve deeper, exponential weighted windows offer a more sophisticated approach to windowing operations. These windows assign exponentially decreasing weights to older observations, making them particularly useful for datasets where more recent observations are more relevant. Pandas supports this through the .ewm()
method, allowing for nuanced control over the decay factor to fine-tune your analysis.
import pandas as pd
# Sample time series data
data = pd.Series([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Apply an exponential weighted window
ewm_window = data.ewm(span=3)
ewm_mean = ewm_window.mean()
print(ewm_mean)
This example calculates the exponential weighted mean with a span of 3, showcasing how to prioritize more recent data points in your analysis.
Practical Tips for Effective Windowing
- Choose the right window size: The window size significantly impacts your analysis. Too small, and you might miss broader trends; too large, and you could smooth over important details. Experiment with different sizes to find the best fit for your data.
- Understand your data: The nature of your data should guide your choice of windowing operation. Consider whether your data has seasonality, trends, or irregular patterns that might affect your analysis.
- Combine window types: Don't be afraid to mix and match window types. For instance, you might use rolling windows for smoothing and expanding windows for cumulative metrics to gain a comprehensive view of your data.
Conclusion
Windowing operations in Pandas offer a powerful toolkit for time series analysis, allowing you to uncover patterns, trends, and insights in your data that might otherwise remain hidden. By mastering rolling, expanding, and exponential weighted windows, you can enhance your data analysis skills and make more informed decisions. Remember, the key to effective windowing is understanding your data and experimenting with different techniques to see what works best. Happy analyzing!
As you continue to explore the capabilities of Pandas and windowing operations, consider the impact of your findings on your projects and how you can apply these techniques to solve real-world problems. The art of time series analysis is a journey of discovery, and with Pandas, you have a powerful companion on your path to mastery.
Recent Posts
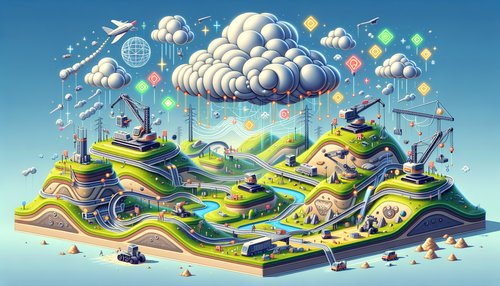
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
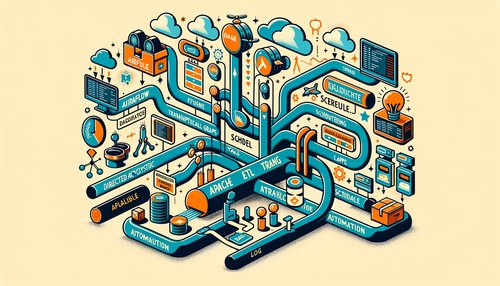
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow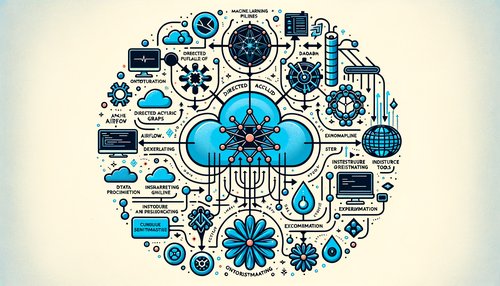
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow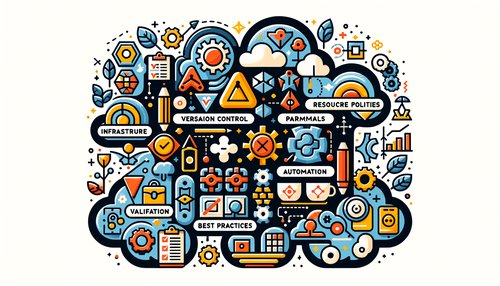
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
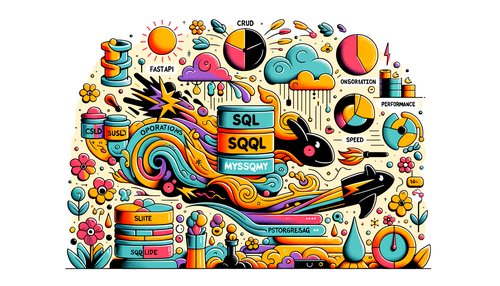