Unlocking the Power of Django Forms: A Comprehensive Guide to Streamlining Your Web Development
Django forms are one of the most powerful tools available to web developers looking to create interactive web applications. Django forms provide an easy way to create and manage user input, and can be used to construct complex data processing pipelines. In this comprehensive guide, we will explore the fundamentals of Django forms, and discuss strategies for streamlining your web development process.
Using Form Classes
At the core of Django forms is the Form
class. This class is responsible for rendering HTML forms and collecting user input. To create a form, you must first define a Form
class and add the desired fields. For example:
from django import forms
class ContactForm(forms.Form):
name = forms.CharField(max_length=100)
email = forms.EmailField()
message = forms.CharField(widget=forms.Textarea)
This example creates a ContactForm
class with three fields: name
, email
, and message
. The max_length
parameter of the CharField
class specifies the maximum number of characters the field can accept. The widget
parameter of the CharField
class specifies the HTML element used to render the field. In this case, we are using a Textarea
element, which allows users to enter multi-line text.
Validating User Input
Django forms also provide a way to validate user input. To do this, we must define a clean()
method for our form class. This method is responsible for checking the validity of the data entered by the user and raising an exception if it fails to meet the requirements. For example:
def clean(self):
cleaned_data = super().clean()
name = cleaned_data.get('name')
email = cleaned_data.get('email')
message = cleaned_data.get('message')
if not name and not email and not message:
raise forms.ValidationError('You must enter a name, email, and message.')
This clean()
method checks to make sure the user has entered a name, email, and message. If any of these fields are blank, it raises a ValidationError
exception.
Rendering Forms in Templates
Once we have defined our form class, we can render it in a template using the {{ form }}
tag. This tag will generate the necessary HTML code for displaying the form. For example:
<form action="{% url 'contact' %}" method="post">
{% csrf_token %}
{{ form }}
<button type="submit">Submit</button>
</form>
This code will render a HTML form with the fields defined in our ContactForm
class. The {{ csrf_token }}
tag is necessary for protecting the form from Cross-Site Request Forgery attacks.
Processing Form Data
Once the form has been submitted, we must process the data entered by the user. To do this, we must define a view that will handle the form submission. For example:
from django.shortcuts import render
def contact_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# process form data
return render(request, 'contact_success.html')
else:
form = ContactForm()
return render(request, 'contact.html', {'form': form})
This view first checks if the request method is POST
. If it is, it creates a ContactForm
instance with the submitted data and checks if it is valid. If the form is valid, we can process the data. Otherwise, we render the form for the user to try again.
Conclusion
Django forms are powerful tools for streamlining web development. By leveraging the capabilities of the Form
class, you can easily create and manage user input, and validate user data. Additionally, Django forms provide an easy way to render HTML forms in templates and process form data in views. By following this comprehensive guide, you will be able to unlock the power of Django forms and streamline your web development process.
Recent Posts
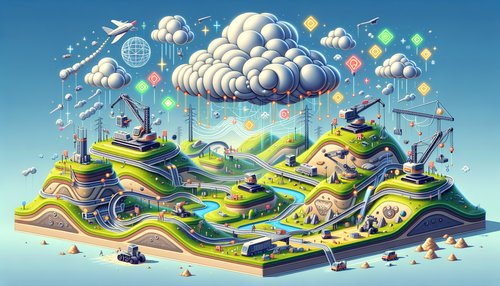
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
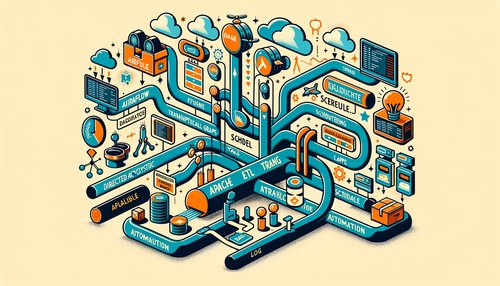
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow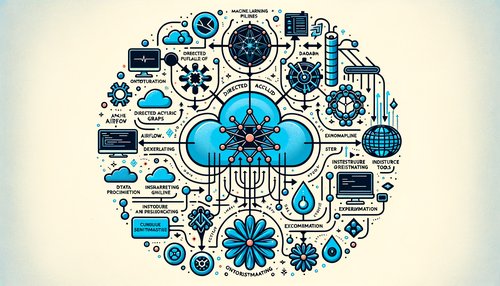
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow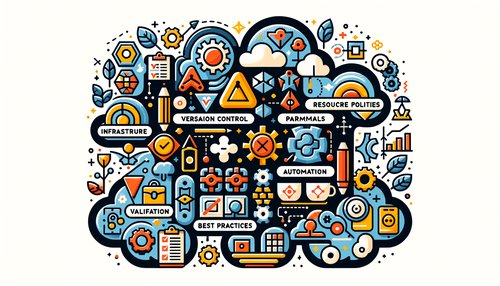
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
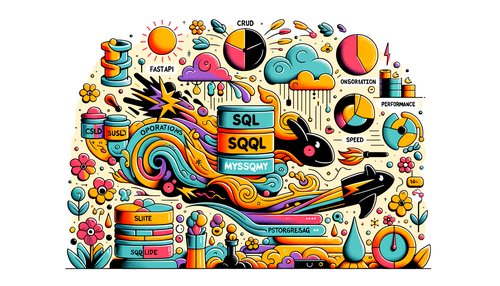