Unlocking Efficiency: Master the Art of Authoring and Scheduling with Apache Airflow
Welcome to the world of workflow automation, where the effective management of data pipelines can significantly enhance productivity and efficiency. Apache Airflow stands out as a powerful tool in this realm, offering robust capabilities for scheduling, orchestrating, and monitoring workflows. In this comprehensive guide, we will delve into the nuances of authoring and scheduling with Apache Airflow, providing practical tips, examples, and insights to help you master this art.
Understanding Apache Airflow
Before diving into the specifics of authoring and scheduling, let's briefly touch upon what Apache Airflow is and why it's a game-changer for workflow automation. Apache Airflow is an open-source platform designed to programmatically author, schedule, and monitor workflows. With its scalable, dynamic, extensible architecture, Airflow enables developers and data engineers to define workflows as code, which promotes modularity, reusability, and versioning.
Authoring Workflows with DAGs
At the heart of Apache Airflow are Directed Acyclic Graphs (DAGs), which represent a collection of all the tasks you want to run, organized in a way that reflects their relationships and dependencies. Authoring a DAG involves defining the tasks and their sequence, making it crucial to understand the basics of Python coding, as DAGs are written in Python.
Practical Tip: Start by sketching out your workflow on paper or a whiteboard. This visual representation will help you identify dependencies and organize tasks logically before translating them into code.
Here's a simple example of a DAG that performs two tasks:
from airflow import DAG from airflow.operators.dummy_operator import DummyOperator from datetime import datetime default_args = { 'owner': 'airflow', 'start_date': datetime(2023, 1, 1), } with DAG('example_dag', default_args=default_args, schedule_interval='@daily') as dag: start_task = DummyOperator(task_id='start') end_task = DummyOperator(task_id='end') start_task >> end_task
This code snippet illustrates a DAG named 'example_dag' that runs daily, starting with a 'start' task and followed by an 'end' task.
Scheduling and Managing Execution
Scheduling tasks is a critical aspect of workflow automation, determining when and how often tasks are executed. Apache Airflow provides a flexible way to schedule tasks using cron expressions or predefined presets (e.g., '@daily', '@hourly').
Insight: Leverage Airflow's rich UI to monitor and manage task execution. The UI offers a detailed view of DAG runs, task statuses, and logs, making it easier to debug and optimize workflows.
Advanced Features for Enhanced Productivity
Apache Airflow comes packed with advanced features that can significantly boost your productivity. Here are a few worth highlighting:
- Dynamic DAGs: Generate DAGs dynamically based on parameters or external data sources. This capability allows for more adaptable and responsive workflows.
- Branching: Use branching operators to run different tasks based on conditions, adding flexibility to your workflows.
- SubDAGs: Break down complex workflows into smaller, manageable pieces by defining subDAGs. This can improve readability and maintainability.
Example: Implementing a branching task based on a condition could look something like this:
from airflow.operators.python_operator import BranchPythonOperator def decide_which_path(): # Logic to choose path A or B if some_condition: return 'path_a' else: return 'path_b' branch_task = BranchPythonOperator( task_id='branching', python_callable=decide_which_path, dag=dag, )
Best Practices for Success
To maximize the benefits of Apache Airflow, consider these best practices:
- Keep your DAGs idempotent: Ensure that rerunning a DAG doesn't produce different results or cause failures.
- Test thoroughly: Make use of Airflow's testing framework to test your DAGs and tasks individually.
- Document your workflows: Use comments and documentation to make your DAGs easy to understand and maintain.
Conclusion
Mastering the art of authoring and scheduling with Apache Airflow can unlock unprecedented efficiency in managing workflows. By understanding the basics of DAGs, leveraging advanced features, and adhering to best practices, you can streamline your data pipelines and automate complex processes with confidence. Remember, the key to success lies in continuous learning and experimentation. So, dive in, start small, and gradually expand your Airflow mastery to harness the full potential of workflow automation.
Embrace the journey towards efficient workflow automation with Apache Airflow, and watch as it transforms your projects and processes, leading to improved productivity and outcomes.
Recent Posts
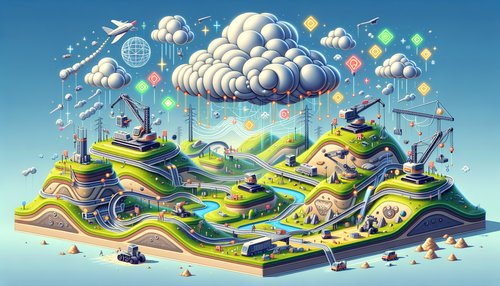
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
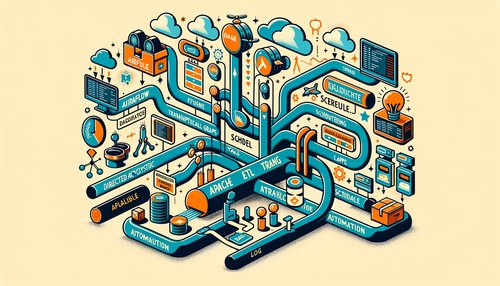
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow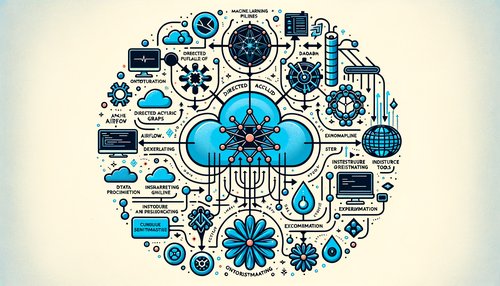
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow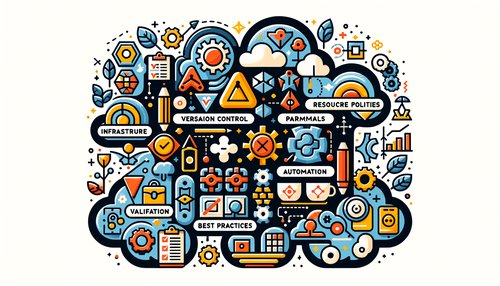
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
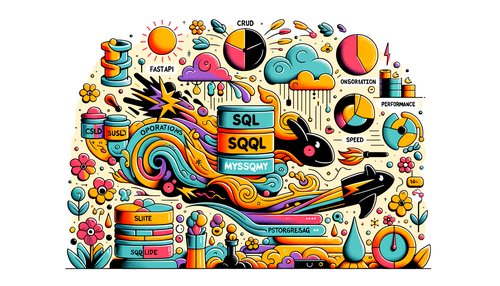