A Comprehensive Guide to Mastering Django Class-Based-Views
Django Class-Based-Views (CBVs) is a powerful way to organize and structure your web application. It is a high-level abstraction that allows developers to build complex web applications quickly and easily. With CBVs, you can build efficient, maintainable, and scalable web applications that are easy to debug and test.
In this guide, we’ll cover the basics of working with Django CBVs, including what they are, how to use them, and some useful tips and tricks. We’ll also look at some example code snippets that you can use to get started.
What are Django CBVs?
Django CBVs are a way of organizing web application code into reusable components. Instead of writing individual functions for each view, you can define a class with a set of methods that represent the different views in your application. This makes it easier to maintain and reuse code since each view can be defined in one place.
Django CBVs are based on the Model-View-Controller (MVC) pattern, where the “model” is the data, the “view” is the way it is presented to the user, and the “controller” is the logic that handles the requests and responses.
How to Use Django CBVs
When working with Django CBVs, you’ll need to define a class for each view. The class will have a set of methods that represent the different actions that can be taken on the view.
For example, if you have a view for displaying a list of blog posts, you could define a PostListView
class with methods for get()
, post()
, and delete()
. Each of these methods will be called when a request is made to the view.
You can also add additional methods to the class to add custom logic or handle specific requests. For example, you could add a search()
method to the PostListView
class to handle search requests.
Example Code Snippets
Here are some example code snippets for working with Django CBVs.
Defining a View
This example shows how to define a PostListView
class that handles requests for displaying a list of blog posts.
class PostListView(View):
def get(self, request):
# Get the list of posts
posts = Post.objects.all()
# Render the template
return render(request, 'posts/list.html', {'posts': posts})
Handling Form Submissions
This example shows how to add a post()
method to the PostListView
class to handle form submissions.
class PostListView(View):
# ...
def post(self, request):
# Get the form data
title = request.POST['title']
content = request.POST['content']
# Create a new post
post = Post(title=title, content=content)
post.save()
# Redirect to the post list page
return redirect('posts:list')
Adding Custom Logic
This example shows how to add a search()
method to the PostListView
class to handle search requests.
class PostListView(View):
# ...
def search(self, request):
# Get the search term
search_term = request.GET['q']
# Get the list of posts
posts = Post.objects.filter(title__contains=search_term)
# Render the template
return render(request, 'posts/list.html', {'posts': posts})
Tips and Tricks
Here are some tips and tricks for working with Django CBVs:
- Use URL namespacing to keep your views organized.
- Use mixins to share common functionality between views.
- Use the
dispatch()
method to add custom logic before or after a request is handled. - Use the
get_context_data()
method to add extra data to the context when rendering a template.
Conclusion
Django Class-Based-Views are a powerful way to organize and structure your web application. With a few simple techniques, you can quickly and easily build efficient, maintainable, and scalable web applications. We hope this guide has helped you understand the basics of working with Django CBVs and given you some useful tips and tricks.
Recent Posts
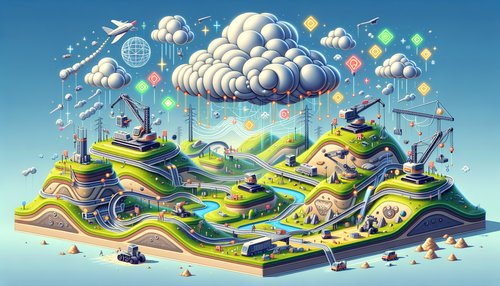
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
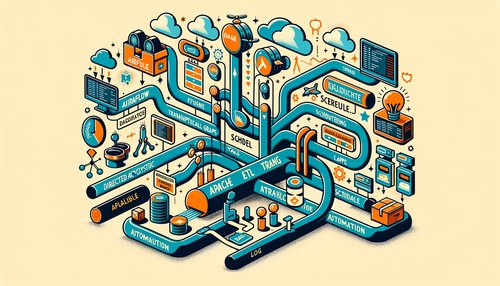
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow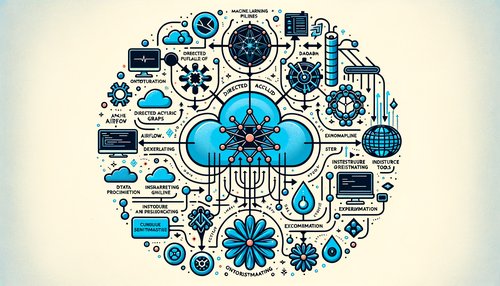
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow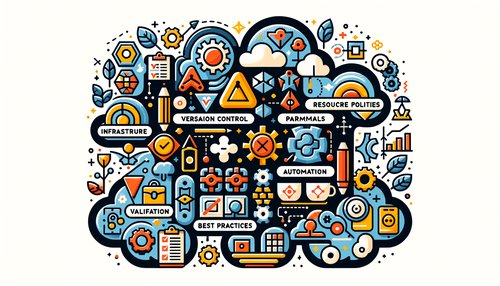
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
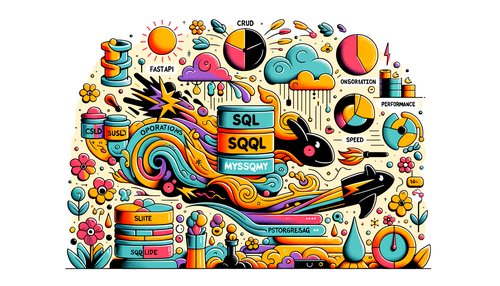