Navigating Your Angular App with Ease: Unlock the Power of Routing
Routing is a key part of any web application, and Angular provides a powerful and intuitive way to handle routing. By taking advantage of the Angular Router, you can easily navigate and manage your application with ease. In this post, we’ll look at the basics of Angular routing and how it can help you create a powerful and user-friendly web application.
What is Angular Routing?
Angular routing allows you to create routes within your application. Routes are URLs that are associated with specific components, and they can be used to navigate your application. When a user navigates to a route, the associated component is displayed in the browser. This makes it easy to navigate to different parts of your application without having to manually update the URL.
Setting Up Routes
Setting up routes in Angular is simple. First, you’ll need to create a file that will hold your routes. This file is typically named app-routing.module.ts
. Inside this file, you’ll need to create an array of routes. Each route should include a path
and a component
property. The path
is the URL that the user will navigate to, and the component
is the component that will be displayed.
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent }
];
Once you’ve created your routes, you’ll need to add them to the RouterModule
. You can do this by calling the forRoot
method and passing in your routes array.
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Adding Links to Routes
Now that you have your routes set up, you can start adding links to them. You can do this using the routerLink
directive. This directive allows you to link to a route from any template.
<a routerLink="/home">Home</a>
<a routerLink="/about">About</a>
<a routerLink="/contact">Contact</a>
Conclusion
Routing is a powerful tool that can help you create a powerful and user-friendly web application. By taking advantage of the Angular Router, you can easily create routes, add links to them, and manage your application with ease. With a few simple steps, you can unlock the power of routing and make navigating your application a breeze.
Recent Posts
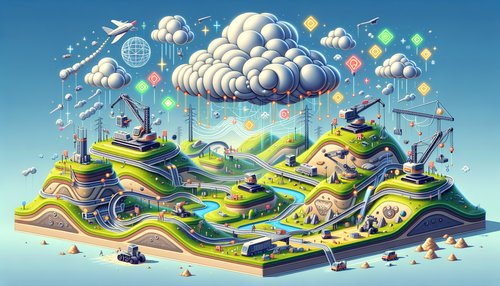
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
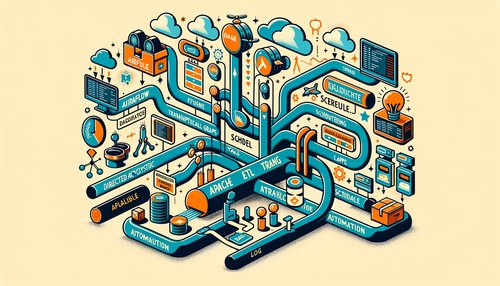
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow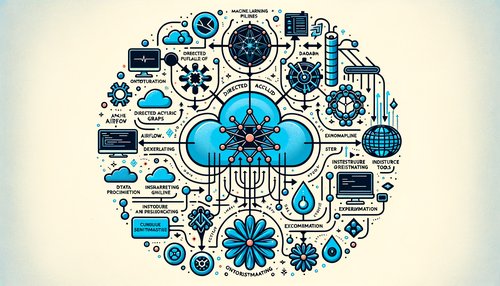
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow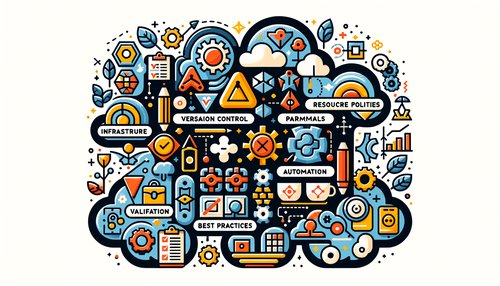
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
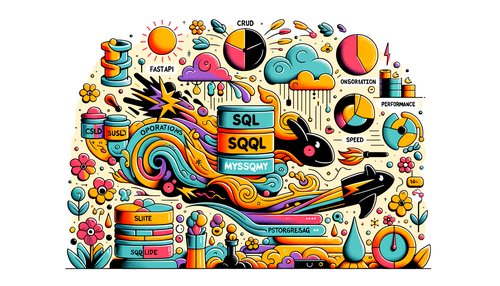