Unlock the Power of Function-Based Views in Your Django App!
Django is an amazing web framework that allows you to create powerful web applications quickly and easily. One of the powerful features of Django is its function-based views, which allow you to quickly create view functions that can be used to handle requests and return responses. Function-based views are incredibly powerful and can be used to create a wide variety of web applications. In this blog post, we’ll take a look at how to use function-based views to create a basic Django app.
Creating the App
The first step to creating a Django app is to create a folder for our project. We’ll call it my_project
. Inside this folder, we’ll create a views.py
file. This file will contain all of our view functions. For our example, we’ll create a basic index page that will display a message.
# views.py def index(request): return HttpResponse("Hello, World!")
Now that we have our view function, we need to create a urls.py
file. This file will contain the URLs for our app. For our example, we’ll create a single URL that will point to our index page.
# urls.py from django.urls import path from .views import index urlpatterns = [ path('', index, name='index'), ]
Now that we have our view function and URL set up, we need to add our app to the INSTALLED_APPS
list in our settings.py
file. We also need to add our URL patterns to the ROOT_URLCONF
list in our settings.py
file.
# settings.py INSTALLED_APPS = [ ... 'my_project', ] ROOT_URLCONF = [ ... 'my_project.urls' ]
Now that our app is set up, we can start the development server and visit our app in the browser. We should see our message being displayed.
Conclusion
Function-based views are incredibly powerful and can be used to create a wide variety of web applications. In this blog post, we looked at how to use function-based views to create a basic Django app. With a few simple steps, you can quickly create powerful web applications with Django.
Recent Posts
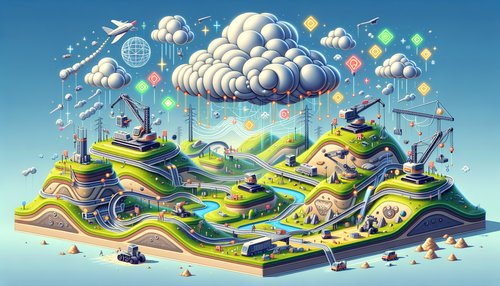
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
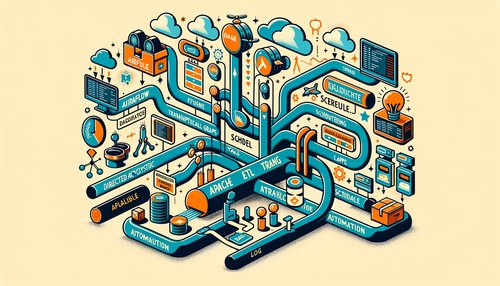
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow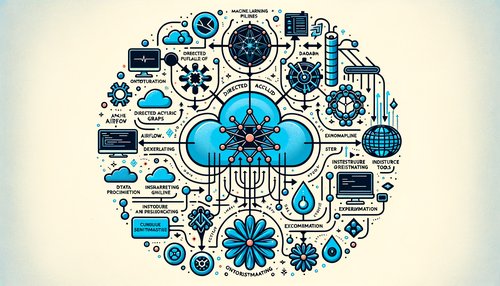
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow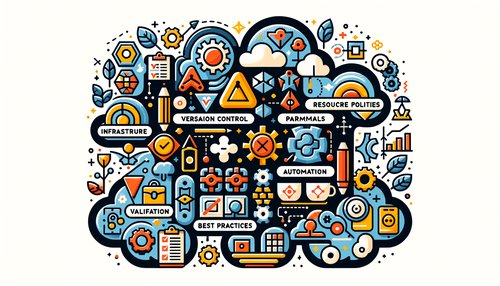
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
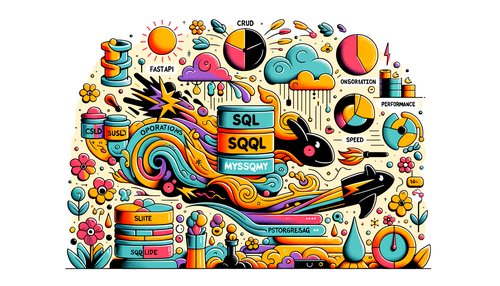