Unlocking FastAPI's Full Potential: Your Ultimate Guide to Handling Request Forms and Files Like a Pro
Welcome to the definitive guide on mastering the handling of request forms and files in FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. If you're looking to elevate your FastAPI skills and learn how to efficiently process form data and files, you've landed in the right place. This guide is packed with practical tips, examples, and insights that will turn you into a FastAPI pro in no time. So, let's dive in and explore the powerful features FastAPI offers for handling forms and files!
Understanding Request Forms in FastAPI
Handling form data is crucial for many web applications, whether it's processing login data, user settings, or any input that relies on a form. FastAPI simplifies this process with its straightforward approach, enabling you to handle form data seamlessly.
Practical Tip: Use FastAPI's Form
class to define form fields within your endpoint functions. This not only makes your code cleaner but also adds automatic request validation based on type annotations.
Example:
from fastapi import FastAPI, Form app = FastAPI() @app.post("/login/") async def login(username: str = Form(...), password: str = Form(...)): return {"username": username}
This snippet showcases a simple login form handling, where the username and password are required fields, thanks to the ellipsis (...).
Mastering File Uploads with FastAPI
Uploading files is another common requirement for web applications. FastAPI provides robust support for file handling that integrates smoothly with its asynchronous nature.
Practical Tip: Utilize FastAPI's File
and UploadFile
classes for file uploads. UploadFile
offers more flexibility than bytes
, as it does not load the whole file into memory, making it ideal for large files.
Example:
from fastapi import FastAPI, File, UploadFile app = FastAPI() @app.post("/uploadfile/") async def create_upload_file(file: UploadFile = File(...)): return {"filename": file.filename}
This example illustrates how to create a file upload endpoint. The file's name is returned as a response, showcasing the ease of accessing file metadata.
Advanced Techniques: Combining Forms and Files
FastAPI's flexibility shines when you need to handle more complex scenarios, such as receiving both form fields and files in a single request.
Practical Insight: When combining forms and files, ensure your HTML form is set to multipart/form-data
. This allows both form data and files to be sent in the same request, which FastAPI can parse efficiently.
Example:
from fastapi import FastAPI, File, UploadFile, Form app = FastAPI() @app.post("/submit/") async def submit_form(file: UploadFile = File(...), notes: str = Form(...)): return {"filename": file.filename, "notes": notes}
This code snippet demonstrates receiving a file and additional notes in one go. It's a common pattern for upload forms that include descriptions or comments.
Best Practices for Handling Large Files
When dealing with large files, it's crucial to manage resource usage effectively to keep your application responsive.
Best Practice: Stream large files instead of loading them into memory. FastAPI's UploadFile
has a file
attribute that acts as a file-like object, making it possible to read chunks of the file asynchronously.
Remember: Always close the file after processing to free up resources.
Summary
In this guide, we've unlocked the secrets to handling request forms and files in FastAPI, covering everything from basic form handling and file uploads to advanced techniques for combining forms and files. We've also touched on best practices for managing large files efficiently.
FastAPI offers a powerful yet elegant toolkit for working with forms and files, making it an excellent choice for developing modern web applications. By applying the tips and practices outlined in this guide, you're well on your way to mastering FastAPI's capabilities in handling user input and file data like a pro.
As you continue to build and refine your FastAPI applications, keep exploring and experimenting with these features to discover even more ways to enhance your app's functionality and user experience.
Final Thought: Dive in, start coding, and unlock the full potential of your FastAPI applications by mastering the art of handling request forms and files!
Recent Posts
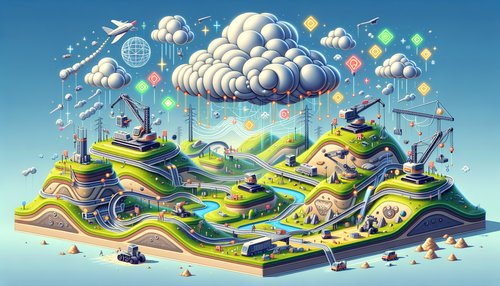
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
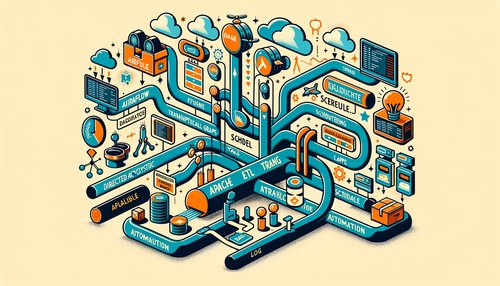
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow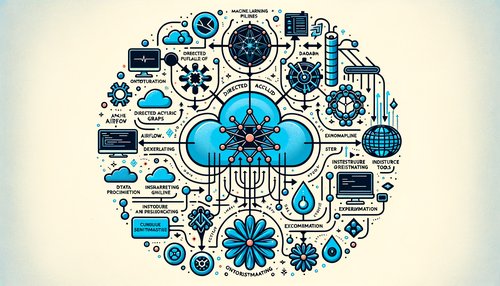
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow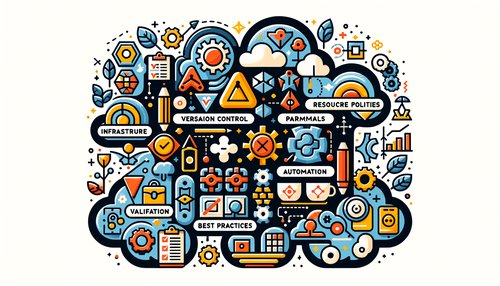
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
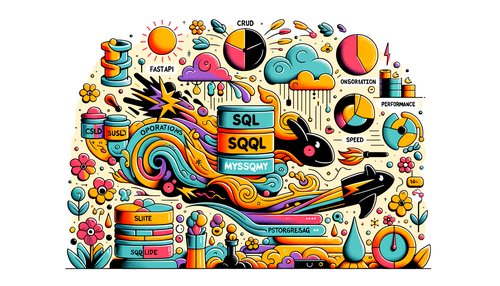