Unlock the Power of Angular Components: A Guide to Easily Building Dynamic Web Applications
Angular is a popular JavaScript framework that makes it easy to create powerful web applications. It's especially useful for building dynamic, interactive user interfaces, and it can help you quickly build complex, data-driven apps. One of the most powerful features of Angular is its components. Components are reusable pieces of code that can be used to create complex features and functions in your web app.
In this guide, we'll look at how to use Angular components to build dynamic web applications. We'll cover how to create components, how to use them in your code, and how to make them more powerful. We'll also take a look at some helpful resources to get you started. Let's get started!
Creating Components
Creating an Angular component is a simple process. You just need to define a template and a controller. The template defines the HTML that will be rendered, and the controller defines the logic that will be used to manipulate the data. Here's an example of a simple component:
<div class="my-component">
<h1>{{title}}</h1>
<p>{{message}}</p>
</div>
app.component('myComponent', {
templateUrl: 'my-component.html',
controller: function() {
this.title = 'Hello World';
this.message = 'This is a message from my component';
}
});
This component will render a simple message on the page. You can use this same pattern to create more complex components.
Using Components
Once you've created a component, you can use it in your code. You can use the ng-include
directive to insert the component into your HTML. You can also use the $compile
service to compile and link the component to your code. Here's an example of using the ng-include
directive:
<div ng-include="'my-component.html'"></div>
Making Components More Powerful
You can make your components more powerful by using directives and services. Directives allow you to manipulate the DOM and create custom elements, while services allow you to share data between components. Here's an example of using a directive to add a custom element to your component:
<div class="my-component">
<h1>{{title}}</h1>
<p>{{message}}</p>
<my-custom-element></my-custom-element>
</div>
app.directive('myCustomElement', function() {
return {
template: '<div>This is a custom element</div>'
};
});
This will add a custom element to your component, which can be used to add additional features and functionality.
Wrapping Up
Angular components are a powerful way to quickly build dynamic web applications. They allow you to create reusable pieces of code that can be used to create complex features and functions in your app. By using directives and services, you can make your components even more powerful.
If you're looking to get started with Angular components, check out the official documentation or some of the helpful resources listed below. Good luck!
Resources
Recent Posts
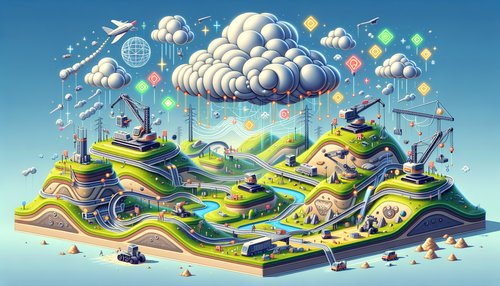
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
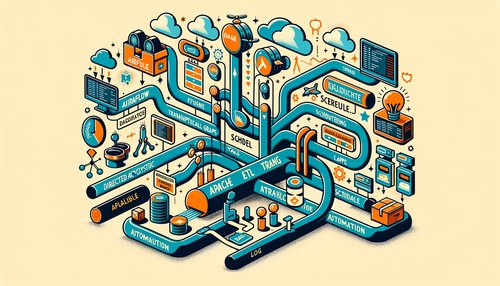
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow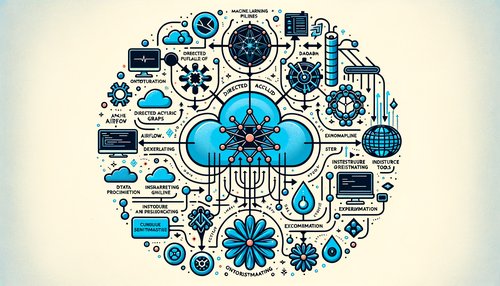
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow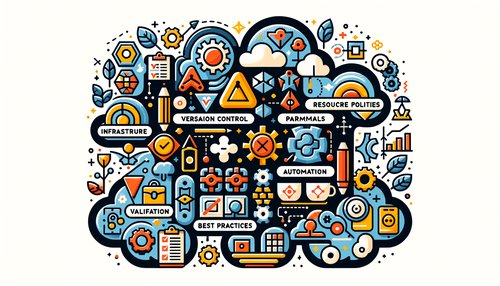
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
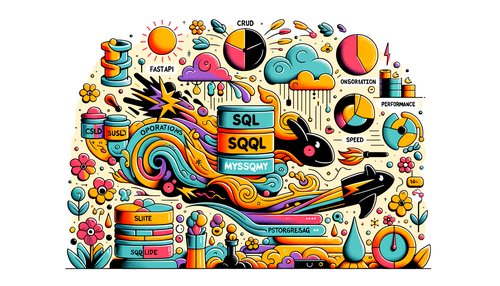