Unlock the Power of React Components - An Introduction to Component-Based Design
React is a powerful front-end library for building user interfaces. One of its main advantages is the way it helps developers create reusable components. In this article, we will discuss the concept of component-based design and how it can help you unlock the power of React components.
Component-based design is a way of organizing code into smaller, reusable pieces. Each component can be thought of as a self-contained module that can be used in multiple places. Components are also easily composable, meaning they can be combined to create larger, more complex pieces of functionality.
When used in the context of React, components allow developers to create large applications with minimal code. Instead of writing all the code from scratch, components can be reused and combined to quickly create a working application.
Benefits of Component-Based Design
Component-based design has several benefits for developers. Here are just a few:
- Reusability: Components can be reused in multiple places, saving time and effort.
- Scalability: Components can be easily combined to create complex pieces of functionality.
- Maintainability: Components can be updated and maintained without affecting the rest of the application.
Working with React Components
React components are written in JavaScript. They consist of a render()
method, which is responsible for rendering the component's HTML.
Here is a simple example of a React component:
import React from 'react';
class MyComponent extends React.Component {
render() {
return (
<div>
<h1>My Component</h1>
<p>This is my component.</p>
</div>
);
}
}
This component will render an <h1>
tag with the text "My Component" and a <p>
tag with the text "This is my component."
Unlocking the Power of React Components
By leveraging the power of components, developers can create powerful, maintainable applications with minimal effort. Components can be reused, combined, and updated without affecting the rest of the application. This makes it easier to create large, complex applications without having to start from scratch.
Component-based design is a great way to unlock the power of React components and quickly create powerful applications. With a little practice, you can quickly become a master of component-based design!
Recent Posts
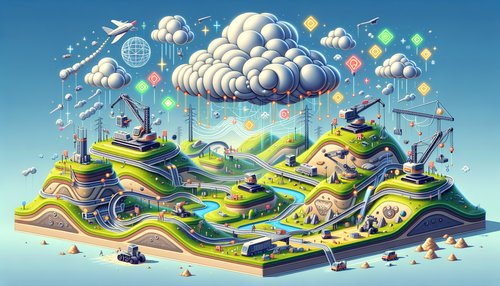
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
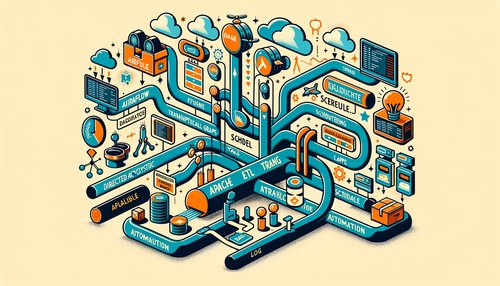
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow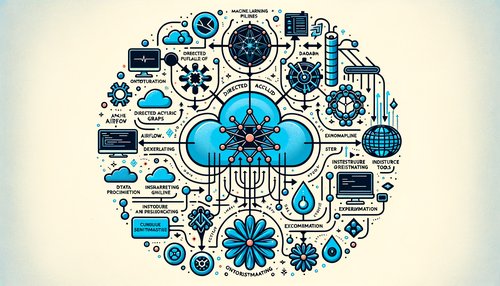
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow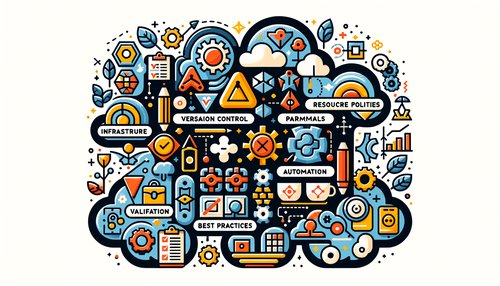
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
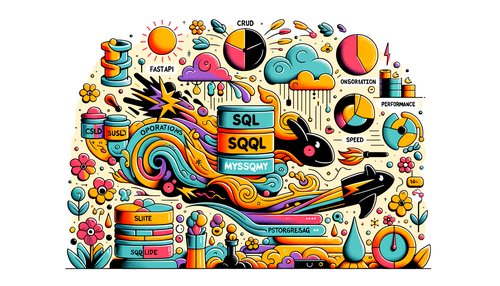