Unlock the Power of React Components: Create Amazing User Experiences with Ease
React components are one of the most powerful tools for creating amazing user experiences. They allow developers to quickly and easily create interactive, dynamic, and responsive components for web applications. React components are flexible, easy to use, and highly customizable, making them a popular choice for developers.
In this post, we’ll explore the basics of React components and how they can be used to create amazing user experiences. We’ll also look at some code examples that demonstrate how to use React components to create powerful and interactive web applications.
What are React Components?
React components are pieces of code that allow developers to create custom user interfaces for web applications. They are reusable, self-contained components that can be used to create a wide range of user experiences. React components are written using JavaScript and are usually composed of multiple components.
React components are designed to be highly customizable and can be used to create complex web applications. They are also designed to be easy to maintain and update, making them an ideal choice for developers.
How to Use React Components
Using React components is fairly simple. To create a React component, you’ll need to create a JavaScript file that contains the component’s code. The code should include the component’s structure, styles, and behavior.
Once the component is created, it can be imported into a web application and used to create a user interface. React components can be used in combination with other components to create a powerful and dynamic user experience.
Code Examples
Below are some code examples that demonstrate how to use React components to create amazing user experiences.
Creating a Simple React Component
This example shows how to create a simple React component.
import React from 'react';
const MyComponent = () => {
return (
<div>
<h1>My Component</h1>
<p>This is my React component.</p>
</div>
);
};
export default MyComponent;
Creating a Component with Props
This example shows how to create a React component that takes props.
import React from 'react';
const MyComponent = (props) => {
return (
<div>
<h1>{props.title}</h1>
<p>{props.description}</p>
</div>
);
};
export default MyComponent;
Conclusion
React components are a powerful tool for creating amazing user experiences. They are easy to use, highly customizable, and can be used to create complex web applications. By following the examples in this post, you can unlock the power of React components and create amazing user experiences with ease.
Recent Posts
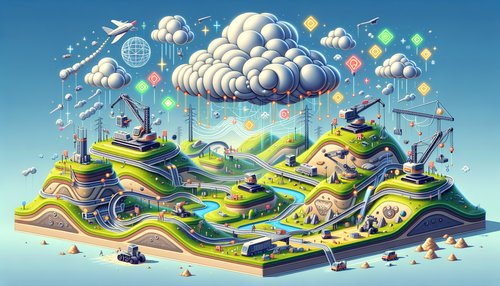
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
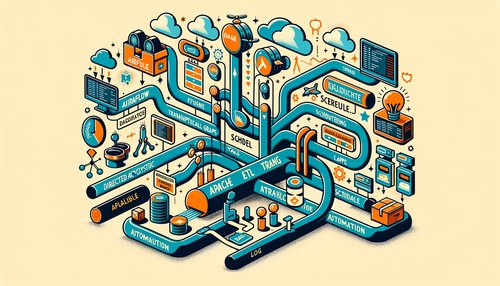
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow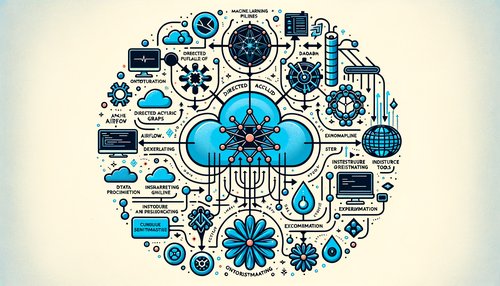
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow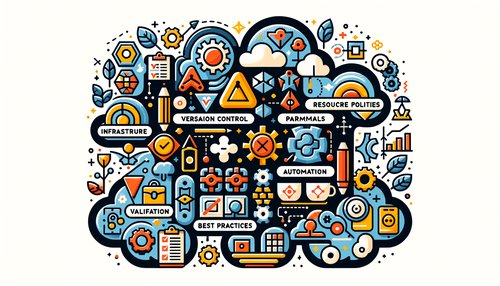
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
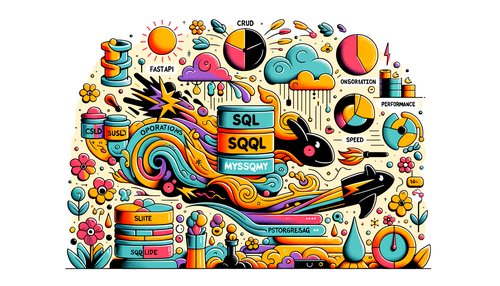