Unlock the Power of Serverless Computing With AWS Lambda!
Serverless computing is the new wave of cloud computing that allows developers to focus on the code they write, instead of managing and configuring servers and infrastructure. With AWS Lambda, a serverless computing platform, developers can quickly and easily create and deploy applications that are able to scale automatically and handle varying traffic loads.
AWS Lambda simplifies the process of creating and managing serverless applications. It enables developers to write code in Node.js, Python, Java, or C# and then deploy it to AWS Lambda. The code is then run on a serverless compute service, which is managed by AWS, so that developers don’t have to worry about managing servers or infrastructure.
AWS Lambda provides several benefits, such as the ability to quickly deploy code without having to manage servers or infrastructure. It also allows developers to scale quickly, as Lambda automatically scales up or down depending on the amount of traffic the application is receiving. Additionally, Lambda is cost-effective, as developers only pay for the time their code is running.
To get started with AWS Lambda, developers must first create and configure a Lambda function. This can be done using the AWS Lambda console, or via the AWS Command Line Interface (CLI). Once the function is created, developers can write code in one of the supported languages and then deploy it to Lambda.
Developers can also use AWS Lambda to create event-driven applications. These applications can be triggered by various events, such as a new file being uploaded to an S3 bucket or a user interacting with an API. When an event occurs, Lambda will automatically invoke the code associated with that event and execute it.
In addition to creating serverless applications, AWS Lambda can also be used to automate tasks. For example, developers can use Lambda to automatically back up files to an S3 bucket, or to invoke a Lambda function when an event occurs. This makes it easy to automate complex tasks without having to manage servers or infrastructure.
AWS Lambda is a powerful tool for developers looking to leverage the power of serverless computing. With its ability to quickly deploy code, scale automatically, and handle varying traffic loads, Lambda makes it easy to create and manage serverless applications. Additionally, its ability to automate tasks makes it an invaluable tool for developers looking to streamline their workflow.
const AWS = require('aws-sdk');
// Create an AWS Lambda instance
const lambda = new AWS.Lambda({
region: 'us-east-1'
});
// Create a Lambda function
const params = {
FunctionName: 'MyFunction',
Runtime: 'nodejs10.x',
Role: 'arn:aws:iam::123456789012:role/lambda-role',
Handler: 'index.handler',
Code: {
ZipFile: '...'
}
};
lambda.createFunction(params, (err, data) => {
if (err) {
console.log(err);
} else {
console.log(data);
}
});
Recent Posts
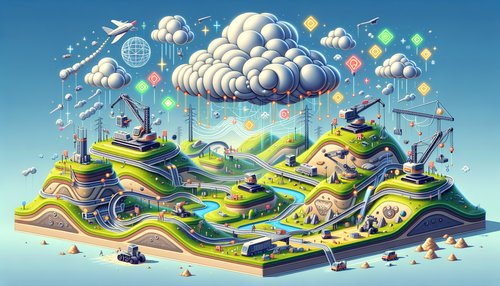
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
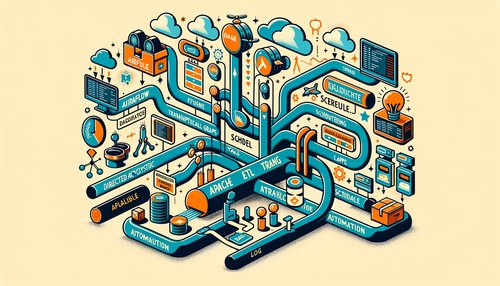
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow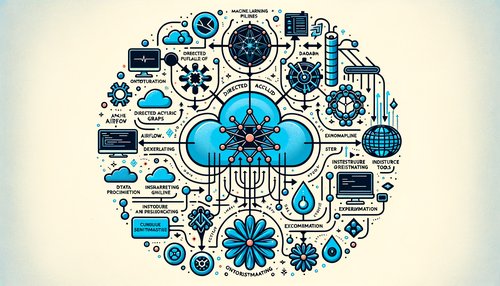
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow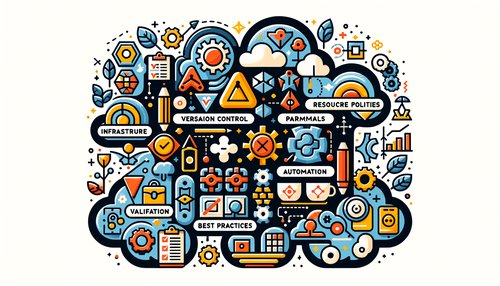
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
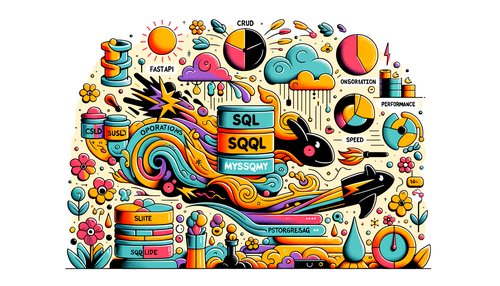