Unlock the Power of Angular Routing: A Comprehensive Guide
Routing is one of the most powerful tools in the Angular framework. It enables developers to create efficient, user-friendly web applications that are easily navigable and accessible. In this comprehensive guide, we'll cover all the basics of Angular routing, from setting up your routes to applying custom styling.
Setting Up the Routes
The first step to setting up your routes is to create a routes.ts
file. This file will contain all the routes that you'll use in your application.
// routes.ts
import { Routes } from '@angular/router';
export const routes: Routes = [
{
path: 'home',
component: HomeComponent
},
{
path: 'about',
component: AboutComponent
},
{
path: 'contact',
component: ContactComponent
}
];
Once the routes are set up, you can import them into your app.module.ts
file and add them to the RouterModule.forRoot
array.
// app.module.ts
import { RouterModule } from '@angular/router';
import { routes } from './routes';
@NgModule({
imports: [
RouterModule.forRoot(routes)
],
...
})
export class AppModule { }
Customizing the Routes
You can also customize the routes with custom styling and parameters. For example, you can add a data
property to each route to store additional information.
// routes.ts
export const routes: Routes = [
{
path: 'home',
component: HomeComponent,
data: {
title: 'My Home Page'
}
},
...
];
You can also add parameters to the route path to capture dynamic data. For example, you could add a :id
parameter to the contact
route to capture the contact's ID.
// routes.ts
export const routes: Routes = [
...
{
path: 'contact/:id',
component: ContactComponent
}
];
Navigating the Routes
Once the routes are set up, you can use the Router
service to navigate between them. This service provides several methods for navigating, including navigateByUrl()
, navigate()
and navigateByUrlTree()
.
// app.component.ts
constructor(private router: Router) {}
goToHome() {
this.router.navigate(['/home']);
}
Conclusion
Routing is an essential part of any Angular application. By following this comprehensive guide, you'll be able to set up your routes and customize them with custom styling and parameters. You'll also learn how to navigate between routes using the Router
service. With these tools, you'll be able to create efficient, user-friendly web applications.
Recent Posts
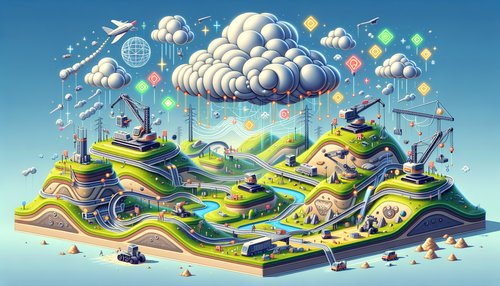
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
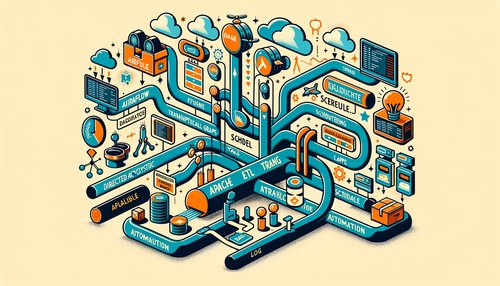
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow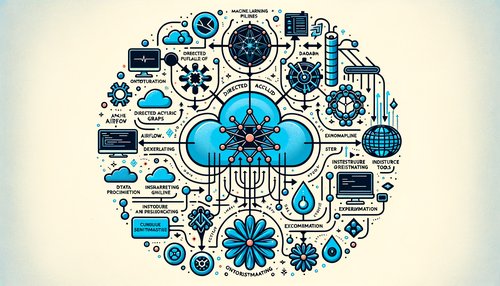
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow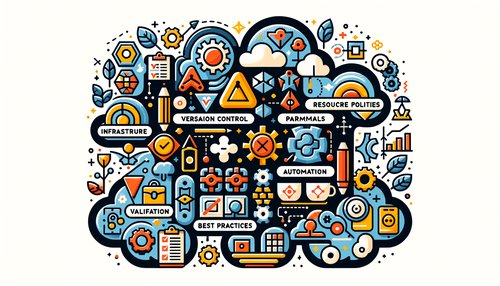
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
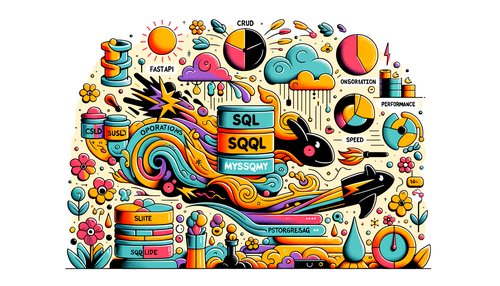