Unlock the Power of Django Receivers - A Comprehensive Guide to Advanced Signaling
Django is a powerful web framework that's used by many developers to build robust applications. One of the most powerful features of Django is its signal system, which allows developers to create custom signals and receive notifications when certain events occur.
Signals are useful for a variety of tasks, such as sending email notifications, updating caches, and triggering other actions. But when working with signals, it's important to understand the different types of signals and how to use them effectively. This guide will explain the basics of Django signal receivers and provide examples of how to use them.
What is a Signal Receiver?
A signal receiver is a Python function that receives a signal when it is sent. Signal receivers are registered with Django's signal system and are triggered when a signal is sent. Signal receivers can be used to perform a variety of tasks, such as sending emails, updating caches, and triggering other actions.
Signal receivers can be registered to a specific signal, or to all signals. When a signal is sent, the signal receiver is called with the signal as an argument. Signal receivers can also be registered to a specific sender, so that only signals from that sender will trigger the signal receiver.
How to Use Signal Receivers
To use signal receivers, you must first create a signal. Signals are created using the @receiver
decorator. This decorator takes two arguments: the signal to receive, and the function to call when a signal is received.
Here's an example of a signal receiver that sends an email when a signal is received:
@receiver(signal)
def send_email(sender, **kwargs):
# Send email code here
The send_email
function will be called when the signal is sent. The sender
argument will contain the sender of the signal, and kwargs
will contain any additional arguments that were sent with the signal.
You can also register signal receivers to receive all signals. This is useful for logging or debugging, as you can see all signals that are sent. To register a signal receiver for all signals, you can use the @receiver_for_all_signals
decorator.
@receiver_for_all_signals
def log_signal(sender, **kwargs):
# Log signal code here
The log_signal
function will be called for every signal that is sent.
Conclusion
Signal receivers are a powerful feature of Django that can be used to perform a variety of tasks. By understanding the different types of signal receivers and how to use them effectively, you can unlock the power of Django's signal system.
Recent Posts
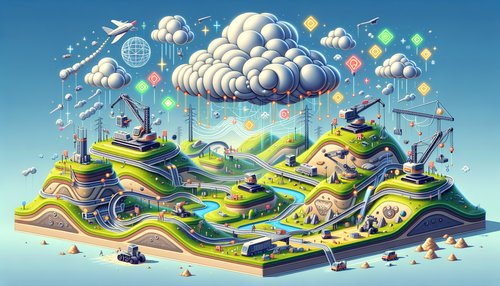
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
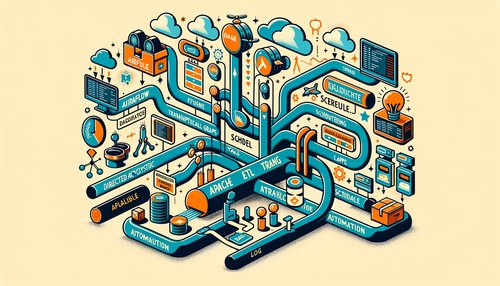
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow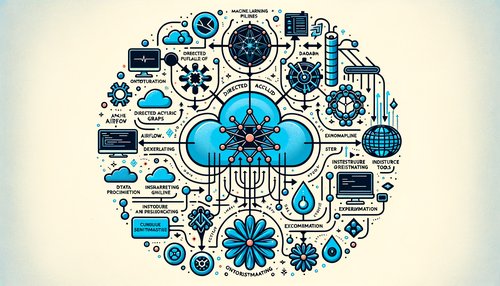
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow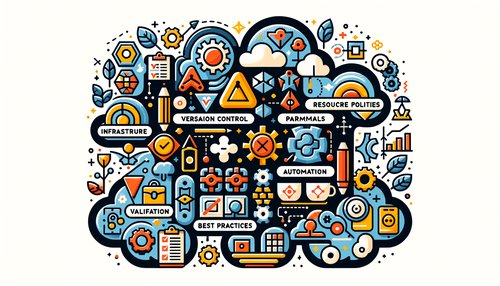
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
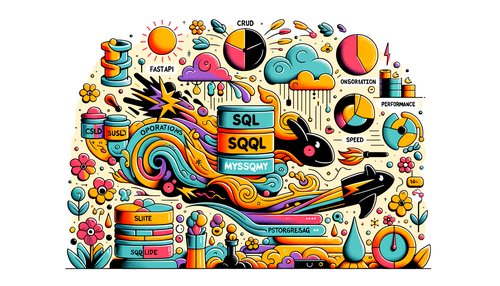