Unlock the Power of React Hooks: Transform Your React Code for Maximum Efficiency
React Hooks are the latest addition to the React library, and they’re quickly becoming the go-to method for writing efficient, powerful React code. Hooks allow you to use state and other React features without writing a class, making them incredibly versatile. They also make your code more organized and easier to read, leading to better performance and fewer bugs.
If you’re ready to take your React code to the next level, it’s time to unlock the power of React Hooks. Here’s how you can transform your React code for maximum efficiency.
Step 1: Understand the Basics of Hooks
Before you can start using React Hooks, it’s important to understand the basics. React Hooks are JavaScript functions that allow you to “hook into” React features such as state and lifecycle methods. They allow you to use React features without writing a class, and they can be used in both function components and class components.
Step 2: Create a Custom Hook
Once you have a basic understanding of React Hooks, you can create your own custom Hooks. Custom Hooks allow you to reuse logic across multiple components, making your code more efficient and organized. To create a custom Hook, simply write a function that follows the naming convention of useMyCustomHook
.
function useMyCustomHook() {
const [myData, setMyData] = useState([]);
useEffect(() => {
fetchData().then(data => setMyData(data));
}, []);
return myData;
}
Step 3: Use Hooks in Your Components
Now that you’ve created a custom Hook, you can start using it in your components. To use a Hook in a component, simply call it at the top of the component and pass any necessary arguments.
function MyComponent() {
const myData = useMyCustomHook();
return (
<div>
{myData.map(item => (
<div>{item.name}</div>
))}
</div>
);
}
Step 4: Refactor Your Components
Once you’ve started using Hooks in your components, you can begin refactoring your code. Hooks make it easy to extract logic from components and move it into custom Hooks, making your code more organized and easier to read. This can lead to better performance and fewer bugs.
Step 5: Take Advantage of Other Hooks
React has a number of built-in Hooks that you can use to make your code even more efficient. For example, the useEffect
Hook allows you to perform side effects such as data fetching and setting up subscriptions. The useContext
Hook allows you to access context from a component, and the useReducer
Hook allows you to manage state in complex components.
Unlock the Power of React Hooks Today
React Hooks are a powerful tool for transforming your React code and making it more efficient. By understanding the basics of Hooks, creating custom Hooks, and taking advantage of other Hooks, you can unlock the power of React Hooks and transform your React code for maximum efficiency.
Recent Posts
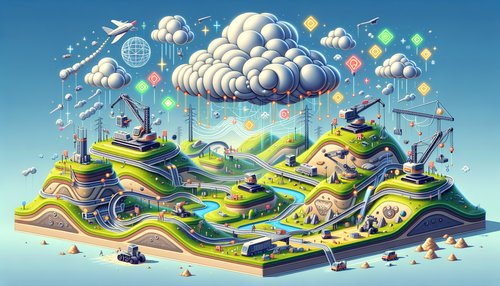
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
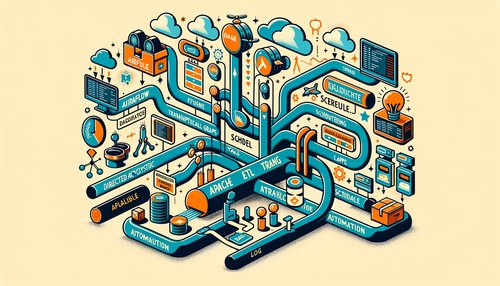
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow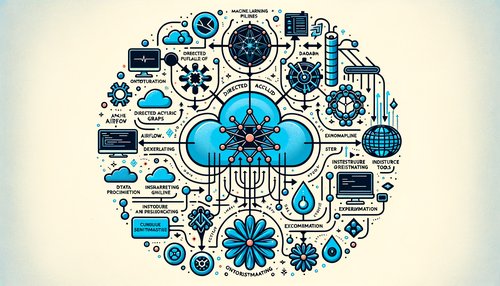
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow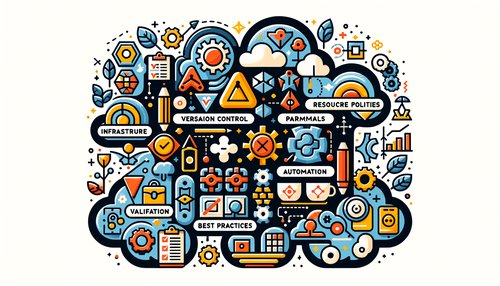
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
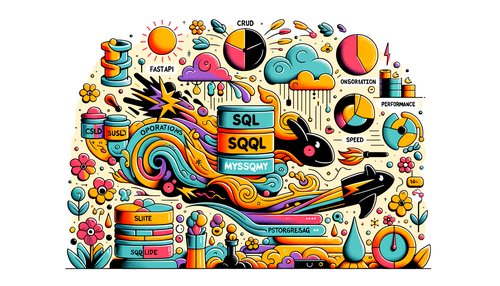