Unlock the Power of React Hooks and Take Your React Development to the Next Level
React hooks have revolutionized the way developers build React applications. React hooks allow developers to use state and other React features without writing a class. This makes React development easier and faster. With hooks, developers can use functional components instead of class components, making their code easier to read and maintain.
In this blog post, we will discuss what React hooks are and how they can help you take your React development to the next level. We will also provide some code snippets to help you get started.
What are React Hooks?
React hooks are functions that allow developers to use state and other React features without writing a class. React hooks let you use state and other React features in functional components, instead of writing a separate class component. This makes React development easier and faster.
How Can React Hooks Help You Take Your React Development to the Next Level?
React hooks can help you take your React development to the next level in several ways. First, they make your code easier to read and maintain. Because you don't have to write a separate class component, your code is easier to read and understand. Second, React hooks allow you to use state and other React features in functional components. This makes it easier to share logic between components, which can help you improve the performance of your application. Finally, React hooks make it easier to test your application, since you don't have to create a separate class for every component.
Code Snippets
Here are some code snippets to help you get started with React hooks.
Using the useState Hook
The useState hook allows you to add state to your functional components. Here's an example of how to use it:
import React, { useState } from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
Using the useEffect Hook
The useEffect hook allows you to perform side effects in your functional components. Here's an example of how to use it:
import React, { useEffect } from 'react';
function Example() {
useEffect(() => {
// Update the document title using the browser API
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
Conclusion
React hooks are an incredibly powerful tool for React developers. They make React development easier and faster, and allow developers to use state and other React features in functional components. With React hooks, you can take your React development to the next level.
Recent Posts
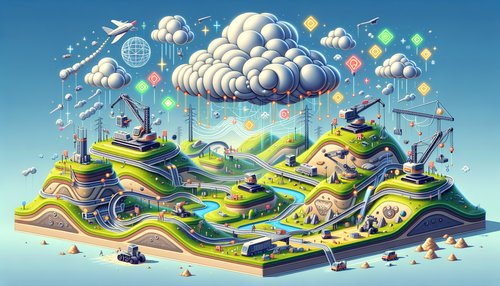
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
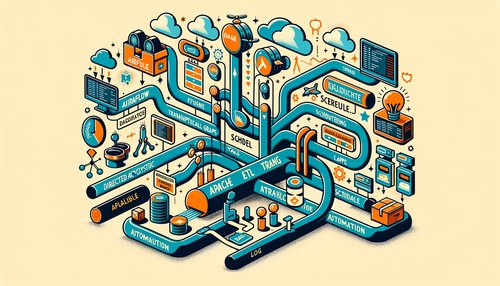
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow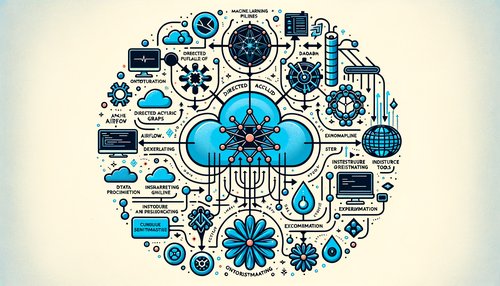
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow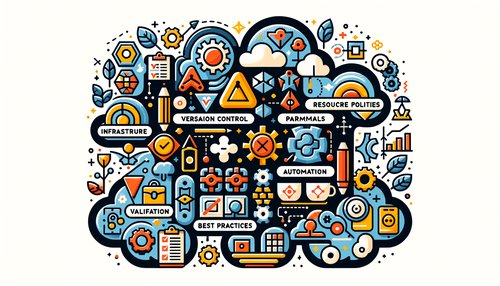
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
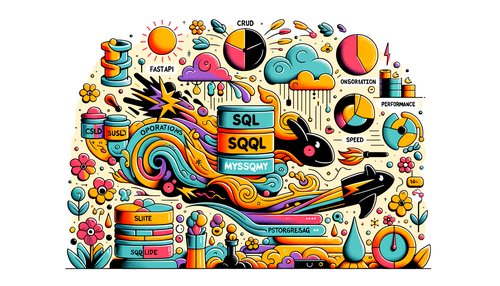