Unlock the Power of Django Function-Based-Views and Take Your Web Development to the Next Level!
Django is an open-source web framework that makes it easy to create complex, data-driven web applications. One of the most powerful features of Django is its robust function-based views (FBV). FBVs are a great way to quickly and easily create webpages from data stored in a database. With FBVs, you can quickly and easily create dynamic webpages that can be used to create interactive user experiences.
FBVs are a great way for developers to quickly create dynamic webpages. They allow developers to quickly create webpages from data stored in a database. With FBVs, you can create webpages that can be used to create interactive user experiences.
FBVs are relatively simple to use and can be quickly integrated into any web application. To create an FBV, you first need to create a function that takes a request object as an argument. This function will contain the code that will be executed when the page is requested.
For example, let's say you want to create a page that displays a list of books. To do this, you would create a function that takes a request object as an argument and then use the request object to retrieve the list of books from the database.
def book_list(request):
books = Book.objects.all()
return render(request, 'book_list.html', {'books': books})
The render
function is used to render the template for the page. The book_list.html
template would contain the HTML code for the page, and the books
variable would be passed to the template so that the list of books can be displayed.
Once the function is created, you can then map it to a URL using the urlpatterns
in your urls.py
file. For example:
urlpatterns = [
path('books/', book_list, name='book_list'),
]
This will map the book_list
view to the /books/
URL. Now, when a user visits the /books/
URL, the book_list
view will be executed and the list of books will be displayed.
FBVs are a great way to quickly and easily create dynamic webpages. With FBVs, you can quickly create webpages from data stored in a database and create interactive user experiences. So if you want to take your web development to the next level, then FBVs are a great way to do it.
Recent Posts
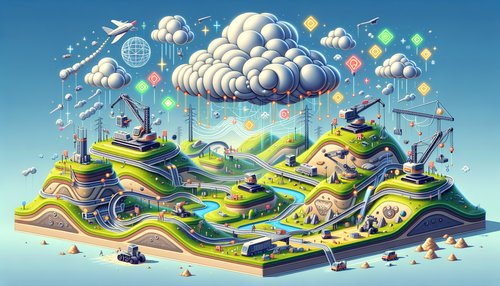
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
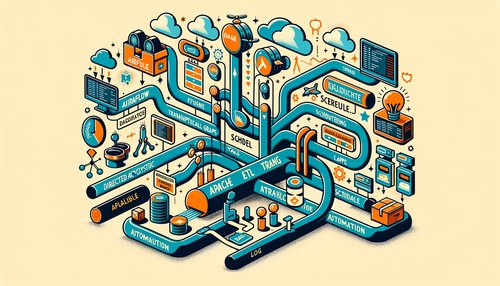
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow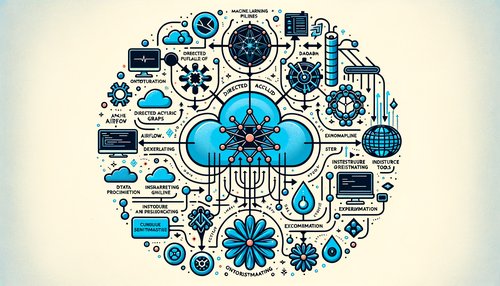
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow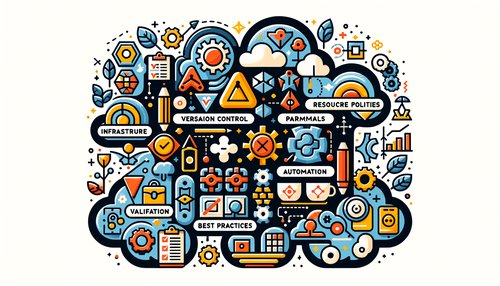
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
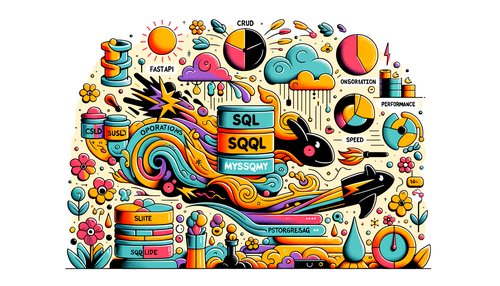