Unleashing Creativity with React Hooks: Transforming Your Web Development Workflow
React Hooks have been a game-changer for functional components in React. Since their introduction, they have transformed the way developers write React components, unleashing new levels of creativity and simplifying state management. In this blog post, we will explore how React Hooks can transform your web development workflow, making it more efficient and enjoyable.
Understanding React Hooks
Hooks are functions that let you "hook into" React state and lifecycle features from function components. They provide a more direct API to the React concepts you already know: props, state, context, refs, and lifecycle. Prior to Hooks, these features were only available in class components.
useState: Managing State in Functional Components
The useState
hook is the first tool in our box. It lets us add state to functional components. Here's a simple counter component that uses useState
to manage its state:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
useEffect: Side Effects in Functional Components
The useEffect
hook lets you perform side effects in function components. It serves the same purpose as componentDidMount
, componentDidUpdate
, and componentWillUnmount
in React class lifecycle. Here's how you might use useEffect
to fetch data from an API:
import React, { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState([]);
useEffect(() => {
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
}
fetchData();
}, []); // The empty array means this effect runs once after the initial render
return (
<ul>
{data.map(item => (
<li key={item.id}>{item.title}</li>
))}
</ul>
);
}
useContext: Simplifying Context
The useContext
hook allows you to access the context more easily. It makes prop-drilling a thing of the past. Here’s a quick example of how you can use useContext
to access a theme context:
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
function ThemedButton() {
const theme = useContext(ThemeContext);
return <button className={theme}>I am styled by theme context!</button>;
}
Custom Hooks: Extracting Component Logic
Custom hooks allow you to extract component logic into reusable functions. A custom hook is a JavaScript function whose name starts with ”use” and that may call other hooks. Here's an example of a custom hook that manages the subscription to a chat API:
import React, { useState, useEffect } from 'react';
function useChatStatus(userId) {
const [isOnline, setIsOnline] = useState(null);
useEffect(() => {
function handleStatusChange(status) {
setIsOnline(status.isOnline);
}
ChatAPI.subscribeToFriendStatus(userId, handleStatusChange);
return () => {
ChatAPI.unsubscribeFromFriendStatus(userId, handleStatusChange);
};
});
return isOnline;
}
function FriendStatus(props) {
const isOnline = useChatStatus(props.userId);
if (isOnline === null) {
return 'Loading...';
}
return isOnline ? 'Online' : 'Offline';
}
Conclusion
React Hooks offer a powerful new way to build your components. They enable you to use state and other React features without writing a class, leading to simpler code and fewer bugs. By embracing hooks, you can transform your development workflow and unleash your creativity. Start experimenting with hooks today and see how they can enhance your React projects!
Recent Posts
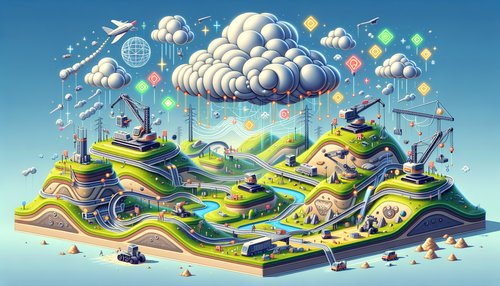
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
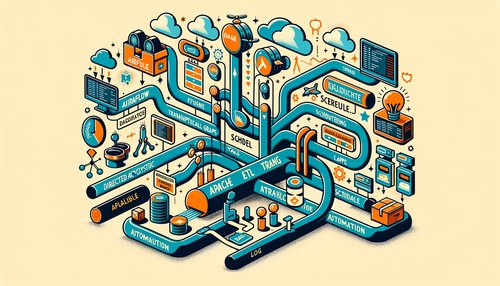
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow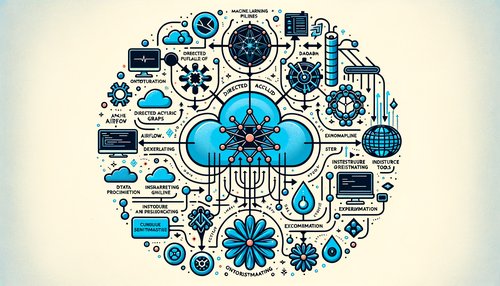
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow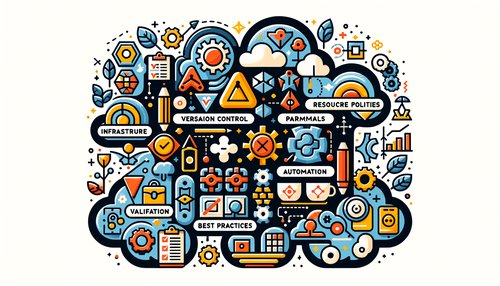
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
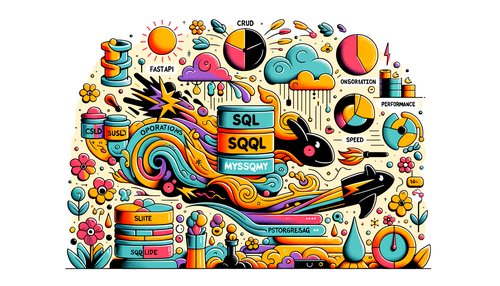