Unlocking the Secrets of FastAPI: A Comprehensive Guide to Mastering User Responses and Status Codes
Welcome to the ultimate guide on mastering user responses and status codes with FastAPI. If you've ever found yourself puzzled by the intricacies of handling HTTP responses or ensuring your API communicates effectively with clients, you're in the right place. This guide will walk you through everything from the basics of user responses to the nuances of status codes in FastAPI. Whether you're a beginner looking to get a solid start or an experienced developer aiming to refine your skills, this comprehensive exploration will provide valuable insights and practical tips to elevate your FastAPI projects.
Understanding User Responses in FastAPI
FastAPI provides a powerful yet simple way to handle user responses. This framework is designed to make your development process faster and more efficient, without sacrificing flexibility or performance. Let's dive into how FastAPI manages user responses and how you can leverage this to your advantage.
Basics of Response Model
At the heart of FastAPI's response handling is the Pydantic model, which allows for easy serialization of data and provides type hints. This ensures that your API's responses are consistent and correctly formatted. Here's a simple example:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
@app.post("/items/")
async def create_item(item: Item):
return item
This code snippet demonstrates how to define a response model and use it in a route. The Item
class dictates the structure of the response, ensuring that each item returned by the create_item
function adheres to this format.
Customizing Responses
FastAPI allows for detailed customization of responses, giving you control over headers, cookies, and status codes. This is crucial for building APIs that need to comply with specific client requirements or follow RESTful principles closely. For example, setting custom headers can be done as follows:
from fastapi import FastAPI, Response
app = FastAPI()
@app.get("/items/", status_code=200)
async def read_items():
content = {"message": "Hello, World"}
headers = {"X-Custom-Header": "Value"}
return Response(content=content, headers=headers)
This snippet illustrates how to return a custom header with your response, enhancing the flexibility of your API's communication with clients.
Mastering Status Codes with FastAPI
Status codes are a critical component of HTTP responses, providing immediate feedback about the result of a request. FastAPI simplifies the process of sending appropriate status codes with responses, ensuring that your API adheres to best practices.
Common Status Codes and Their Usage
Here are some of the most commonly used HTTP status codes in API development:
- 200 OK: Indicates that the request has succeeded.
- 201 Created: Signifies that a resource has been successfully created.
- 400 Bad Request: Used when the server cannot process the request due to client error.
- 404 Not Found: Indicates that the requested resource was not found.
- 500 Internal Server Error: Used when the server encountered an unexpected condition that prevented it from fulfilling the request.
FastAPI allows you to specify the status code for a response directly in your route decorator, ensuring that your API communicates effectively with clients.
Advanced Status Code Handling
For more complex scenarios, FastAPI offers advanced tools for handling status codes, such as the HTTPException
class. This can be used to control the flow of your application and send detailed error messages to clients. For example:
from fastapi import FastAPI, HTTPException
app = FastAPI()
@app.get("/items/{item_id}")
async def read_item(item_id: int):
if item_id != 42:
raise HTTPException(status_code=404, detail="Item not found")
return {"item_id": item_id}
This code snippet demonstrates how to use HTTPException
to return a 404 status code with a custom error message if the requested item is not found.
Conclusion
Mastering user responses and status codes is essential for developing robust and efficient APIs with FastAPI. By understanding how to define response models, customize responses, and effectively use status codes, you can ensure that your API communicates clearly and efficiently with clients. Remember, the key to successful API development is not just about handling requests but also about providing meaningful and appropriate responses. With the insights and tips provided in this guide, you're now well-equipped to take your FastAPI projects to the next level.
As you continue to explore the capabilities of FastAPI, keep experimenting with different response models and status codes to find the best fit for your API's needs. Happy coding!
Recent Posts
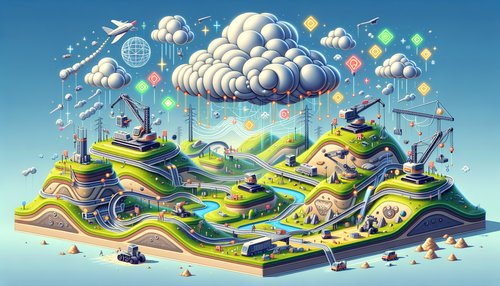
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
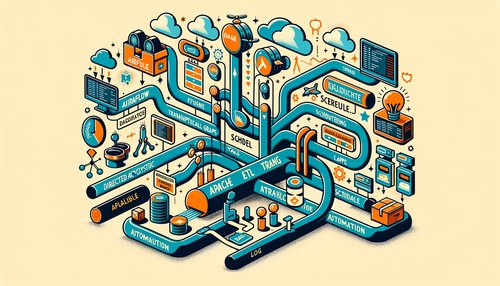
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow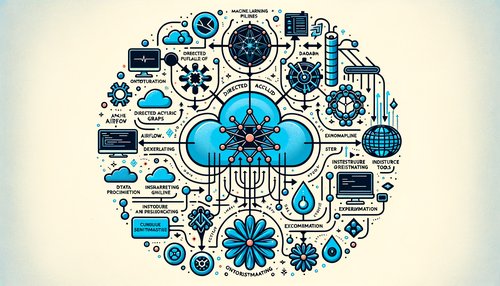
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow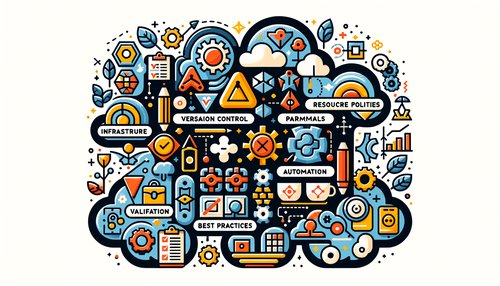
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
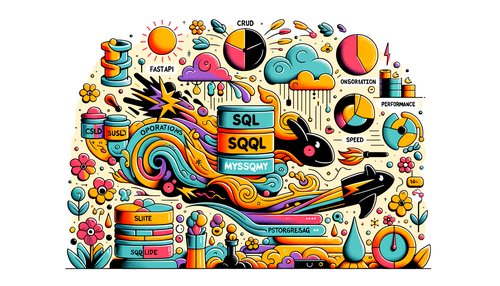