Unlocking the Secrets of Python Typing: How Strong Typing Elevates Your Code
Welcome to a journey into the heart of Python typing, a feature that, when leveraged properly, can significantly elevate the quality, readability, and robustness of your code. In this comprehensive exploration, we'll uncover the secrets of strong typing in Python, demystify its complexities, and provide you with actionable insights to harness its power in your daily coding activities. Whether you're new to Python or a seasoned developer looking to refine your skills, this guide will offer valuable perspectives on making the most of Python's typing system.
The Basics of Python Typing
Python is a dynamically typed language, which means that the type of a variable is determined at runtime. However, from version 3.5 onwards, Python introduced optional type hints, allowing developers to explicitly state the expected type of variables, function arguments, and return values. This system, known as type annotation, serves as a foundation for static type checking in Python, offering a blend of dynamic typing flexibility with the benefits of static type safety.
Example:
def greet(name: str) -> str: return f"Hello, {name}"
This simple example illustrates how type hints can make the function's expectations clear: name
should be a string, and the function will return a string.
Why Embrace Strong Typing?
Strong typing in Python might seem like an optional luxury at first glance, but it comes with a plethora of benefits:
- Improved Code Quality: Type hints make your code more readable and self-documenting. By knowing the expected types, developers can understand the codebase faster and more accurately.
- Error Reduction: Static type checking tools like Mypy can catch type-related errors at an early stage, reducing runtime errors and making the code more reliable.
- Better Development Experience: Modern IDEs use type hints to provide more accurate code completion, error detection, and refactoring tools.
Practical Tips for Using Type Annotations
Here are some practical tips to get the most out of type annotations in Python:
- Start Small: If you're new to typing, start by adding type hints to new functions and variables. Gradually, you can add annotations to existing code during refactoring sessions.
- Use Generic Types for Collections: Python's
typing
module provides generic types likeList
,Dict
, andSet
that you can annotate with specific types to indicate the contents of collections. - Leverage Third-Party Libraries: Many popular libraries and frameworks are now supporting type hints. Use their type stubs or annotations to ensure compatibility and enhance type checking.
Advanced Typing Features
As you become more comfortable with basic type annotations, Python offers advanced typing features that can handle more complex scenarios:
- Union Types: Use
Union
when a variable can be of multiple types. Python 3.10 introduces the|
operator for a cleaner syntax. - Type Aliases: Simplify complex type hints by defining them as a type alias. This is particularly useful for complex nested types.
- Callable Types: Specify the signature of callback functions or any callable object using
Callable
.
Integrating Type Checking into Your Workflow
Static type checking is not part of the standard Python runtime. To reap the benefits of strong typing, integrate a static type checker like Mypy into your development workflow. Run it as part of your testing or continuous integration process to catch type errors before they make it to production.
Conclusion
Python's typing system offers a powerful toolset for improving your code's quality, reliability, and maintainability. By starting with basic type annotations and gradually adopting more advanced features, you can significantly enhance your development experience and output. Remember, the journey to mastering Python typing is a progressive one—start small, be consistent, and keep exploring the depths of this robust feature.
Embrace strong typing in Python, and let it elevate your code to new heights.
Recent Posts
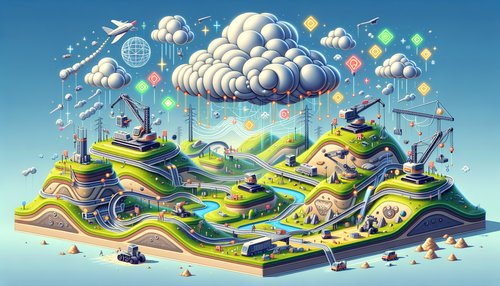
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
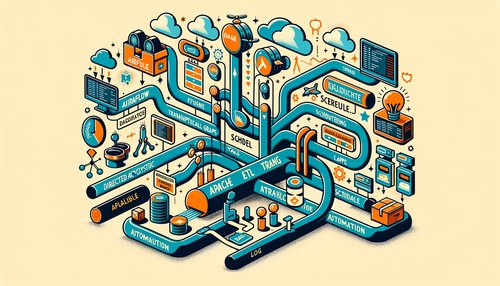
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow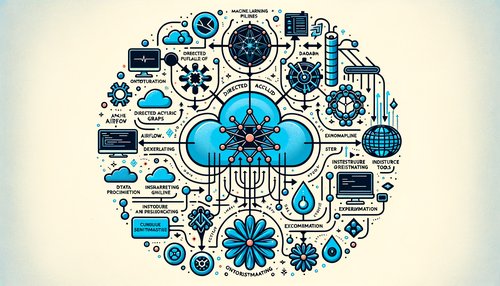
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow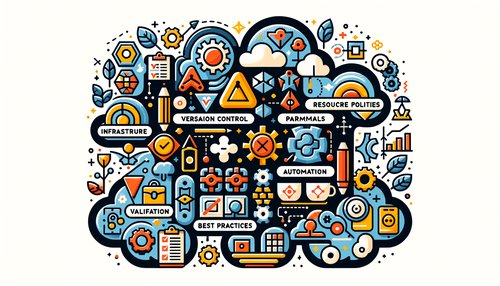
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
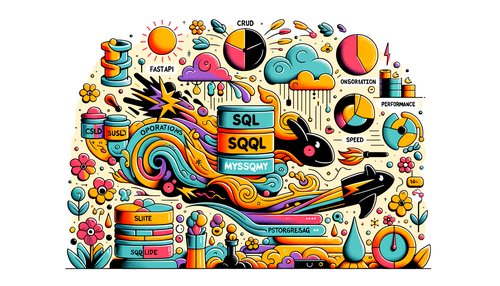