Unlocking Secure User Management in FastAPI: Your Ultimate Guide to Implementing GetCurrent User Functionality
Welcome to the definitive guide on securing and managing user sessions in FastAPI, a high-performance web framework for building APIs with Python 3.7+. In this comprehensive post, we'll explore how to effectively implement the GetCurrent User functionality, a critical component for any application that requires user authentication and authorization. Whether you're building a new project or looking to enhance an existing one, this guide will equip you with the knowledge and tools to ensure your user management is both secure and efficient.
Understanding FastAPI's Authentication Mechanisms
Before diving into the GetCurrent User functionality, it's essential to understand the authentication mechanisms available in FastAPI. FastAPI provides support for various authentication schemes, including OAuth2 with Password (and hashing), Bearer with JWT tokens, and more. These mechanisms are designed to protect your API endpoints by ensuring that only authenticated users can access them.
Practical Tip: Always use HTTPS in production to secure the authentication tokens in transit.
Setting Up User Authentication
Implementing user authentication is the first step towards managing user sessions. This involves creating user models, hashing passwords, and generating tokens. FastAPI's security utilities and dependencies make it straightforward to set up these functionalities.
Example:
from fastapi import FastAPI, Depends, HTTPException, status from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm from passlib.context import CryptContext app = FastAPI() pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto") oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") # Your user authentication logic here
Insight: Utilizing libraries such as Passlib for password hashing can significantly enhance the security of your user passwords.
Integrating GetCurrent User Functionality
Once your authentication system is in place, the next step is to implement the GetCurrent User functionality. This feature allows your application to identify the current user based on their authentication token and provide a personalized experience or restrict access to certain resources.
Example:
from fastapi import Depends, HTTPException from jose import JWTError, jwt from models import User from fastapi.security import OAuth2PasswordBearer oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") async def get_current_user(token: str = Depends(oauth2_scheme)): credentials_exception = HTTPException( status_code=status.HTTP_401_UNAUTHORIZED, detail="Could not validate credentials", headers={"WWW-Authenticate": "Bearer"}, ) try: payload = jwt.decode(token, SECRET_KEY, algorithms=[ALGORITHM]) username: str = payload.get("sub") if username is None: raise credentials_exception token_data = TokenData(username=username) except JWTError: raise credentials_exception user = get_user(db, username=token_data.username) if user is None: raise credentials_exception return user
Practical Tip: Ensure your JWT secret key is stored securely and is not hard-coded in your application code.
Protecting Endpoints and Managing Roles
With the GetCurrent User functionality in place, you can now protect your API endpoints by requiring a valid user token. FastAPI makes it easy to add dependencies that check for the current user before allowing access to an endpoint. Additionally, managing user roles and permissions becomes straightforward, allowing for more granular access control.
Example:
from fastapi import Depends, HTTPException @app.get("/items/") async def read_items(current_user: User = Depends(get_current_user)): # Your endpoint logic here, knowing `current_user` is authenticated
Insight: Leveraging FastAPI's dependency injection system for role management allows for cleaner and more maintainable code.
Summary
In this post, we've explored how to secure and manage user sessions in FastAPI by implementing the GetCurrent User functionality. From setting up user authentication to protecting endpoints and managing roles, we've covered the essential steps to ensure your application's user management is both secure and efficient. Remember, the security of your application is paramount, and implementing these features correctly is critical to protecting your users' data.
As a final thought, consider continuously reviewing and updating your authentication mechanisms to keep up with the latest security best practices. Happy coding!
Recent Posts
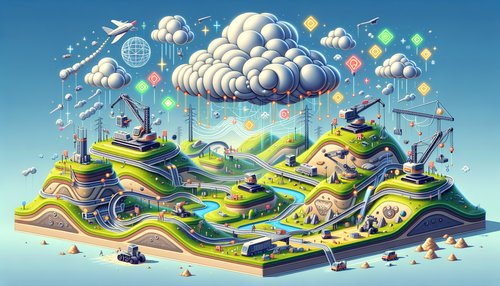
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
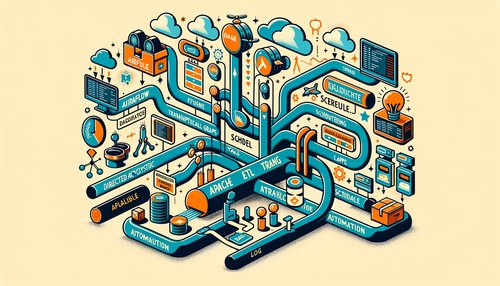
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow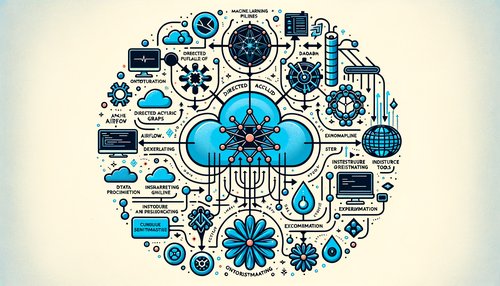
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow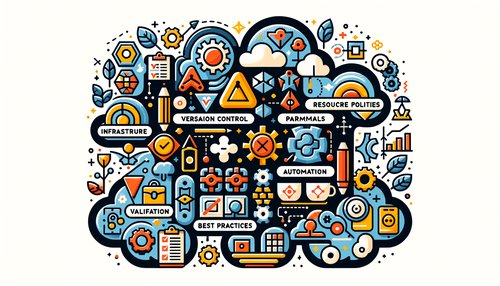
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
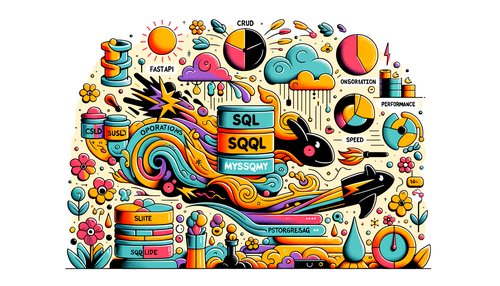