Unlocking Robust Security in FastAPI: A Comprehensive Guide to OAuth2, Password Hashing, and Bearer JWT Tokens
Welcome to the frontier of web application security, where the safeguarding of user data and ensuring secure access control are paramount. In this comprehensive guide, we'll dive deep into the mechanisms of OAuth2, password hashing, and bearer JWT tokens within the context of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. Whether you're a seasoned developer or just starting out, this guide aims to equip you with the knowledge and tools to enhance the security of your FastAPI applications.
Understanding OAuth2 with FastAPI
OAuth2 is the industry-standard protocol for authorization. It focuses on client developer simplicity while providing specific authorization flows for web applications, desktop applications, mobile phones, and living room devices. Integrating OAuth2 with FastAPI allows you to authorize users and provide secure access to your application's data.
Practical Tip: Start by registering your application with an OAuth2 provider (e.g., Google, Facebook) to obtain the necessary credentials (client ID and client secret) for the OAuth2 flow.
FastAPI simplifies the implementation of OAuth2 by providing a comprehensive security module. To integrate OAuth2 in your FastAPI application, you'll need to define the security scheme using OAuth2PasswordBearer
or OAuth2PasswordRequestForm
, depending on your requirements.
from fastapi import Depends, FastAPI, HTTPException, status from fastapi.security import OAuth2PasswordBearer app = FastAPI() oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") @app.post("/token") async def token(): return {"token": "your_token_here"}
Password Hashing: Essential for Protecting User Data
Password hashing is a critical aspect of securing your application. It transforms the password entered by the user into a fixed-size string of characters, which is virtually impossible to reverse. FastAPI does not enforce a specific hashing algorithm, giving you the flexibility to choose one that best fits your security requirements.
Practical Tip: Use passlib
, a Python library that provides password hashing utilities, to hash and verify passwords securely. The bcrypt
algorithm is highly recommended for its balance between security and performance.
from passlib.context import CryptContext pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto") def hash_password(password: str): return pwd_context.hash(password) def verify_password(plain_password: str, hashed_password: str): return pwd_context.verify(plain_password, hashed_password)
Bearer JWT Tokens: The Key to Efficient Authentication
JSON Web Tokens (JWT) offer a compact and self-contained way for securely transmitting information between parties as a JSON object. In the context of FastAPI, utilizing bearer JWT tokens is a common practice for handling user authentication and authorization.
Practical Tip: Use the PyJWT
library to generate and verify JWT tokens in your FastAPI application. Ensure to keep your secret key safe and rotate it periodically to maintain token integrity.
import jwt from datetime import datetime, timedelta SECRET_KEY = "your_secret_key" ALGORITHM = "HS256" def create_access_token(data: dict): to_encode = data.copy() expire = datetime.utcnow() + timedelta(minutes=60) to_encode.update({"exp": expire}) encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM) return encoded_jwt
Conclusion
In this guide, we've explored the foundational elements of securing FastAPI applications through OAuth2, password hashing, and bearer JWT tokens. By implementing these strategies, you can significantly enhance the security posture of your web applications, protecting both user data and access to resources.
Remember, security is an ongoing process, not a one-time setup. Continuously update your knowledge on security best practices, monitor your applications for any vulnerabilities, and adapt to the ever-evolving threat landscape.
Now that you're equipped with the knowledge of these powerful security mechanisms, it's time to put them into practice. Secure your FastAPI applications and ensure a safer digital experience for your users. Happy coding!
Recent Posts
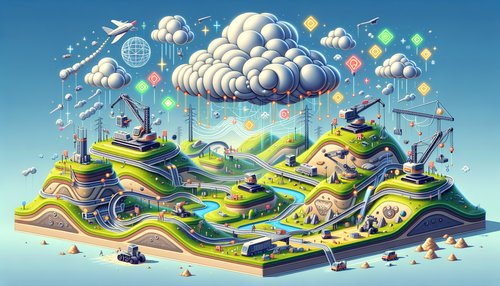
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
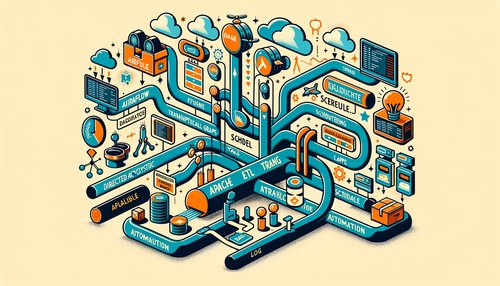
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow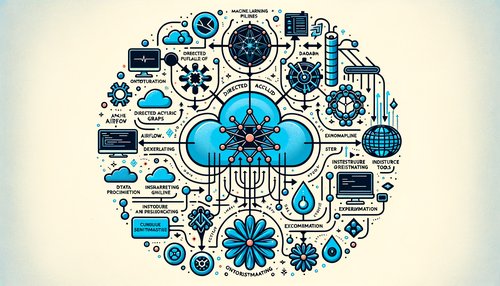
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow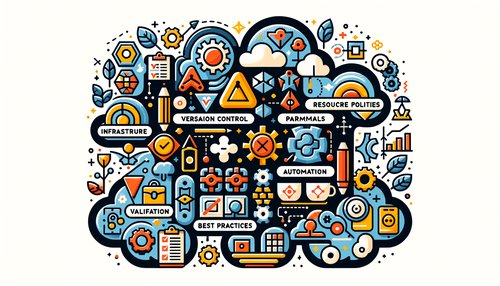
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
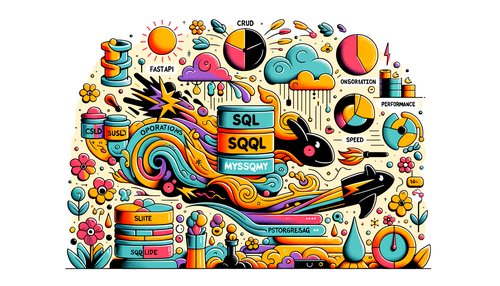