Unlocking Real-Time Efficiency: A Deep Dive into Background Tasks with the FastAPI User Guide
In today’s fast-paced digital world, efficiency and speed are the cornerstones of a successful application. One of the key strategies to achieve this is by leveraging background tasks, a concept that allows for non-blocking operations within your applications. This blog post will take you through an insightful journey into the world of background tasks within FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. FastAPI provides a seamless approach to implementing background tasks, making your applications more efficient and responsive. Let’s dive into how you can unlock real-time efficiency by mastering background tasks with the FastAPI user guide.
Understanding Background Tasks
Before we delve into the specifics of FastAPI, it’s crucial to understand what background tasks are and why they are important. Background tasks are operations that run asynchronously, separate from the main execution thread of your application. This means your application can continue processing requests and responding to users while the background task handles time-consuming operations like sending emails, processing data, or calling external APIs. This parallel processing capability is vital for improving the user experience and scaling your application.
Getting Started with Background Tasks in FastAPI
FastAPI simplifies the process of implementing background tasks, making it accessible even to those new to asynchronous programming. To get started, you first need to import BackgroundTasks
from FastAPI. You can then add a background task to any endpoint by including it as a parameter in your function. FastAPI will handle the execution of these tasks in the background, allowing your main application to run uninterrupted.
from fastapi import FastAPI, BackgroundTasks
app = FastAPI()
def write_log(message: str):
# Imagine this writes to a log file
with open("log.txt", "a") as log:
log.write(message + "\n")
@app.post("/send-notification/")
async def send_notification(email: str, background_tasks: BackgroundTasks):
background_tasks.add_task(write_log, f"Notification sent to {email}")
return {"message": "Notification sent in the background"}
Advanced Background Task Management
While adding background tasks in FastAPI is straightforward, managing more complex workflows requires a deeper understanding. For example, you might need to ensure that tasks are completed before shutting down the server, or you might want to prioritize certain tasks over others. FastAPI’s background tasks are built on top of Starlette, which means you can use Starlette’s event loop and task management features for more advanced control over your background operations.
Best Practices for Background Tasks
- Task Isolation: Keep your background tasks isolated from your main application logic. This separation makes it easier to manage tasks and ensures that any failure in a background task does not directly impact the main application flow.
- Error Handling: Implement comprehensive error handling within your background tasks. Since these tasks run asynchronously, unhandled errors can crash your background worker without affecting the main application, leading to silent failures.
- Monitoring and Logging: Always monitor and log the execution of your background tasks. This practice is crucial for debugging and understanding the behavior of your application in production.
Scaling Background Tasks
As your application grows, you may need to scale your background task processing capabilities. FastAPI itself does not limit how you scale, offering flexibility in how you manage background tasks at scale. One common approach is to use a task queue with workers, such as Celery, to distribute tasks across multiple workers. This method allows for horizontal scaling and can significantly improve the efficiency of handling background tasks in large-scale applications.
Conclusion
Background tasks are an essential feature for modern web applications, offering a path to real-time efficiency and improved user experiences. FastAPI provides a powerful yet simple way to implement background tasks in your Python applications. By understanding and utilizing the concepts and practices outlined in this guide, you can unlock the full potential of asynchronous programming, making your applications faster, more responsive, and scalable. Remember, the key to mastering background tasks is practice and continuous learning. So, start experimenting with FastAPI’s background tasks today and take your applications to the next level.
As we conclude this deep dive, consider integrating background tasks into your next FastAPI project. Not only will it enhance the performance of your applications, but it will also provide you with a deeper understanding of asynchronous programming in Python. Happy coding!
Recent Posts
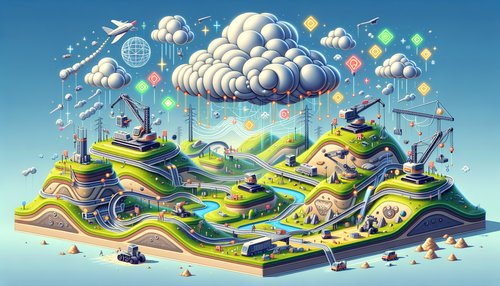
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
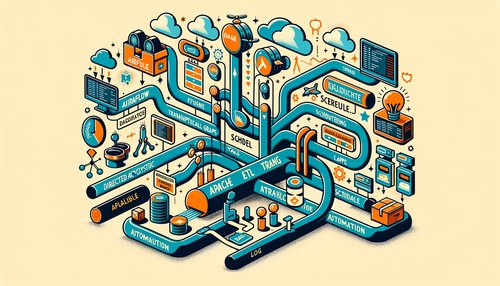
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow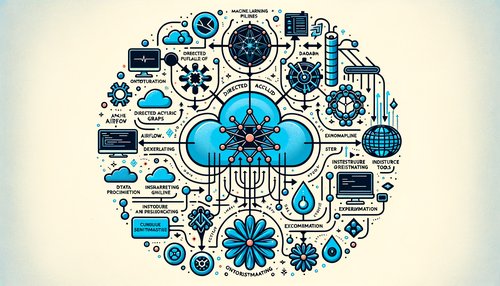
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow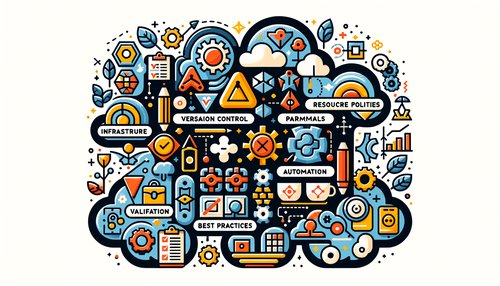
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
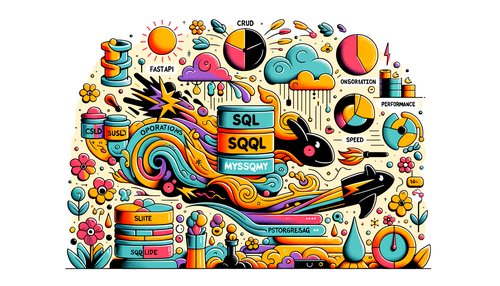