Exploring the RxJS Framework
Reactive programming is becoming an increasingly popular way of developing applications. RxJS is a popular library for reactive programming, and it provides a powerful way to handle asynchronous data streams and data flows. In this blog post, we'll take a look at the basics of RxJS and explore why it's such a powerful tool for application development.
What is RxJS?
RxJS is a library for reactive programming. It provides an API for creating and manipulating data streams, which are sequences of data over time. RxJS makes it easy to handle asynchronous data flows, such as user input or network requests, by providing operators that can be chained together to manipulate the data.
What are the Benefits of Using RxJS?
The main benefit of using RxJS is that it allows developers to easily handle asynchronous data flows. Using RxJS, developers can quickly create data streams, manipulate them, and subscribe to them. This makes it easy to handle complex data flows, such as user input or network requests.
RxJS also makes it easier to reason about asynchronous data flows. By using the RxJS operators, developers can easily chain together data transformations and ensure that the data flows are predictable.
How to Get Started with RxJS
Getting started with RxJS is easy. All you need is a basic understanding of JavaScript and a text editor.
First, create a new project and install RxJS. To do this, run the following command in your terminal:
npm install rxjs
Once RxJS is installed, you can start writing code. To create a new stream, use the Observable
constructor:
const stream = new Observable(observer => {
observer.next('Hello World!');
});
Once you have created a stream, you can manipulate it using the RxJS operators. To do this, you can use the pipe
method:
const transformedStream = stream.pipe(
map(value => value.toUpperCase()),
filter(value => value.length > 5)
);
Finally, you can subscribe to the stream and handle the data:
transformedStream.subscribe(value => {
console.log(value);
});
Conclusion
RxJS is a powerful library for reactive programming, and it provides a great way to handle asynchronous data flows. With its operators and easy-to-use API, developers can quickly create data streams, manipulate them, and subscribe to them. RxJS is an invaluable tool for application development, and it's worth exploring further if you're interested in reactive programming.
Recent Posts
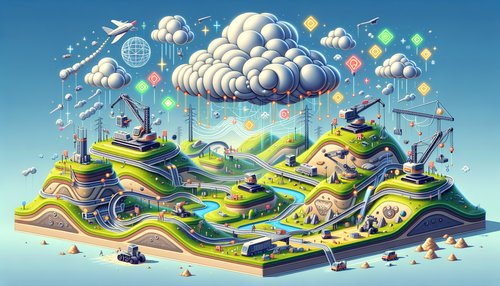
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
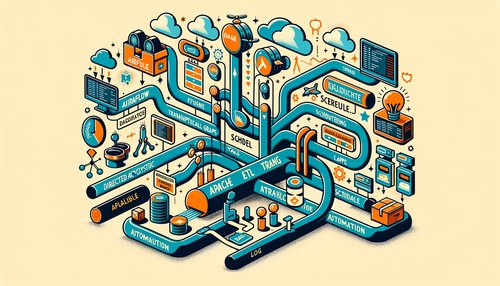
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow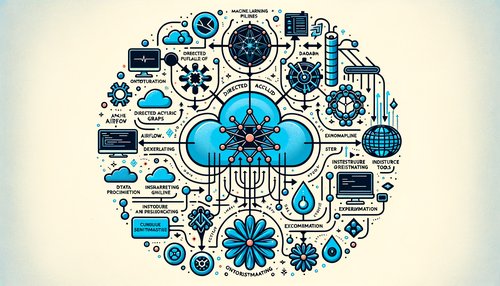
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow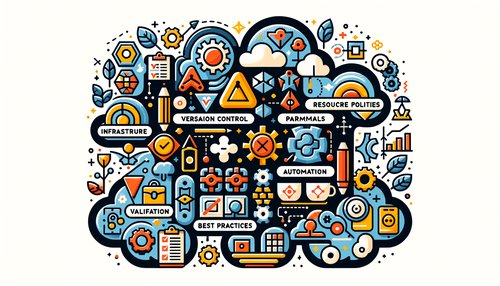
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
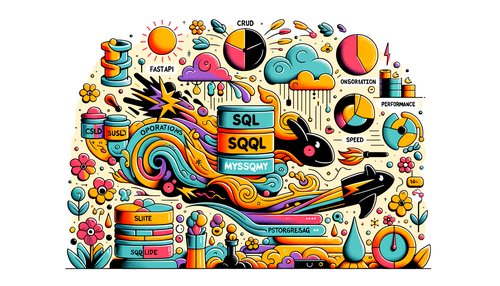