Unlock the Power of Data: Mastering Pandas IO Tools for Text, CSV, HDF5 and Beyond!
Data is the lifeblood of the modern digital world, driving insights, innovation, and decision-making across every sector. However, the raw data itself is often unwieldy and inaccessible without the right tools to unlock its potential. Enter Pandas: a powerful Python library that has become synonymous with data manipulation and analysis. In this comprehensive guide, we'll dive deep into the heart of Pandas IO tools, focusing on how to effectively work with text, CSV, HDF5 formats, and explore what lies beyond. Prepare to master the art of data handling and transform the way you interact with datasets!
Getting Started with Pandas for Data Import and Export
Before we delve into the specifics, it's essential to understand the basics of Pandas and its IO capabilities. Pandas provides a robust set of tools for reading data from various sources, including but not limited to text files, CSVs, Excel spreadsheets, SQL databases, and HDF5 formats. These tools are designed to facilitate the process of data ingestion, making it seamless to convert raw data into a more manageable form - the DataFrame.
Working with Text and CSV Files
Text and CSV (Comma Separated Values) files are among the most common formats for storing and sharing data. Luckily, Pandas makes it incredibly easy to load and manipulate these files using read_csv()
and to_csv()
functions. Here's a quick primer:
import pandas as pd
# Reading a CSV file
df = pd.read_csv('path/to/your/file.csv')
# Writing a DataFrame to a CSV file
df.to_csv('path/to/your/newfile.csv', index=False)
These functions are highly customizable, allowing you to handle various nuances of CSV files, such as different delimiters, quoting conventions, and file encodings.
Leveling Up with HDF5
For those dealing with large datasets that don't fit into memory, HDF5 can be a game-changer. HDF5 files offer a hierarchical structure for storing data, which can be particularly useful for organizing complex datasets. Pandas supports HDF5 through the HDFStore
class, enabling efficient read/write operations:
# Writing to HDF5
store = pd.HDFStore('my_data.h5')
store['my_dataframe'] = df # Saving the DataFrame
store.close()
# Reading from HDF5
store = pd.HDFStore('my_data.h5')
df = store['my_dataframe']
store.close()
This format is not only efficient for large datasets but also supports querying, making it possible to retrieve specific subsets of the data without loading the entire dataset into memory.
Exploring Beyond: SQL, Excel, and More
Pandas' capabilities are not limited to text, CSV, and HDF5. It also provides functions for interacting with SQL databases, Excel files, and even JSON. Whether you're pulling data from a SQL server using read_sql()
, importing an Excel spreadsheet with read_excel()
, or parsing a JSON file using read_json()
, Pandas has you covered. These tools open up a world of possibilities for data analysis and integration, enabling you to pull together diverse data sources into a unified analysis framework.
Best Practices for Efficient Data Handling
While Pandas provides powerful tools for data IO, there are best practices to ensure efficiency, especially with large datasets:
- Use chunking: When dealing with very large files, read them in chunks instead of loading the entire dataset into memory.
- Specify data types: When possible, specify column data types upfront to avoid the overhead of type inference.
- Consider compression: When reading or writing data, consider using compression (e.g.,
compression='gzip'
) to save disk space and potentially speed up IO operations.
Conclusion
Mastering Pandas IO tools is a critical step towards unlocking the full potential of your data. By understanding how to efficiently import and export data in various formats, you can streamline your data analysis workflow, handle large datasets with ease, and integrate diverse data sources. Whether you're working with text files, CSVs, HDF5, or exploring other data storage options, the flexibility and power of Pandas can help you achieve your data handling goals. So go ahead, dive into your data with Pandas, and unlock insights that were previously out of reach!
Remember, the journey of data analysis is ongoing, and there's always more to learn. Keep experimenting, keep learning, and most importantly, keep sharing your knowledge with the community. Happy data wrangling!
Recent Posts
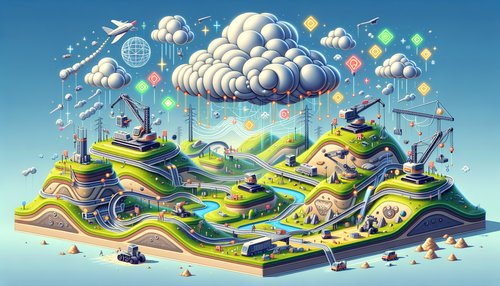
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
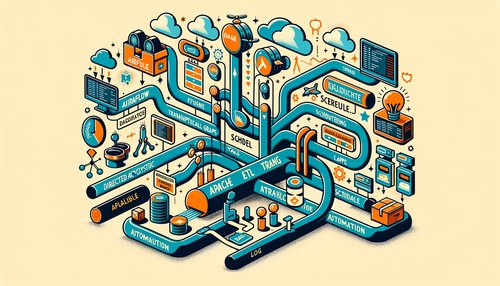
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow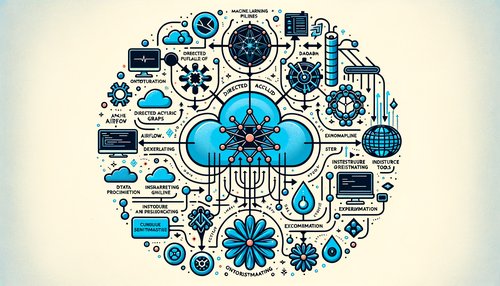
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow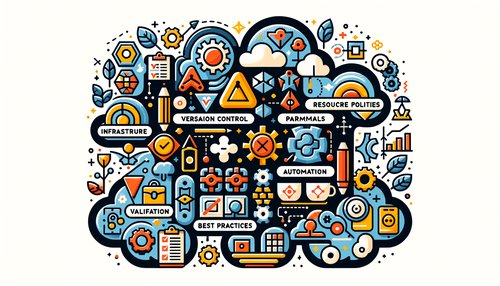
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
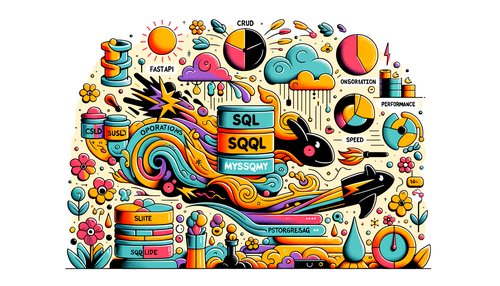