Unlock the Power of Angular Modules: Create Dynamic Applications with Ease!
Are you looking for a way to create high-performance, dynamic applications with ease? Look no further than Angular Modules. Angular Modules are a powerful tool for developers that allow them to create robust applications quickly and easily. In this blog post, we'll explore the power of Angular Modules and how they can help you create dynamic applications with ease.What are Angular Modules?
Angular Modules are a way to organize and structure an application. They allow you to divide your application into smaller, more manageable pieces. This makes it easier to maintain and debug your application. Angular Modules also enable you to create reusable components that can be used across multiple applications.Benefits of Angular Modules
There are numerous benefits of using Angular Modules in your application. Let's take a look at some of the most important ones.- Organized code: Angular Modules help you to organize your codebase and keep it organized. This makes it easier to find and debug any issues.
- Reusable components: Angular Modules allow you to create reusable components that can be used in multiple applications.
- Efficient loading: Angular Modules help to reduce the loading time of your application by only loading the necessary components.
- Ease of maintenance: Modular structure makes it easier to maintain and update your application.
How to Use Angular Modules
Using Angular Modules is quite straightforward. To create a module, you define a class with the @NgModule decorator. This class will contain the components, directives, and services that make up the module.@NgModule({ declarations: [ MyComponent, MyDirective, MyService ], imports: [ // Module dependencies ], providers: [], bootstrap: [MyComponent] }) export class MyModule { }Once you have defined your module, you can import it in other modules and use the components, directives, and services it contains.
Conclusion
Angular Modules are a powerful tool for developers that can help them create dynamic applications with ease. They allow you to organize and structure your application, create reusable components, and efficiently load components. If you have an application that needs to be organized and optimized, then Angular Modules are the way to go.Recent Posts
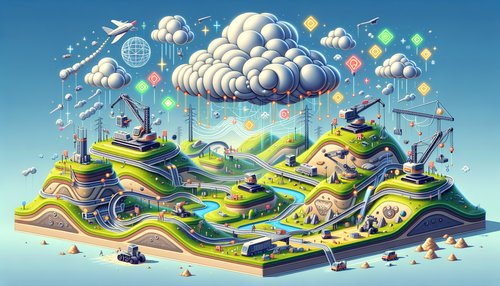
7 months, 2 weeks ago
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
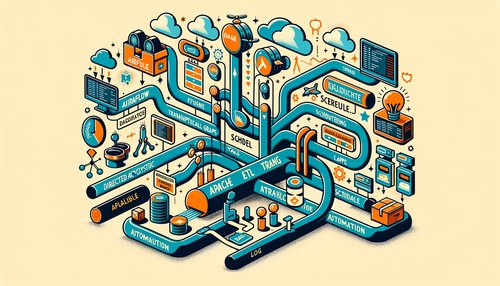
7 months, 3 weeks ago
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow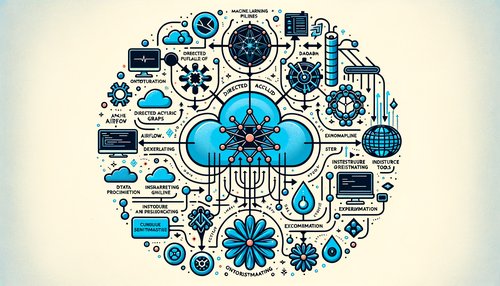
7 months, 3 weeks ago
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow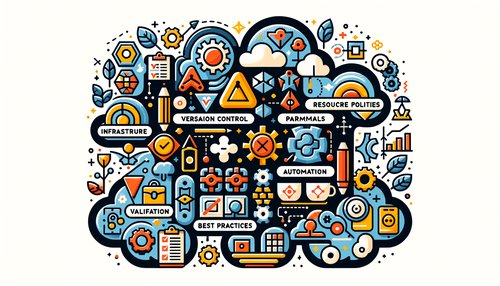
7 months, 3 weeks ago
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
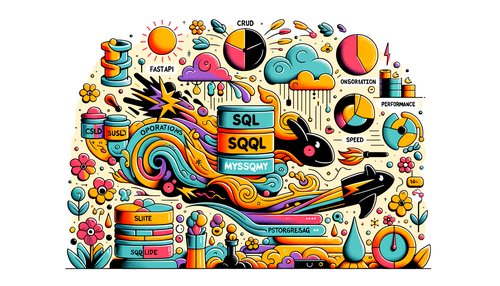
7 months, 3 weeks ago
Show All