Unlocking the Power of FastAPI: A Deep Dive into User Guides and Leveraging Extra Models for Seamless Development
Welcome to a comprehensive exploration of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. In this blog post, we'll embark on a journey to unlock the full potential of FastAPI, guiding you through its intricacies and showing you how to leverage its features for a seamless development experience. Whether you're a beginner eager to dive into the world of APIs or an experienced developer looking to enhance your toolkit, this post is tailored for you.
Introduction to FastAPI
FastAPI is an innovative web framework that simplifies the process of building APIs while ensuring they are fast, reliable, and easy to use. Its design, centered around Python 3.7+'s type hints, enables automatic request validation, serialization, and documentation. This introduction will cover the basics of FastAPI, setting the stage for a deeper exploration of its capabilities.
Getting Started with FastAPI
To kick things off, let's look at how to set up a basic FastAPI project. This involves installing FastAPI and Uvicorn, an ASGI server, to run your application. A simple API example will demonstrate how to define routes, accept parameters, and return responses. This section aims to provide a solid foundation for newcomers to get up and running with FastAPI.
pip install fastapi uvicorn
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
Running this app with uvicorn your_file_name:app --reload
will start a local server, showcasing the simplicity and power of FastAPI.
Deep Diving into User Guides
FastAPI's comprehensive user guides are a treasure trove of information, covering everything from request validation and serialization to security and database handling. This section delves into these guides, highlighting key features and providing practical examples to illustrate how FastAPI can streamline API development. Topics like Pydantic models for data validation, OAuth2 for security, and background tasks for asynchronous operations will be covered.
Leveraging Extra Models for Seamless Development
One of FastAPI's strengths is its support for Pydantic models, which simplifies data validation, serialization, and documentation. However, FastAPI takes this a step further by allowing developers to leverage extra models for more complex scenarios. This section explores advanced patterns, such as response model declaration, reducing code duplication with model inheritance, and using generic models to handle various data types dynamically.
Example: Advanced Patterns with Extra Models
Consider a scenario where you need to return different sets of data for the same endpoint, based on user roles. FastAPI's extra models feature makes this straightforward, enhancing flexibility and maintainability. This example demonstrates how to define and use extra models to achieve this.
from fastapi import FastAPI, Depends
from pydantic import BaseModel
from typing import Union
class UserBase(BaseModel):
username: str
email: str
full_name: str = None
class UserAdmin(UserBase):
is_active: bool = True
is_superuser: bool = False
app = FastAPI()
@app.get("/users/me", response_model=Union[UserAdmin, UserBase])
async def read_current_user(is_superuser: bool = Depends(check_superuser)):
if is_superuser:
return UserAdmin(...)
return UserBase(...)
This approach ensures that your API remains clean and your codebase DRY (Don't Repeat Yourself), while still being flexible enough to cater to complex requirements.
Conclusion
Throughout this deep dive into FastAPI, we've explored its foundational concepts, delved into its comprehensive user guides, and examined how to leverage extra models for seamless development. FastAPI stands out as a powerful, efficient, and versatile framework for building APIs, offering developers the tools they need to create robust, scalable, and maintainable applications.
As we conclude, remember that the journey to mastering FastAPI doesn't stop here. The framework's extensive documentation, active community, and continuous updates provide a rich ecosystem for growth and exploration. Whether you're building a small project or a large-scale application, FastAPI offers the features and flexibility to meet your needs. So, take what you've learned, experiment with new ideas, and unlock the full potential of your development projects with FastAPI.
Happy coding!
Recent Posts
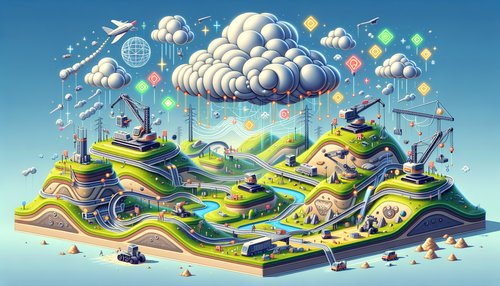
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
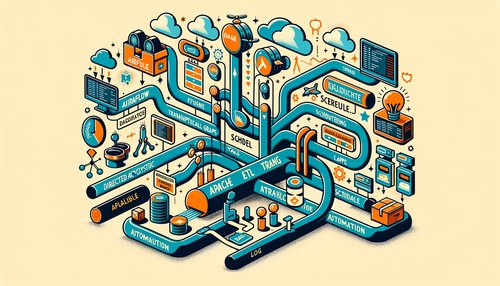
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow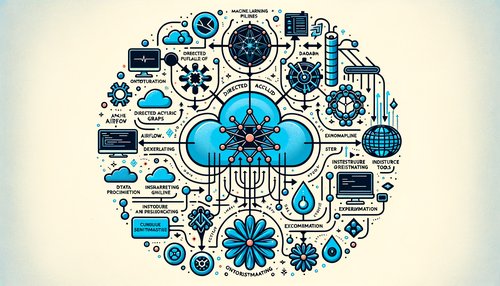
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow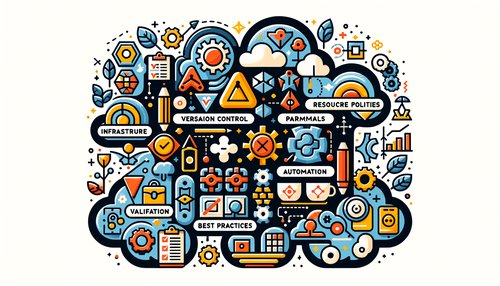
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
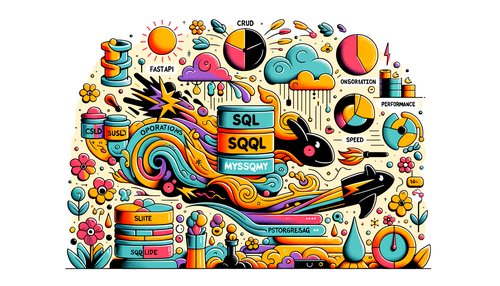