Unlocking Powerful API Development: Master FastAPI with our Comprehensive User Guide on Dependencies and Sub-Dependencies
Welcome to the ultimate guide on leveraging FastAPI for building robust and scalable APIs. In this comprehensive post, we'll dive deep into the world of dependencies and sub-dependencies, crucial elements that can significantly enhance your API development process. Whether you're a seasoned developer or just starting, understanding how to effectively use dependencies in FastAPI will empower you to create more efficient, secure, and maintainable applications. So, let's embark on this journey together and unlock the full potential of your API projects.
Understanding Dependencies in FastAPI
Dependencies in FastAPI are a powerful feature that allows you to create reusable components that can be injected into your path operation functions. This not only promotes code reusability but also helps in maintaining a clean and organized codebase. Dependencies can perform a wide range of functions, from database connections and user authentication to complex validation and business logic.
Practical Tip: Start by defining simple dependencies for tasks you find yourself repeating across different endpoints, such as user authentication checks or database session creation.
Creating Your First Dependency
Creating a dependency in FastAPI is straightforward. You define a function that FastAPI can call whenever a route operation requires it. Here's a basic example:
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
This dependency can then be injected into any path operation function by including it as a parameter:
@app.get("/items/")
async def read_items(db: Session = Depends(get_db)):
return db.query(Item).all()
Going Deeper: Sub-Dependencies
FastAPI's dependency system doesn't stop with direct dependencies; it also supports sub-dependencies. This means a dependency can have its dependencies, creating a chain that FastAPI will resolve for you. This is particularly useful for structuring complex business logic or when dealing with multiple layers of data processing and validation.
Example of Sub-Dependency Implementation
Imagine you have a dependency that requires a database session and another that requires validation of user input. You can chain these dependencies together, so FastAPI resolves them in the correct order:
def get_current_user(db: Session = Depends(get_db), token: str = Depends(oauth2_scheme)):
# Your logic here to return the current user
pass
In this example, get_current_user
becomes a sub-dependency that itself depends on get_db
and another dependency for token validation.
Best Practices for Using Dependencies
While dependencies and sub-dependencies offer immense flexibility and power, they also introduce complexity. Here are some best practices to keep your project manageable:
- Keep dependencies simple and focused: Each dependency should have a clear purpose. Avoid creating large, monolithic dependencies that are hard to maintain and understand.
- Use sub-dependencies judiciously: While sub-dependencies are powerful, they can make your dependency graph complex. Use them when necessary but don't overcomplicate your dependency structure.
- Document your dependencies: Good documentation is crucial, especially for complex dependency chains. Ensure each dependency is well-documented, explaining its purpose, inputs, and outputs.
Conclusion
Mastering dependencies and sub-dependencies in FastAPI can significantly elevate your API development, making your applications more modular, reusable, and maintainable. By understanding the basics of dependency injection and applying best practices, you can harness the full power of FastAPI to create robust and efficient web applications. Remember to start simple, keep your dependencies focused, and document everything well. Happy coding!
As you continue to explore FastAPI and its capabilities, consider revisiting these concepts and experimenting with more complex dependency structures. The more you practice, the more intuitive these powerful features will become, enabling you to craft APIs that stand out in both functionality and design.
Recent Posts
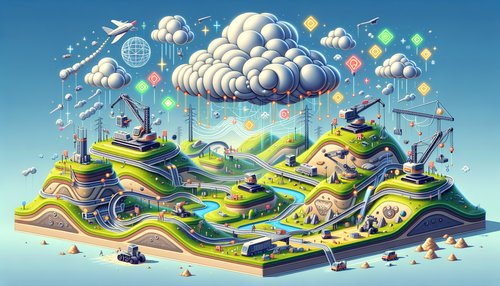
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
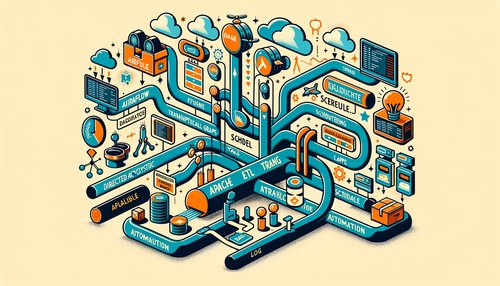
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow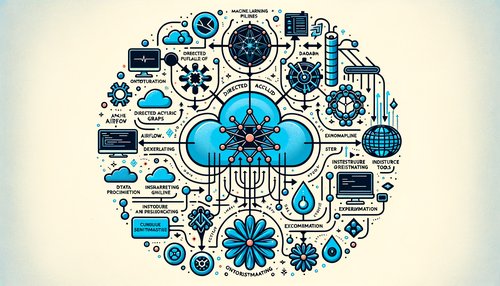
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow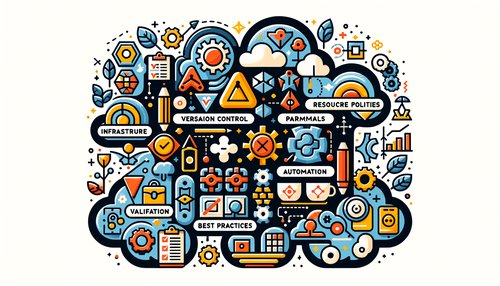
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
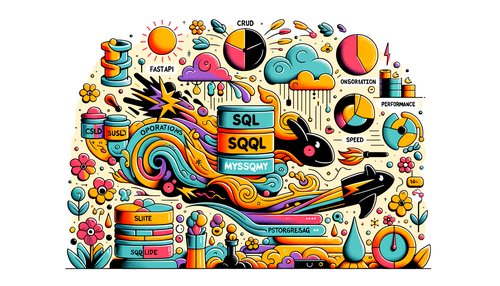