Getting Started with React: Tips and Tricks for Developing with the React Framework
React is a powerful, popular JavaScript library for building user interfaces. It's used by some of the world's biggest companies, including Facebook, Instagram, Netflix, and Airbnb. With its component-based architecture and declarative syntax, React makes it easy to create complex and interactive user interfaces.
If you're new to React, getting started can be a daunting task. But fear not! This blog post will provide you with some tips and tricks to help you get up and running quickly. We'll cover everything from setting up your development environment to writing your first component. Let's get started!
Setting Up Your Environment
The first step in getting started with React is setting up your development environment. You'll need to install Node.js and the React development tools, which include the React library, a development server, and a build system.
Once you've installed the tools, you'll need to create a project folder and initialize it with the npm init
command. This will create a package.json
file, which is used to manage your project's dependencies.
Writing Your First Component
Now that you have your environment set up, it's time to write your first component. Components are the building blocks of React, and they're used to create reusable pieces of code.
To create a component, you'll need to create a JavaScript file and import the React library. Then, you'll use the React.createClass
method to define your component. Here's an example of a simple component:
import React from 'react';
const MyComponent = React.createClass({
render() {
return <div>Hello World!</div>;
}
});
Styling Your Components
Once you've written your component, you'll need to style it. React supports both inline styles and CSS. For inline styles, you can use the style
prop on your component. For example:
const MyComponent = React.createClass({
render() {
return (
<div style={{ backgroundColor: '#000', color: '#fff' }}>
Hello World!
</div>
);
}
});
For more advanced styling, you can use CSS. To do this, you'll need to create a .css
file and import it into your component. For example:
import React from 'react';
import './MyComponent.css';
const MyComponent = React.createClass({
render() {
return <div className="MyComponent">Hello World!</div>;
}
});
Adding Interactivity
The last step in getting started with React is adding interactivity. React components are designed to be dynamic, so you can add interactivity with event handlers. For example, if you wanted to add a click event to a button, you could use the onClick
prop:
const MyComponent = React.createClass({
handleClick() {
console.log('button was clicked');
},
render() {
return (
<button onClick={this.handleClick}>
Click me!
</button>
);
}
});
Wrapping Up
Congratulations! You've now learned the basics of getting started with React. With a few simple steps, you can create complex user interfaces and add interactivity to your components.
Good luck on your React journey!
Recent Posts
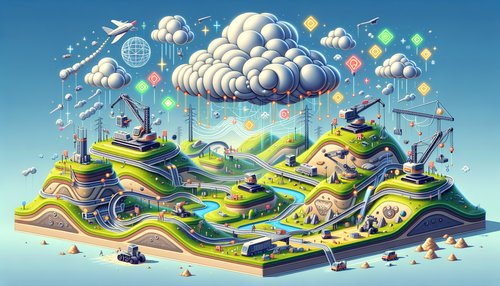
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
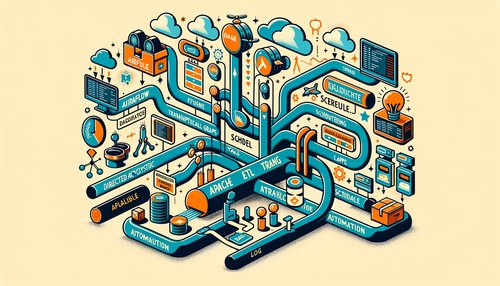
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow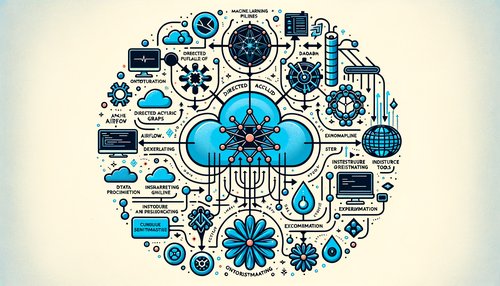
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow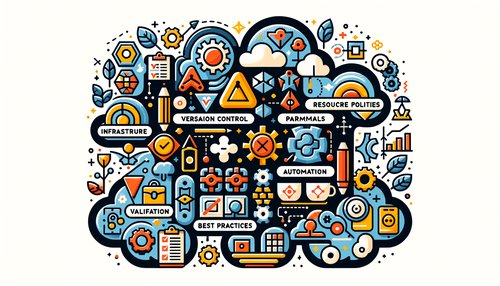
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
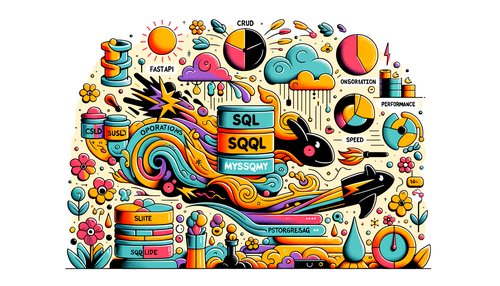