FastAPI local Docker-Compose Setup
To run FastAPI with Docker Compose locally, you will need to follow these steps:
Step 1: Create a new directory for your project and navigate to it in your terminal.
Step 2: Create a new file called Dockerfile
in the project directory and paste the following code into it:
FROM tiangolo/uvicorn-gunicorn-fastapi:python3.9
COPY ./app /app
This will create a Docker image based on the official tiangolo/uvicorn-gunicorn-fastapi
image and copy the contents of the app
directory into the /app
directory in the image.
Step 3: Create a new file called docker-compose.yml
in the project directory and paste the following code into it:
version: '3'
services:
app:
build: .
ports:
- "8000:80"
This will create a new service called app
based on the Docker image we just created, and map port 8000
on our local machine to port 80
in the container.
Step 4: Create a new directory called app
in the project directory, and create a new file called main.py
inside it. This file should contain your FastAPI code.
Step 5: Start the container by running the following command in your terminal:
docker-compose up
This will build the Docker image and start the container. You should be able to access your FastAPI app by going to http://localhost:8000
in your web browser.
Here's the final directory structure of your project:
.
├── Dockerfile
├── docker-compose.yml
└── app
└── main.py
And here's an example main.py
file that you can use to test the setup:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"message": "Hello, World!"}
With this setup, you should be able to run and test your FastAPI app locally using Docker Compose.
Recent Posts
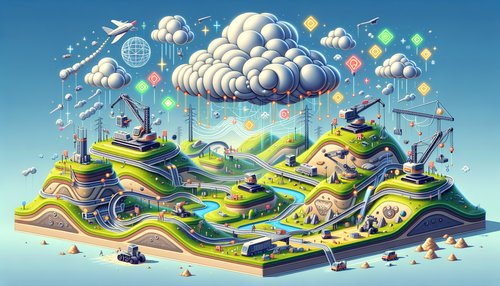
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
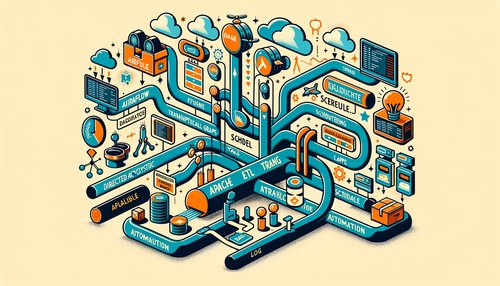
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow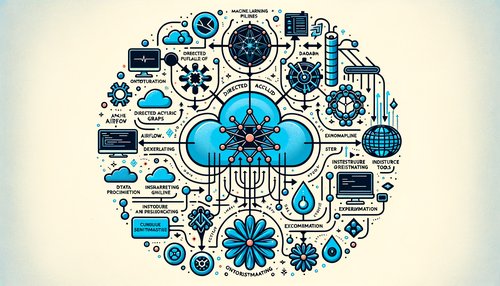
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow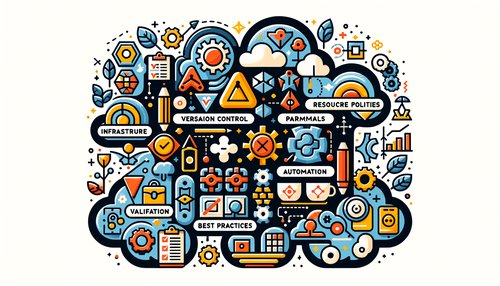
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
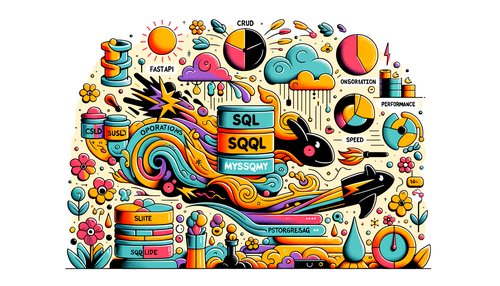