How Django Signals Can Help Streamline Your Web Application Development
Django Signals are an invaluable tool for streamlining your web application development. Django Signals allow developers to create custom events that can be triggered by certain actions in the application. This can be incredibly useful for automating certain tasks or for responding to specific user actions.
For example, let's say you have a web application that requires users to submit a form. You can use Django Signals to trigger a callback when a user submits the form. This allows you to do things like send an email to the user or log the submission in a database.
Using Django Signals can help you automate certain tasks and respond to user actions quickly and easily. This can help reduce development time and make your application more efficient.
Using Django Signals is fairly simple. All you need to do is define the signal and its callback function. Here is an example of how to do this:
from django.dispatch import Signal
form_submitted = Signal(providing_args=["user", "form_data"])
def on_form_submitted(sender, **kwargs):
user = kwargs['user']
form_data = kwargs['form_data']
# do something with user and form_data
form_submitted.connect(on_form_submitted)
In this example, we are creating a signal called form_submitted
and connecting it to a callback function called on_form_submitted
. When the signal is triggered, the callback function will be called with the user and form data as arguments.
Once the signal has been created and connected to a callback, you can trigger the signal whenever a user submits a form. This will automatically call the callback function and execute the code inside.
Using Django Signals can help streamline your web application development by allowing you to automate certain tasks and respond to user actions quickly and easily. They are a great tool for reducing development time and making your application more efficient.
Recent Posts
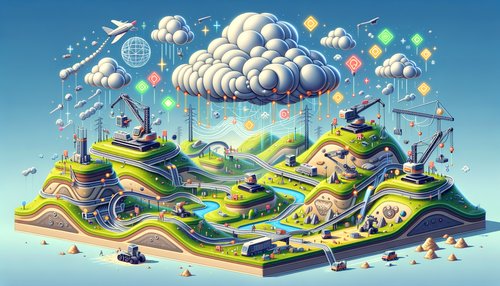
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
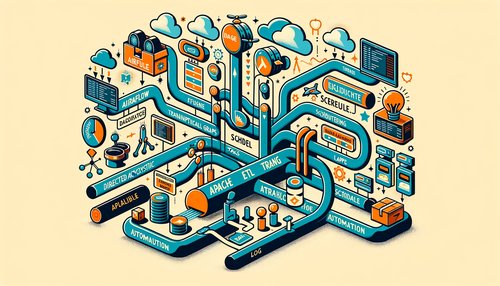
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow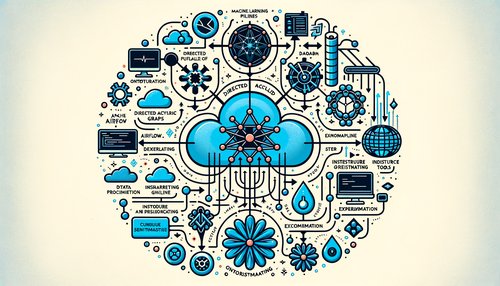
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow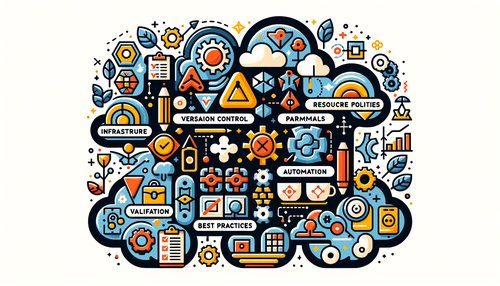
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
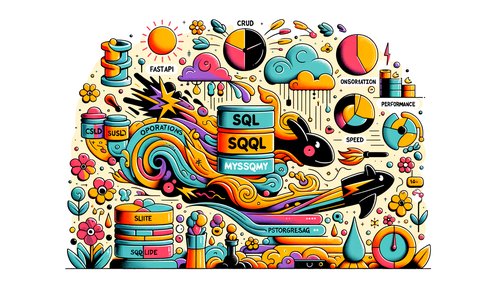