Mastering Angular Routing: Navigate Your Way to Seamless Web Applications
Welcome to the definitive guide on mastering Angular routing! Angular, a platform and framework for building single-page client applications using HTML and TypeScript, provides a robust set of tools for managing navigation and URLs. In this comprehensive post, we will delve into the intricacies of Angular routing, offering practical tips, illustrative examples, and key insights to help you navigate your way to creating seamless web applications. Whether you're a seasoned Angular developer or just starting out, this guide aims to equip you with a deeper understanding of routing concepts, techniques, and best practices.
Understanding Angular Routing
At its core, Angular routing allows us to define navigation paths within our application. It enables us to map our application's components to different URLs, making it possible to bookmark and share URLs that lead directly to specific states of the application. This is essential for creating a user-friendly, navigable web application. Angular's Router module serves as the backbone of this functionality, handling navigation from one view to the next as users perform application tasks.
Setting Up and Configuring Routes
Getting started with Angular routing involves setting up and configuring routes. This process typically begins with importing the RouterModule
and defining routes in your application module. A route in Angular is defined as an object with at least two properties: a path and a component. The path is a string that represents the URL fragment, while the component is the Angular component that should be displayed when the route is activated.
const appRoutes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
After defining your routes, the next step is to add the RouterModule
to your application's imports, passing your routes to it through the forRoot()
method. This integrates routing capability into your application.
Router Outlet and Navigation
The <router-outlet>
directive acts as a placeholder that Angular dynamically fills based on the current router state. It is where your component will be displayed when its route is navigated to. Navigation can be achieved programmatically using the Router
service or declaratively with the <a routerLink="/path">
directive.
Advanced Routing Features
Angular's routing capabilities go beyond basic setup and configuration. Features such as route parameters allow for dynamic routing, where you can pass and retrieve parameters through the URL. Guarding routes with CanActivate
and CanDeactivate
guards ensures that certain criteria are met before a route is activated or deactivated, adding a layer of security and control over the navigation flow of your application.
Lazy Loading Modules
One of Angular's powerful optimization techniques is lazy loading, which involves loading feature modules on demand rather than at the initial loading of the application. This can significantly reduce the initial load time of your application. Implementing lazy loading requires configuring your routes to define a path to a module and using the loadChildren
property instead of a component.
Practical Tips and Best Practices
When working with Angular routing, there are several best practices to keep in mind:
- Organize your routes in a dedicated module when your application grows in complexity.
- Use route guards to protect routes that require authentication or authorization.
- Employ lazy loading to improve your application's performance.
- Make use of the
data
property in route definitions to store custom data associated with a route.
Additionally, always keep your routing configuration clean and well-documented to ensure that it's easy to manage and understand, especially in large applications.
Conclusion
Angular routing is a cornerstone feature for developing single-page applications, offering a sophisticated mechanism for managing navigation and URL manipulation. By understanding and implementing the concepts, techniques, and best practices outlined in this guide, you'll be well on your way to mastering Angular routing. This will not only enhance the user experience of your web applications but also improve their performance and maintainability. Remember, the journey to mastering Angular routing is ongoing, and there's always more to learn and explore. So, keep experimenting, keep learning, and most importantly, keep building amazing applications with Angular.
As we conclude this guide, consider revisiting your current projects or starting a new one to apply and reinforce what you've learned. The path to mastering Angular routing is a journey best traveled by doing. Happy coding!
Recent Posts
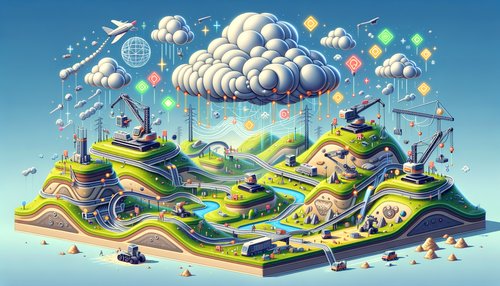
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
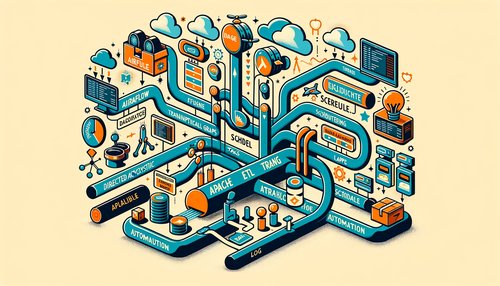
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow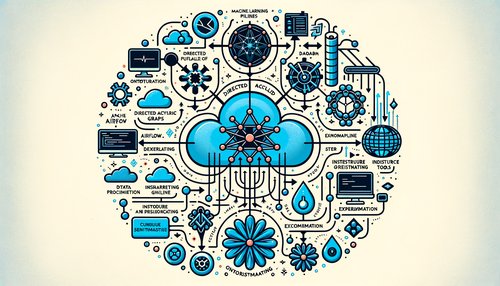
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow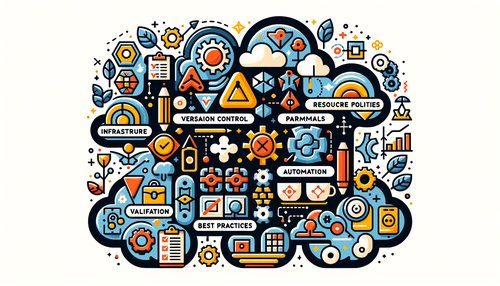
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
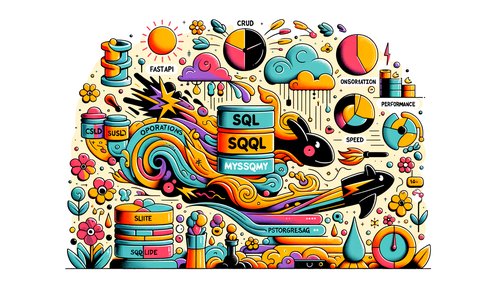