Mastering Background Tasks with FastAPI: A Comprehensive User Guide
FastAPI is a powerful web framework that has gained much attention for its speed, efficiency, and intuitive design. One of its features, background tasks, allows developers to handle time-consuming processes without blocking the main application flow. In this comprehensive user guide, we'll explore everything you need to know to master background tasks with FastAPI.
What Are Background Tasks?
Background tasks are processes that run in the background of your application, freeing up the main thread to handle user requests more efficiently. This can be especially beneficial for tasks such as sending emails, processing large files, or making external API calls. By offloading these tasks, you can enhance the responsiveness and scalability of your application.
Setting Up Your FastAPI Project
Before diving into background tasks, make sure you have FastAPI set up. If not, you can quickly set up a new FastAPI project with:
pip install fastapi
pip install uvicorn[standard]
With the installation complete, create a new FastAPI app:
from fastapi import FastAPI
app = FastAPI()
Implementing Background Tasks
FastAPI's background tasks are easy to implement. Begin by importing the necessary module:
from fastapi import BackgroundTasks
Next, create a background task that you want to run:
def background_task(message: str):
print(f"Processing message: {message}")
Now integrate this task into an endpoint:
@app.post("/send-message")
def send_message(message: str, background_tasks: BackgroundTasks):
background_tasks.add_task(background_task, message)
return {"message": "Message processing in background"}
When a client sends a request to /send-message
, the background task will run without preventing the endpoint from returning a response immediately.
Real-World Use Cases
The true power of background tasks shines in real-world applications. Here are a few examples:
Sending Emails
Handling email sending in the background can vastly improve user experience. Integrate an email library like smtplib
with FastAPI's background tasks:
import smtplib
from email.mime.text import MIMEText
async def send_email_task(recipient: str, subject: str, body: str):
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = "your_email@example.com"
msg['To'] = recipient
with smtplib.SMTP('your_smtp_server.example.com') as server:
server.login("your_username", "your_password")
server.sendmail("your_email@example.com", [recipient], msg.as_string())
Then, use this task in an endpoint:
@app.post("/send-email")
def send_email(recipient: str, subject: str, body: str, background_tasks: BackgroundTasks):
background_tasks.add_task(send_email_task, recipient, subject, body)
return {"message": "Email is being sent"}
Data Processing
For tasks like data processing, where you might need to process files or images, background tasks can be particularly useful:
def process_data_task(data_id: int):
data = get_data_by_id(data_id)
processed_data = perform_some_processing(data)
store_processed_data(data_id, processed_data)
This can be called in an endpoint as follows:
@app.post("/process-data/{data_id}")
def process_data(data_id: int, background_tasks: BackgroundTasks):
background_tasks.add_task(process_data_task, data_id)
return {"message": "Data processing in the background"}
Practical Tips for Effective Background Tasks
Here are some practical tips for using background tasks in FastAPI effectively:
- Resource Management: Be mindful of resource usage and avoid overloading your server with too many concurrent background tasks.
- Error Handling: Implement robust error handling within your background tasks to avoid silent failures.
- Logging: Log important activities within your background tasks for easier debugging and monitoring.
- Async Tasks: Utilize asynchronous functions for I/O-bound operations to maximize efficiency.
Conclusion
Background tasks in FastAPI allow you to handle lengthy operations seamlessly, improving the performance and user experience of your application. By following the guidelines and examples provided in this guide, you can effectively integrate background tasks into your FastAPI projects. Start leveraging the power of background tasks today to build more responsive and scalable applications.
Happy coding!
Recent Posts
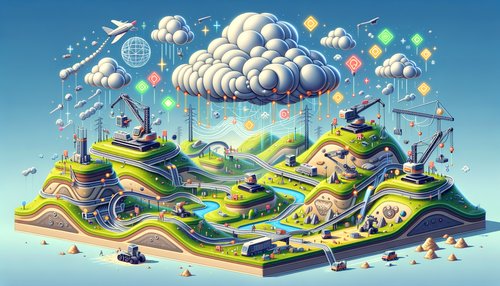
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
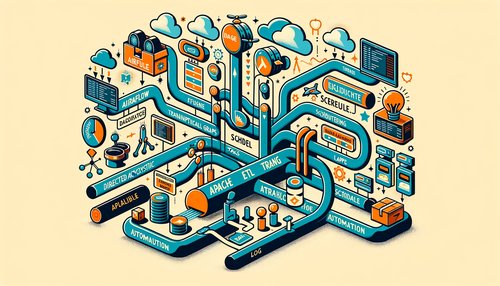
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow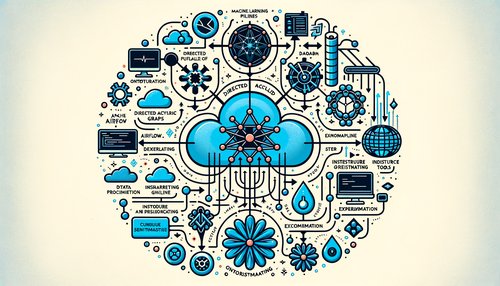
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow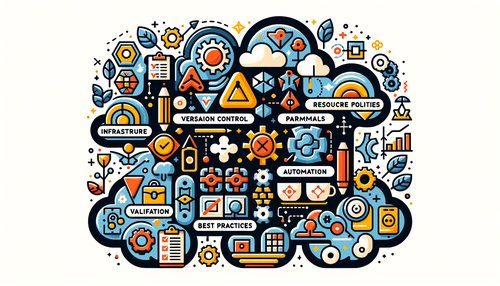
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
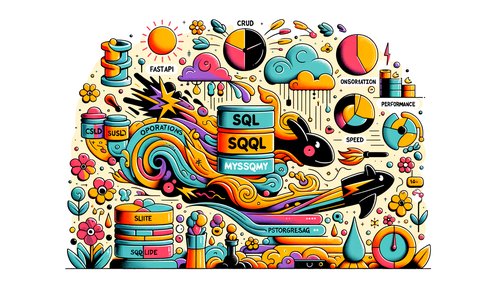