Mastering Data: Dive into Pandas with Our Ultimate Guide to Split-Apply-Combine Techniques
Welcome to our comprehensive guide on harnessing the power of the Split-Apply-Combine strategy using Pandas, the cornerstone library for data manipulation in Python. This guide is designed to empower you with the knowledge and skills to efficiently process and analyze large datasets. Whether you're a data science enthusiast, a budding analyst, or a seasoned professional looking to refine your proficiency, you're in the right place. We'll explore the intricacies of the split-apply-combine paradigm, provide practical examples, and share tips to enhance your data manipulation capabilities. Let's embark on this journey to mastering data with Pandas.
Understanding Split-Apply-Combine
The Split-Apply-Combine strategy is a powerful method for data analysis that involves three steps: splitting the data into groups based on certain criteria, applying a function to each group independently, and then combining the results into a data structure. This approach is particularly useful for aggregating or summarizing data, performing group-wise transformations, and many other operations. We'll delve into how Pandas implements this strategy through its groupby
method and explore the flexibility it offers for advanced data manipulation.
Splitting Data with GroupBy
The first step in the Split-Apply-Combine technique is to split the data. In Pandas, this is achieved using the groupby
method, which segregates data into groups based on one or more keys. This method is incredibly versatile, allowing for simple to complex grouping operations. We'll guide you through creating straightforward single-key groupings to more sophisticated multi-key groupings and demonstrate how to leverage indices for efficient data splitting.
Practical Example: Grouping by a Single Column
Let's start with a basic example. Imagine you have a dataset containing sales data for various stores, and you're interested in calculating the total sales for each store. Here's how you can achieve this with Pandas:
import pandas as pd
# Sample sales data
data = {'Store': ['A', 'B', 'A', 'B', 'A', 'B'],
'Sales': [100, 200, 150, 250, 300, 350]}
df = pd.DataFrame(data)
# Grouping by the "Store" column
grouped = df.groupby('Store')
# Summing up the sales within each group
total_sales = grouped.sum()
print(total_sales)
This simple example illustrates the essence of the splitting step—segregating the data into distinct groups based on the store's name, which allows for individual operations within each group.
Applying Functions to Groups
After splitting the data, the next step is to apply a function to each group. Pandas offers a wide array of built-in methods for common operations such as sum, mean, and median, but it doesn't stop there. You can also apply custom functions to groups, providing a high degree of flexibility. We'll explore how to use both built-in and custom functions to perform group-wise data manipulation and analysis.
Practical Example: Custom Function Application
To illustrate applying custom functions, let's extend our previous example by calculating the average sales for each store, but only for sales above a certain threshold. Here's how you can do it:
def average_sales_above_threshold(group, threshold=200):
filtered_group = group[group['Sales'] > threshold]
return filtered_group['Sales'].mean()
# Applying the custom function to each group
avg_sales = grouped.apply(average_sales_above_threshold, threshold=200)
print(avg_sales)
This example showcases the flexibility of applying functions to groups, allowing for sophisticated analyses tailored to specific requirements. p>
Combining the Results
The final step in the Split-Apply-Combine technique is to combine the results of the function applications into a new data structure. Pandas handles this step automatically in many cases, neatly organizing the results of group-wise operations into a DataFrame or Series, depending on the operation. This seamless integration of results allows for intuitive further analysis and visualization. We'll discuss how Pandas facilitates the combination of results and how you can customize the output format for your needs.
Summary and Final Thoughts
In this guide, we've explored the Split-Apply-Combine technique in Pandas, a powerful strategy for data analysis. We began by understanding the concept and its significance, followed by a deep dive into each of the three steps: splitting data with groupby
, applying functions to groups, and combining the results. Through practical examples, we demonstrated how to perform group-wise analyses and manipulate data efficiently.
Mastering the Split-Apply-Combine technique can significantly enhance your data analysis capabilities, allowing you to tackle complex tasks with ease. As you continue to explore data manipulation with Pandas, remember that practice is key to proficiency. We encourage you to experiment with different datasets, explore various grouping keys, and apply diverse functions to groups to deepen your understanding and skills.
Happy data wrangling!
Recent Posts
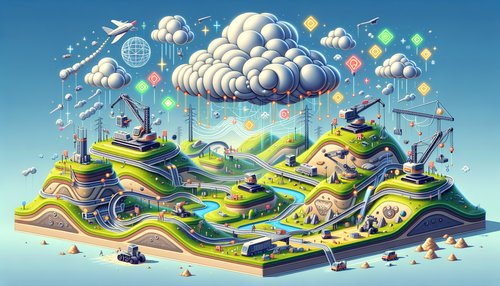
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
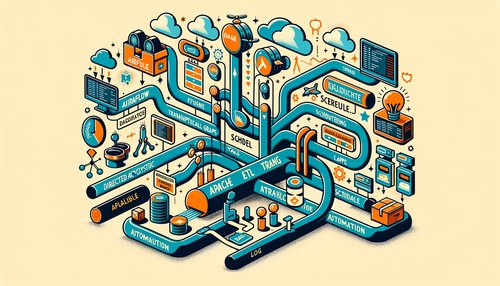
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow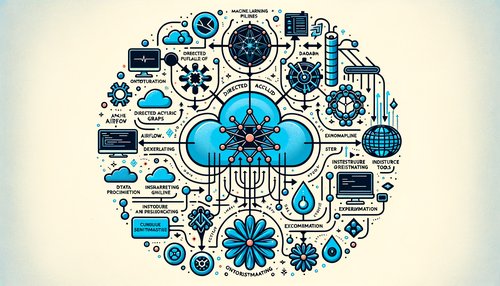
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow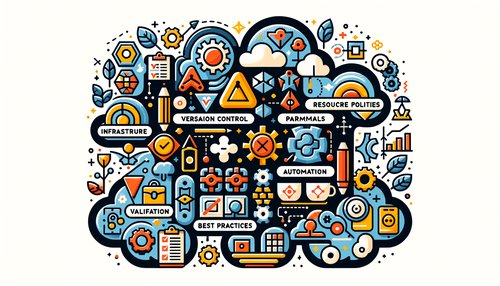
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
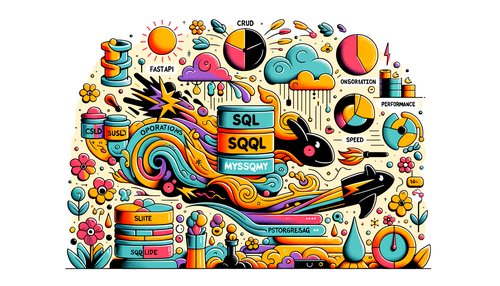