Mastering Data Manipulation: How to Merge, Join, Concatenate, and Compare like a Pro with the Ultimate Pandas User Guide
Welcome to your go-to guide on mastering data manipulation using Pandas, the powerhouse Python library that has transformed data analysis and manipulation. Whether you're a beginner eager to dive into the world of data science or a seasoned analyst looking to refine your skills, this guide is designed to arm you with the techniques to merge, join, concatenate, and compare datasets with confidence and precision. Get ready to unlock the full potential of your data with the ultimate Pandas user guide.
Understanding Pandas Data Structures
Before diving into data manipulation techniques, it's crucial to understand the core data structures in Pandas: Series and DataFrame. A Series is a one-dimensional array capable of holding any data type, while a DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). Grasping these concepts is the first step towards mastering Pandas.
Merging DataFrames
Merging is a powerful tool for combining datasets based on common columns or indices. Think of it as joining tables in a database. Pandas provides the merge()
function, which offers SQL-like capabilities right within Python. The key parameters to understand are how
(type of merge to perform), on
(column or index names to join on), and indicator
(adds a column to the output DataFrame showing the source of each row).
import pandas as pd
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'],
'value': range(4)})
df2 = pd.DataFrame({'key': ['B', 'D', 'D', 'E'],
'value': range(4)})
merged_df = pd.merge(df1, df2, on='key', how='inner')
print(merged_df)
This example shows an inner join between two DataFrames, combining rows with matching keys.
Joining DataFrames
Joining is similar to merging but focuses on combining DataFrames based on their indexes. Using the join()
function, you can concatenate DataFrames horizontally. This is especially useful when you have related datasets with different information for the same observations.
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']},
index=['K0', 'K1', 'K2', 'K3'])
df2 = pd.DataFrame({'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']},
index=['K0', 'K1', 'K2', 'K3'])
joined_df = df1.join(df2)
print(joined_df)
This operation defaults to a left join, keeping all rows from the left DataFrame and adding columns from the right DataFrame.
Concatenating DataFrames
Concatenation is the process of appending one sequence of data to another. With Pandas' concat()
function, you can combine Series or DataFrame objects horizontally or vertically, allowing for a variety of flexible data manipulations.
pd.concat([df1, df2], axis=1)
This snippet demonstrates horizontal concatenation, combining DataFrames side by side.
Comparing DataFrames
Comparing DataFrames is a common task when dealing with multiple data sources. You might need to identify differences in data loaded at different times or compare datasets for consistency. Pandas offers the compare()
function to simplify this process, highlighting differences between DataFrames.
diff = df1.compare(df2)
print(diff)
This function returns a new DataFrame showing the changes from the first DataFrame to the second, making it easier to spot discrepancies.
Summary
In this comprehensive guide, we've covered the essential techniques of merging, joining, concatenating, and comparing DataFrames using Pandas. These operations are crucial for data manipulation and analysis, allowing you to clean, prepare, and understand your data more deeply. By mastering these techniques, you'll be well-equipped to tackle complex data challenges and extract meaningful insights from vast datasets.
As you continue your data manipulation journey, remember that practice is key to becoming proficient. Experiment with different datasets, explore the nuances of each operation, and leverage the full power of Pandas to transform raw data into actionable knowledge.
Happy data wrangling!
Recent Posts
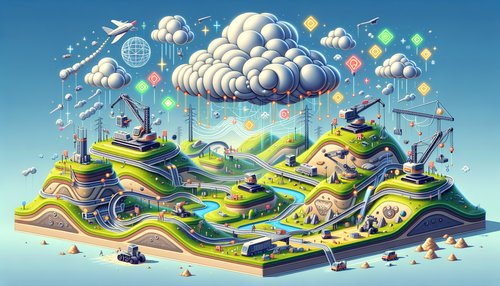
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
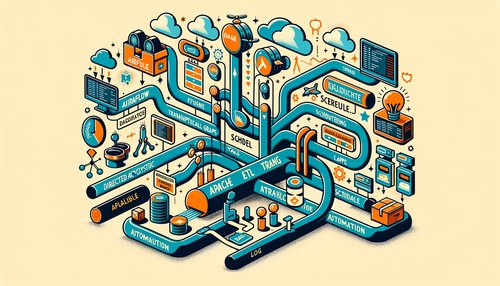
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow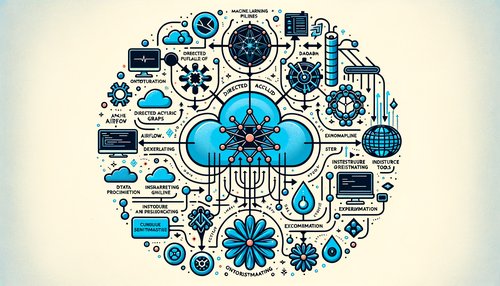
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow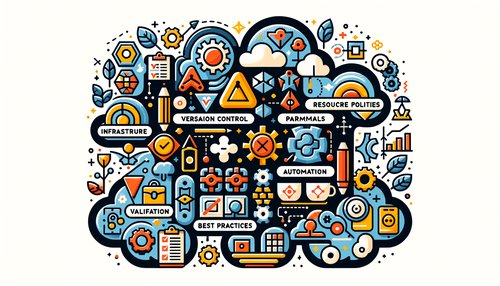
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
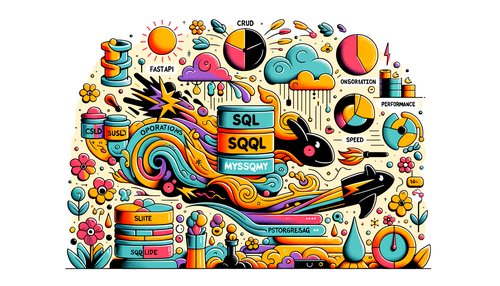