Mastering FastAPI: A Comprehensive Guide to Building Bigger Applications with Multiple Files
In today's fast-paced digital world, agility and efficiency in building web applications are crucial. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints, is a powerful tool that caters to these needs. But when projects grow beyond a single script, how do you manage and structure your FastAPI applications effectively? This guide will walk you through the journey of splitting your FastAPI app into multiple files, making your code modular, maintainable, and ready for expansion.
Why Structure Your FastAPI Application?
As your FastAPI application grows, a single-file setup can become cumbersome. Much like how you wouldn't write an entire book in a single chapter, laying out your application in different modules or files helps with:
- Readability: Smaller, single-responsibility files are easier to read and navigate.
- Maintainability: Bugs and requirements changes can be managed without diving into an unwieldy monolithic script.
- Scalability: Modular code is easier to test and extend.
Organizing Your Project Structure
A well-organized project structure is the backbone of any scalable application. Here is a common structure you might consider for a FastAPI project:
.
├── app
│ ├── main.py
│ ├── routes
│ │ ├── __init__.py
│ │ └── user.py
│ ├── models
│ │ ├── __init__.py
│ │ └── user.py
│ ├── schemas
│ │ ├── __init__.py
│ │ └── user.py
│ └── database.py
└── requirements.txt
Each directory contains files dedicated to specific responsibilities, like defining routes, models, and schemas.
Building Your App
Let's go into details on how to split each component into different files:
Main Application File
Your primary entry point will be main.py
. This is where the FastAPI instance is created and includes all the routing:
from fastapi import FastAPI
from app.routes import user
app = FastAPI()
app.include_router(user.router)
Defining Routes in a Separate File
Start by creating a user.py
file within the routes
directory, where you'll define your API routes:
from fastapi import APIRouter
router = APIRouter()
@router.get("/users")
async def get_users():
return [{"username": "user1"}, {"username": "user2"}]
Models and Schemas
It's essential to have clear models and schemas, particularly if you're dealing with databases. In models/user.py
, define your ORM models, and you're likely to use Pydantic models in schemas/user.py
:
# models/user.py
from sqlalchemy import Column, Integer, String
from app.database import Base
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True, index=True)
username = Column(String, index=True)
# schemas/user.py
from pydantic import BaseModel
class User(BaseModel):
id: int
username: str
class Config:
orm_mode = True
Connecting to the Database
For a robust application, you will need to integrate a database connection. This is typically handled in database.py
:
from sqlalchemy import create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
SQLALCHEMY_DATABASE_URL = "sqlite:///./test.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL, connect_args={"check_same_thread": False})
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
Practical Tips for FastAPI Development
As you develop with FastAPI, consider these practical tips:
- Use environment variables: Keep sensitive data out of your code by using environment files (e.g.,
.env
). - Documentation: FastAPI automatically generates interactive documentation which is a great way to keep your API consumer in the loop.
- Testing: FastAPI provides great testing support using the
TestClient
, ensuring you can write endpoints tests with ease.
Conclusion
Structuring your FastAPI application into multiple files is more than just a best practice—it's essential for building maintainable, scalable, and high-quality applications. By following the right approach to project organization, defining routes, models, and other components in separate modules, you prepare your application for future growth and complexity. Start restructuring your FastAPI project today, and experience the benefits of clear, organized, and systematic applications.
Take action by revisiting your existing FastAPI projects and consider applying these principles. Happy coding!
Recent Posts
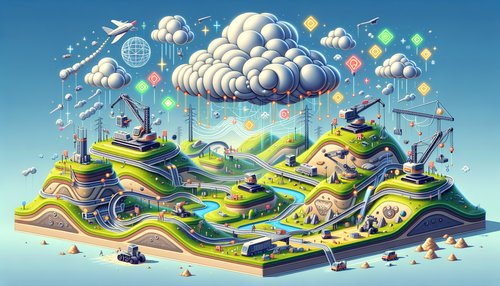
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
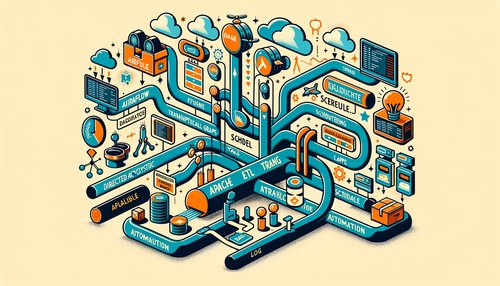
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow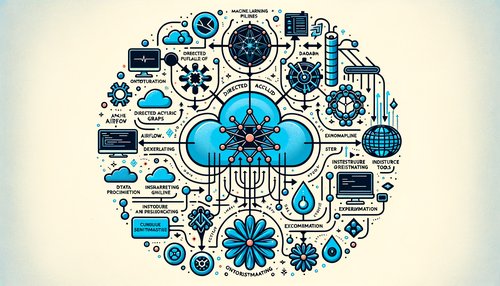
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow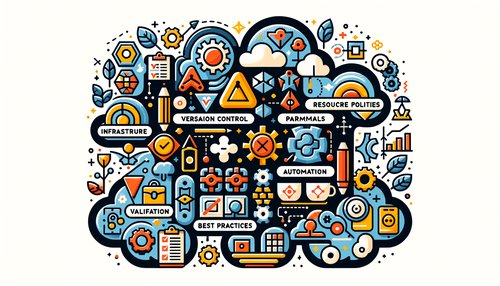
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
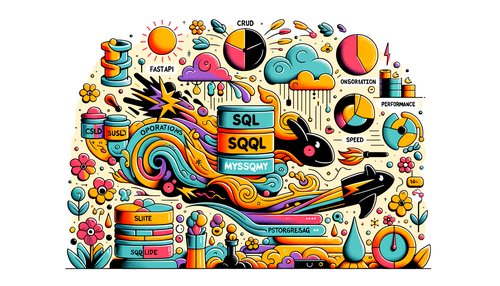