Mastering FastAPI: A Comprehensive Guide to Harnessing Header Parameters Like a Pro!
Welcome to the definitive guide on leveraging header parameters in FastAPI, an essential skill set for any aspiring or seasoned developer looking to create robust and efficient web applications. FastAPI, known for its high performance and ease of use, provides an excellent framework for building APIs with Python 3.7+. This guide will delve into the intricacies of using header parameters within FastAPI, ensuring you can utilize these features to their full potential. From authentication to custom client data handling, mastering header parameters will elevate your API development skills.
Understanding Header Parameters in FastAPI
Before diving into the practical aspects, it's crucial to grasp what header parameters are and why they're vital in API development. Header parameters are part of the HTTP request sent from the client to the server, containing metadata about the request or the client itself. In FastAPI, these parameters can be used for various purposes, including but not limited to, content negotiation, authentication, and controlling cache policies.
Declaring Header Parameters
FastAPI simplifies the declaration of header parameters using Python type annotations. Here's a basic example:
from fastapi import FastAPI, Header
app = FastAPI()
@app.get("/items/")
async def read_items(user_agent: str = Header(None)):
return {"User-Agent": user_agent}
This snippet demonstrates how to retrieve the User-Agent
header from the client's request. By setting the default value to None
, the header becomes optional.
Best Practices for Using Header Parameters
While FastAPI provides the flexibility to use header parameters as needed, adhering to certain best practices can enhance the security, performance, and maintainability of your API.
Security Considerations
Always sanitize header values to prevent injection attacks. Although FastAPI automatically handles many security concerns, being vigilant about input validation is a good practice. For sensitive data, such as authentication tokens, ensure they are transmitted over secure channels (HTTPS).
Performance Implications
Minimize reliance on header parameters for critical operations. While they are useful, excessive use can lead to bloated request headers, impacting the overall performance of your API.
Advanced Usage of Header Parameters
FastAPI's flexibility allows for more sophisticated use cases, including:
- Custom Headers: Creating custom headers for specific functionalities can be particularly useful for versioning, localization, or custom authentication schemes.
- Dependency Injection: FastAPI supports dependency injection, allowing you to create reusable components that depend on header values. This feature can simplify the development of complex applications.
Example: Custom Authentication Header
from fastapi import FastAPI, Header, HTTPException
app = FastAPI()
def verify_token(x_token: str = Header(...)):
if x_token != "secret-token":
raise HTTPException(status_code=400, detail="Invalid X-Token header")
return x_token
@app.get("/secure-endpoint/")
async def secure_endpoint(token: str = Depends(verify_token)):
return {"message": "Welcome to the secure endpoint!"}
This example showcases a simple way to implement custom authentication using header parameters and dependency injection.
Conclusion
Mastering header parameters in FastAPI is a journey that can significantly improve the quality and security of your API projects. By understanding how to efficiently declare, utilize, and best practice these parameters, you'll be well-equipped to take your API development skills to the next level. Remember, the key to mastering FastAPI lies in experimentation and continuous learning, so don't hesitate to explore more advanced features as you grow.
Embrace the power of FastAPI and its handling of header parameters to create more dynamic, secure, and efficient web applications. Happy coding!
Recent Posts
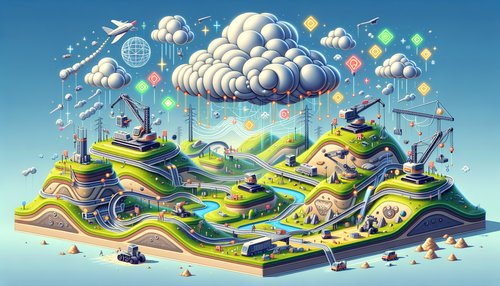
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
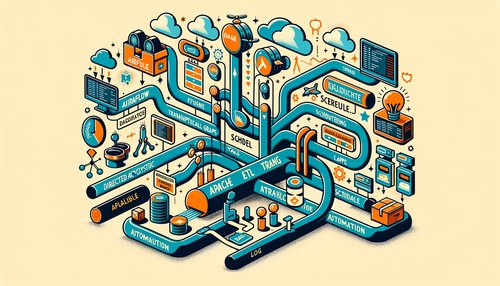
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow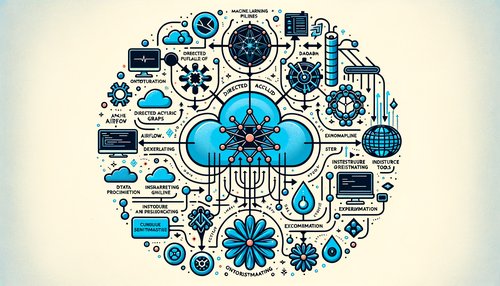
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow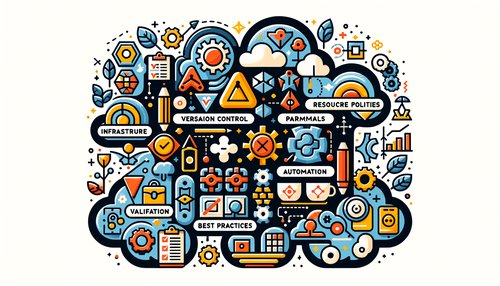
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
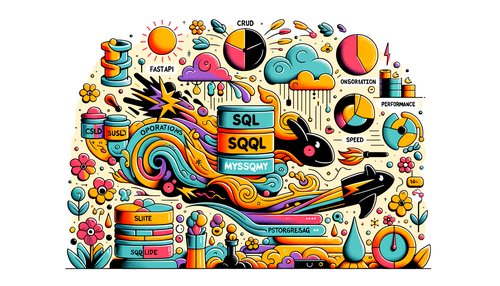