Mastering FastAPI: A Comprehensive Guide to Serving Static Files Efficiently
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. While FastAPI is known for its power in handling API endpoints, serving static files efficiently is also an important aspect of many web applications. This comprehensive guide will help you master the art of serving static files using FastAPI.
Introduction to Serving Static Files in FastAPI
Static files, such as HTML, CSS, JavaScript, and images, are crucial components of web applications. FastAPI provides several ways to serve these files efficiently. In this guide, we will cover:
- Setting up a basic FastAPI application.
- Configuring static file serving.
- Efficiently organizing and caching static files.
- Handling common issues and best practices.
Setting Up a Basic FastAPI Application
Before jumping into serving static files, let's set up a basic FastAPI application. If you haven't already installed FastAPI, you can do so using pip:
pip install fastapi uvicorn
Next, create a Python file named main.py
and write the following code:
from fastapi import FastAPI
import uvicorn
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
if __name__ == "__main__":
uvicorn.run(app, host="127.0.0.1", port=8000)
Run this application using the command:
uvicorn main:app --reload
Configuring Static File Serving
FastAPI makes it straightforward to serve static files. Use the StaticFiles
class from starlette.staticfiles
. Add the following to your main.py
file:
from fastapi.staticfiles import StaticFiles
app.mount("/static", StaticFiles(directory="static"), name="static")
Here, we're telling FastAPI to serve files from the ./static
directory under the /static
URL path. Create a directory named static
and add some files (e.g., style.css
) to test.
You can now access these files at http://127.0.0.1:8000/static/style.css
.
Efficiently Organizing and Caching Static Files
To keep your project organized, consider creating a nested directory structure within the static
folder for different types of static files, such as:
static/css
static/js
static/images
Additionally, enabling caching headers can dramatically improve the performance of serving static files by allowing the browser to cache files. You can use a middleware to set caching headers:
from starlette.middleware.base import BaseHTTPMiddleware, RequestResponseEndpoint
from starlette.requests import Request
from starlette.responses import Response
class CacheControlMiddleware(BaseHTTPMiddleware):
async def dispatch(self, request: Request, call_next: RequestResponseEndpoint) -> Response:
response = await call_next(request)
response.headers["Cache-Control"] = "public, max-age=3600"
return response
app.add_middleware(CacheControlMiddleware)
Handling Common Issues and Best Practices
Serving static files can come with a few common issues, such as:
- File Not Found Errors: Ensure that the file paths are correct and your static directory is correctly referenced.
- Caching Problems: Sometimes browsers aggressively cache files. Clear the cache or use versioning in filenames (e.g.,
style.v1.css
).
Some best practices include:
- Minify CSS and JavaScript files to reduce load times.
- Optimize images for the web to save bandwidth.
- Keep your static directory structure organized for maintainability.
Conclusion
Serving static files efficiently is a crucial aspect of web development. In this guide, we covered setting up a basic FastAPI application, configuring static file serving, organizing and caching static files, and handling common issues. By following these practices, you will ensure a smooth and efficient user experience for your web applications.
Implement these steps in your FastAPI projects and take your application's performance to the next level. Happy coding!
Recent Posts
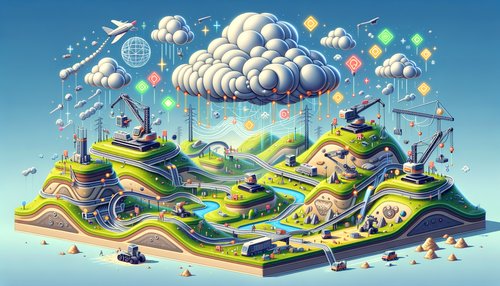
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
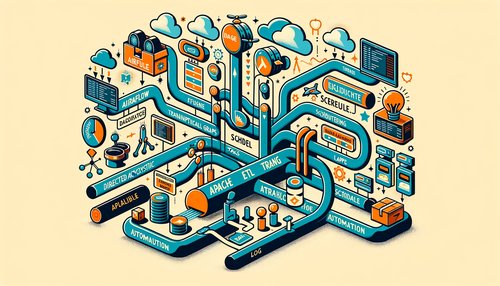
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow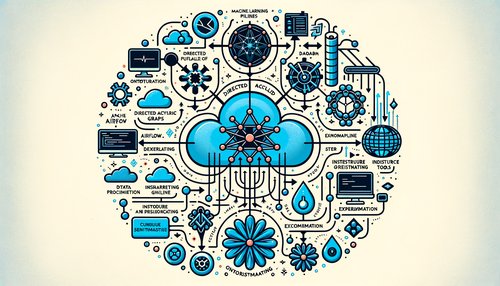
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow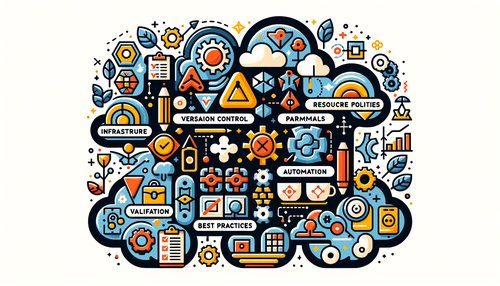
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
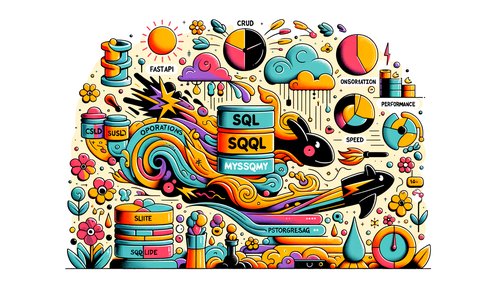