Mastering FastAPI: A Comprehensive User Guide to Efficient Debugging
FastAPI has quickly gained popularity among developers for its speed, simplicity, and flexibility in building APIs. But even the most robust applications are not immune to bugs and errors. Efficient debugging is a crucial skill that can save developers countless hours and frustration. In this guide, we’ll explore strategies to master debugging in FastAPI, ensuring your applications run smoothly and efficiently.
Understanding Common FastAPI Errors
Before you can solve a problem, you need to understand it. FastAPI introduces specific errors that might not always be intuitive, especially for those coming from different frameworks. Here are some common FastAPI errors and how to address them:
- Validation Errors: FastAPI automatically validates request data against your Pydantic models. These errors are often straightforward but crucial to handle. Use detailed error messages to quickly identify mismatches between your model and incoming data.
- Dependency Injection Errors: FastAPI’s dependency injection is powerful but can lead to errors if dependencies aren't configured correctly. Make sure all required dependencies are passed correctly.
- Routing Issues: Misconfigured paths or HTTP methods can lead to 404 errors. Double-check your path definitions and remember that order matters!
Setting Up Your Development Environment
An effective development environment is key to efficient debugging. Here are some setup tips:
- Use an IDE with FastAPI Support: Visual Studio Code with Python extensions or PyCharm can greatly enhance your debugging process with features like breakpoints and integrated terminals.
- Enable Autoreload: Use
uvicorn main:app --reload
during development to automatically reload your application after code changes, which saves time. - Logging Configuration: Properly configured logging provides detailed insight into application flow and errors, helping track down issues quickly.
Leveraging Tools and Extensions
FastAPI, coupled with a rich ecosystem of tools, makes debugging more manageable. Consider these tools:
- Postman/Insomnia: For testing your endpoints quickly without needing a frontend.
- Swagger UI: FastAPI’s built-in generation of interactive API documentation allows for real-time testing and inspection of API endpoints.
- Pydantic's Validation and Debugging Tools: Pydantic models not only validate data but also provide utilities for debugging, such as the
dict()
method, for inspecting model fields.
Advanced Debugging Techniques
Once you master the basics, here are some advanced techniques to consider:
- Profiling: Use Python’s
cProfile
or similar tools to pinpoint performance bottlenecks in your application. - Tracing: Libraries like OpenTelemetry can trace requests across microservices, providing a holistic view of your application’s execution path.
- Interactive Debugging: Integrating a debugger to stop execution at any frame of the stack offers real-time inspection of variable states.
Conclusion
Debugging is an art and a science, requiring patience and practice. By understanding common pitfalls, setting up a conducive development environment, utilizing key tools, and employing advanced techniques, you’ll be well-equipped to tackle any issue FastAPI throws your way. Remember, the key to efficient debugging is not just finding and fixing errors but enhancing the overall robustness and performance of your application. So, explore these tools and tips, and take your FastAPI debugging skills to the next level!
Ready to put these tips into practice? Start evaluating your current FastAPI projects, and apply these strategies to ensure a seamless, error-free development experience!
Recent Posts
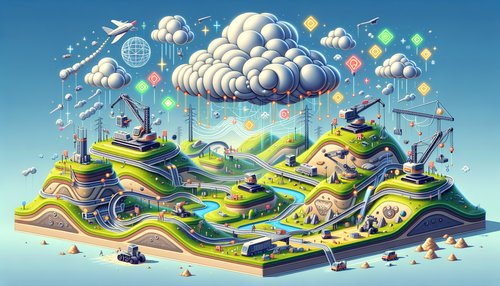
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
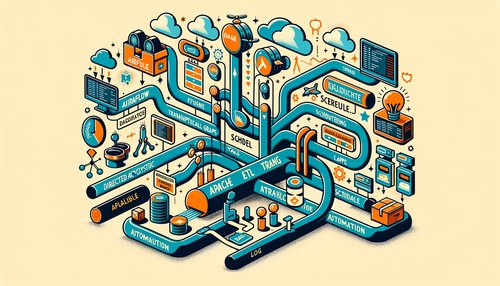
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow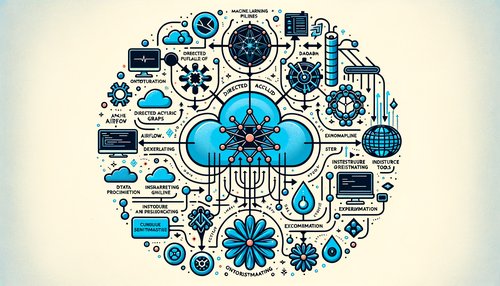
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow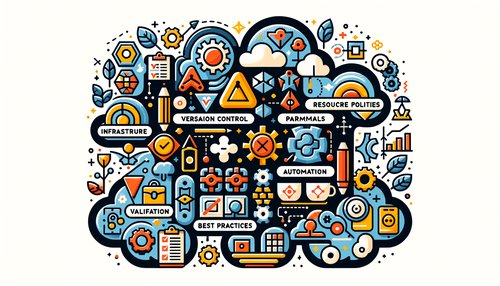
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
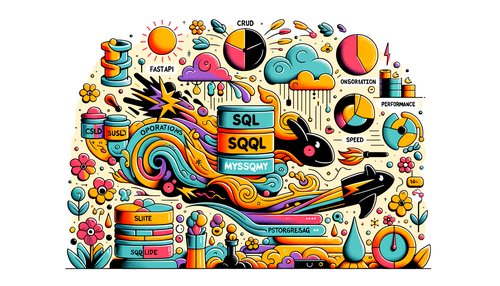