Mastering FastAPI: A Comprehensive User Guide to Efficiently Managing Background Tasks
FastAPI has rapidly gained traction among developers for its ability to create robust and high-performance APIs effortlessly. One of its powerful features is the ability to handle background tasks efficiently. In this comprehensive guide, we will explore how to master background tasks in FastAPI, providing you with practical tips, detailed examples, and essential insights to enhance your applications.
Introduction to Background Tasks in FastAPI
Background tasks allow you to offload time-consuming operations from the main request-response cycle, enabling your API to respond more quickly to initial requests. This section will introduce the concept of background tasks in FastAPI, giving you a high-level understanding of how and when to use them.
Setting Up FastAPI for Background Tasks
Before diving into background tasks, it's essential to set up your FastAPI environment correctly. Ensure you have FastAPI and an ASGI server like uvicorn
installed:
pip install fastapi uvicorn
Now, let's create a simple FastAPI application:
from fastapi import FastAPI
app = FastAPI()
Understanding Background Tasks in FastAPI
FastAPI provides a built-in BackgroundTasks
class to handle background execution. Let's explore a basic example of how to use this feature. Consider an API endpoint that sends a welcome email to new users:
from fastapi import BackgroundTasks, FastAPI
app = FastAPI()
def send_welcome_email(email: str):
# Simulate sending email
print(f"Sending welcome email to {email}")
@app.post("/signup")
def signup(email: str, background_tasks: BackgroundTasks):
background_tasks.add_task(send_welcome_email, email)
return {"message": "User signup successful"}
In this example, the signup
endpoint triggers a background task to send an email, allowing the API to respond immediately without waiting for the email process to complete.
Advanced Background Task Management
For more complex scenarios, you might need robust task management. Consider using Celery, a distributed task queue, along with FastAPI. First, install Celery:
pip install celery
Next, configure Celery to work with FastAPI:
from celery import Celery
celery_app = Celery("tasks", broker="redis://localhost:6379/0")
@celery_app.task
def send_welcome_email(email: str):
print(f"Sending welcome email to {email}")
@app.post("/signup")
def signup(email: str):
send_welcome_email.delay(email)
return {"message": "User signup successful"}
In this setup, send_welcome_email
tasks are queued and processed by Celery workers, allowing FastAPI to handle more extensive and distributed task management effectively.
Practical Tips for Efficient Task Management
- Use appropriate task queues: Select a queue system like Redis or RabbitMQ that fits your needs.
- Monitor task performance: Keep an eye on task execution times and manage tasks to prevent bottlenecks.
- Prioritize tasks: Ensure critical tasks are prioritized appropriately to maintain application performance.
Conclusion
Mastering background tasks in FastAPI can significantly enhance the efficiency and performance of your applications. Whether you use FastAPI's built-in BackgroundTasks
class for simple tasks or integrate Celery for more complex requirements, proper task management is crucial. By applying the practical tips and insights provided in this guide, you'll be well on your way to creating responsive and high-performance FastAPI applications.
Ready to take your FastAPI skills to the next level? Start experimenting with background tasks in your projects and experience the difference they can make!
Recent Posts
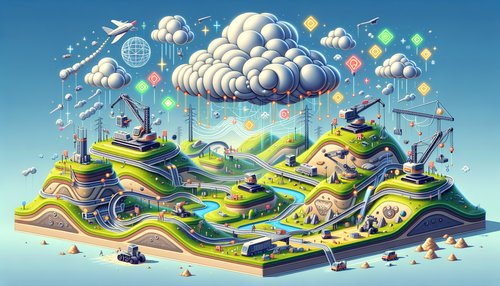
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
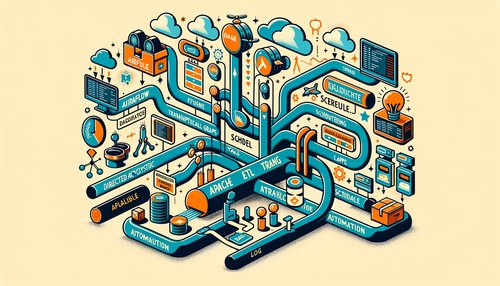
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow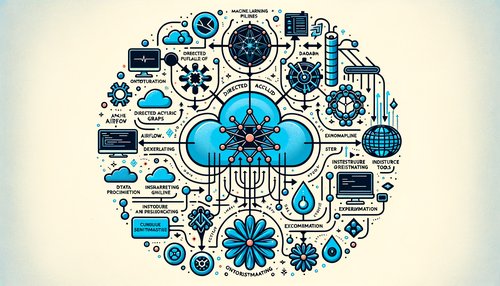
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow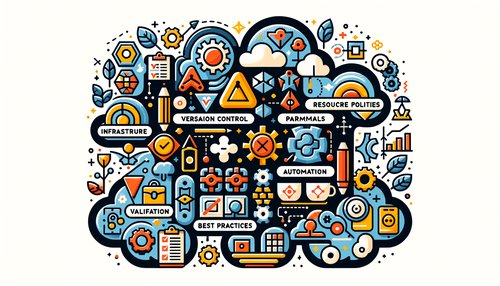
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
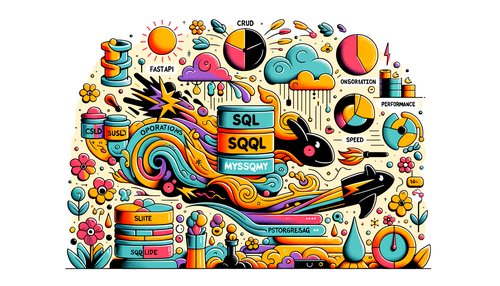