Mastering FastAPI: A Comprehensive User Guide to Gracefully Handling Errors
Welcome to the ultimate guide on how to master error handling in FastAPI, the modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. In this comprehensive user guide, we will dive deep into the art of gracefully handling errors in FastAPI, ensuring your API not only works efficiently but also robustly manages any issues that arise, providing a seamless experience for your users. Whether you're just starting with FastAPI or looking to refine your skills, this guide has something for everyone.
Understanding Error Handling in FastAPI
Before we delve into the specifics, it's crucial to understand what error handling is and why it's important in FastAPI. Error handling refers to the process of catching and managing errors that occur during the execution of a program. In the context of FastAPI, this means dealing with any issues that arise during request processing in a way that doesn't crash your application and provides useful feedback to the client.
Types of Errors in FastAPI
FastAPI can encounter several types of errors, including:
- Validation Errors: Occur when the data sent by the client doesn't match the expected format.
- Authentication Errors: Happen when there are issues validating the user's identity.
- Permission Errors: Arise when a user tries to access resources they don't have access to.
- Internal Server Errors: Occur due to issues within the server itself, such as coding errors or failed external services.
Implementing Basic Error Handling
FastAPI provides built-in support for handling errors gracefully. At the most basic level, you can handle errors in FastAPI using try
and except
blocks within your route functions. This allows you to catch exceptions and return appropriate responses.
from fastapi import FastAPI, HTTPException
app = FastAPI()
@app.get("/items/{item_id}")
async def read_item(item_id: int):
try:
# Attempt to retrieve the item
except Exception as e:
raise HTTPException(status_code=404, detail=f"Item not found: {e}")
Using HTTPException for Standard Errors
For more standardized error handling, FastAPI provides the HTTPException
class. You can use this class to return any HTTP status code and detail message. It's a more structured approach compared to handling exceptions manually.
Custom Exception Handlers
For more control over the error responses, you can create custom exception handlers. This involves using the @app.exception_handler
decorator to register a function as the handler for a specific exception type.
from fastapi import FastAPI, Request
from fastapi.responses import JSONResponse
from custom_exceptions import CustomException
app = FastAPI()
@app.exception_handler(CustomException)
async def custom_exception_handler(request: Request, exc: CustomException):
return JSONResponse(
status_code=418,
content={"message": f"Oops! {exc.name} did something. There goes a teapot."},
)
Logging Errors
Logging is an essential aspect of error handling. It helps in diagnosing issues by providing insights into what went wrong. FastAPI doesn't come with a built-in logging system, but you can easily integrate Python's standard logging module to log errors that occur in your application.
Best Practices for Error Handling in FastAPI
- Be Explicit: Clearly define the types of errors your API can encounter and handle them explicitly.
- Use HTTP Status Codes Appropriately: Make use of HTTP status codes to indicate the nature of the error (client-side or server-side).
- Provide Useful Error Messages: Always return error messages that can help the client understand what went wrong and how to fix it.
- Log Errors: Implement logging to keep track of errors, which can be invaluable for debugging purposes.
Conclusion
Mastering error handling in FastAPI is crucial for building robust and user-friendly APIs. By understanding the types of errors that can occur and leveraging FastAPI's built-in features and best practices for handling these errors, you can ensure that your application gracefully manages any issues, providing a better experience for your users. Remember, the goal of error handling is not just to prevent crashes but to make your API more reliable and easier to use. With the insights and examples provided in this guide, you're well on your way to mastering error handling in FastAPI.
As a final thought, always keep in mind that error handling is an ongoing process. As your API evolves, new types of errors might emerge. Therefore, continuously refine your error handling strategies to cover new scenarios, and don't forget to log errors and monitor your API's health. Happy coding!
Recent Posts
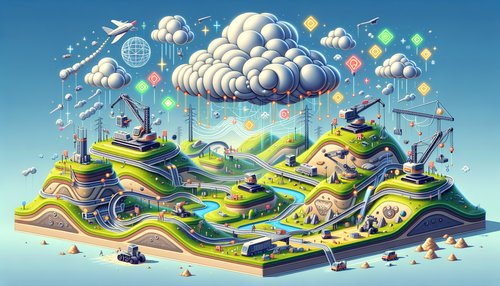
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
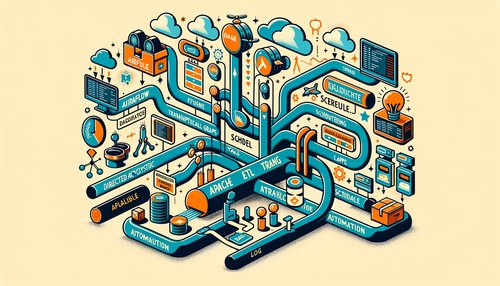
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow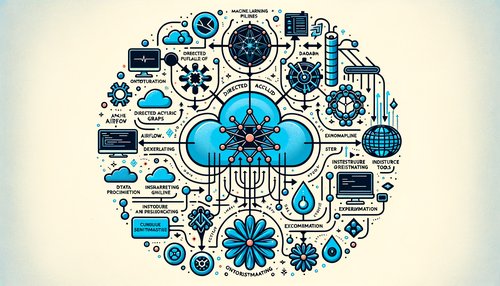
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow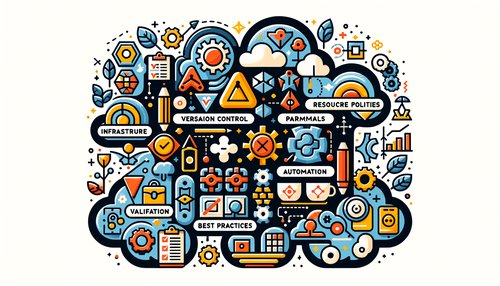
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
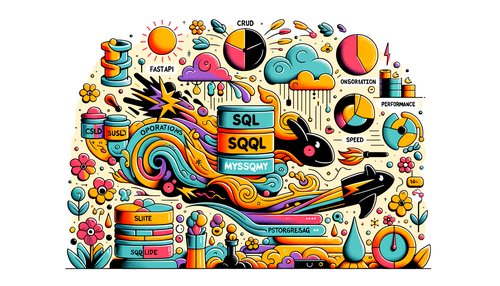