Mastering FastAPI: A User's Ultimate Guide to Leveraging Yield in Dependency Management
Welcome to the definitive guide on harnessing the power of yield
in managing dependencies with FastAPI. This cutting-edge framework has transformed the way we build high-performance, scalable web applications. But to truly unlock its potential, understanding the nuances of dependency management is key. In this comprehensive post, we'll explore the role of yield
in FastAPI's dependency injection system, offering practical tips, real-world examples, and insights to elevate your development skills. Whether you're a seasoned developer or just starting out, this guide will equip you with the knowledge to master FastAPI's dependency management like a pro.
Understanding Dependency Injection in FastAPI
Before diving into the specifics of yield
, let's first understand the concept of dependency injection in FastAPI. Dependency injection is a design pattern that FastAPI utilizes to manage resources and share functionality across your application. It allows you to define components (dependencies) that can be reused throughout your application, promoting cleaner, more modular code.
In FastAPI, dependencies can perform a range of tasks, from database connections and CRUD operations to authentication and authorization. The framework's powerful dependency injection system is one of its standout features, enabling developers to build robust, efficient web services.
Leveraging yield
in Dependency Management
The yield
statement in Python plays a pivotal role in FastAPI's dependency management, particularly when it comes to creating and tearing down resources. When a dependency function includes a yield
statement, FastAPI knows to execute the code before the yield
as setup code and the code after the yield
as teardown code.
This mechanism is perfect for managing resources that need to be initialized and disposed of with each request, such as database connections. By using yield
, you ensure that resources are cleanly allocated and released, avoiding common issues like memory leaks and connection overloads.
Example: Managing Database Connections
from fastapi import FastAPI, Depends
from sqlalchemy.orm import Session
from . import models
from .database import SessionLocal, engine
models.Base.metadata.create_all(bind=engine)
app = FastAPI()
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
@app.get("/items/")
def read_items(db: Session = Depends(get_db)):
return db.query(models.Item).all()
This example demonstrates how to use yield
to manage a database session in FastAPI. The get_db
dependency creates a new database session with each request and closes it after the request is processed.
Best Practices for Using yield
in FastAPI
To make the most out of yield
in dependency management, consider the following best practices:
- Resource Cleanup: Always ensure that resources allocated before
yield
are properly cleaned up or released afteryield
. This is crucial for avoiding resource leaks. - Error Handling: Implement error handling mechanisms around the
yield
statement to catch and handle exceptions, ensuring that resources are released even when errors occur. - Testing: When writing tests for your FastAPI applications, mock dependencies that use
yield
to ensure that your tests are not dependent on external resources.
Conclusion
FastAPI's dependency injection system, powered by the yield
statement, offers a robust solution for managing resources efficiently and effectively. By understanding and leveraging yield
in your dependencies, you can build more reliable, maintainable, and scalable web applications. Remember to follow best practices for resource management and error handling to ensure your FastAPI projects run smoothly. Now, equipped with this knowledge, you're ready to take your FastAPI development to the next level. Happy coding!
As we wrap up, consider experimenting with yield
in your next FastAPI project to see firsthand the benefits it brings to dependency management. And as always, keep exploring and pushing the boundaries of what's possible with FastAPI.
Recent Posts
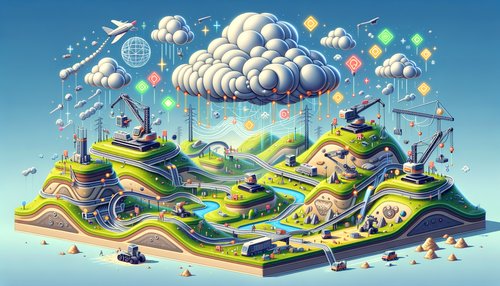
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
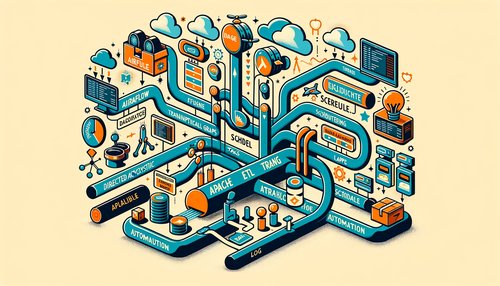
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow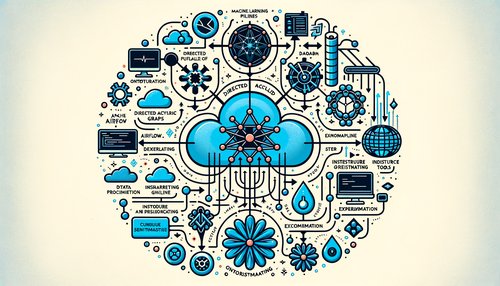
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow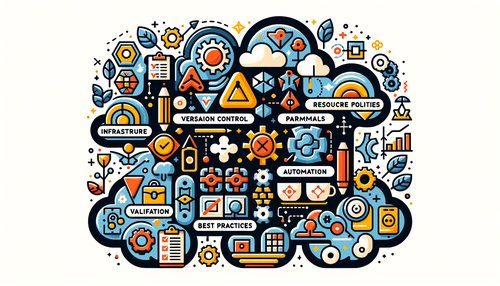
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
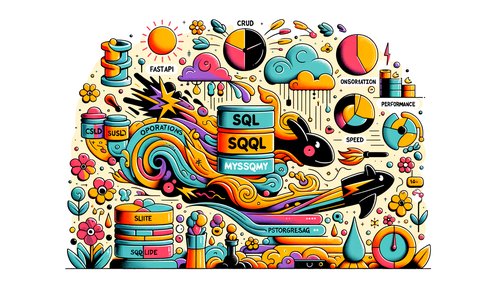