Mastering FastAPI: Navigating the Depths of User Guide for Dependencies and Sub-dependencies
Welcome to the thrilling world of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. The speed of FastAPI, coupled with its ease of use, makes it an attractive choice for developers looking to deploy scalable and efficient web applications. In this comprehensive blog post, we'll dive deep into the intricacies of managing dependencies and sub-dependencies within FastAPI, an aspect that is pivotal for creating robust and maintainable applications. From understanding the basics to exploring advanced techniques, we'll cover everything you need to know to master dependencies in FastAPI. So, buckle up and prepare to unleash the full potential of your web applications with FastAPI!
Understanding Dependencies in FastAPI
Before we delve into the complexities of managing dependencies, let's first understand what dependencies are in the context of FastAPI. Dependencies are a powerful feature in FastAPI that allow you to share logic between different parts of your application, such as database connections, authentication, and authorization routines. Essentially, dependencies are functions that get executed before your endpoint function and can return data to it. This modular approach ensures that your codebase is DRY (Don't Repeat Yourself) and easy to maintain.
Creating Your First Dependency
Creating a dependency in FastAPI is straightforward. Here's a simple example to get you started:
from fastapi import Depends, FastAPI
app = FastAPI()
def get_query(token: str):
return {"token": token}
@app.get("/items/")
async def read_items(token: str = Depends(get_query)):
return {"token": token}
This example demonstrates how to create a basic dependency (`get_query`) that extracts a token from the query parameters and how to use it in an endpoint (`read_items`).
Advanced Dependency Management
As your FastAPI applications grow in complexity, you'll likely encounter scenarios where you need to manage more intricate dependency chains or share dependencies across multiple endpoints.
Sub-dependencies
FastAPI supports sub-dependencies, allowing dependencies to have their own dependencies. This feature is incredibly useful for creating reusable components that can be composed in various configurations. Here's an example to illustrate:
from fastapi import Depends, FastAPI
app = FastAPI()
def query_extractor(q: str = None):
return q
def query_modifier(q: str = Depends(query_extractor)):
return f"{q} modified" if q else "No query"
@app.get("/items/")
async def read_items(modified_query: str = Depends(query_modifier)):
return {"modified_query": modified_query}
In this example, `query_modifier` is a sub-dependency of the `read_items` endpoint, and it itself depends on `query_extractor`. This setup allows for modular and maintainable code.
Using Dependency Classes
For more complex dependency scenarios, FastAPI allows you to define dependencies using classes. This approach offers greater flexibility and the ability to use dependency injection patterns more extensively. Here's a quick look at how you can define a class-based dependency:
from fastapi import Depends, FastAPI
app = FastAPI()
class CommonQueryParams:
def __init__(self, q: str = None, skip: int = 0, limit: int = 100):
self.q = q
self.skip = skip
self.limit = limit
@app.get("/items/")
async def read_items(commons: CommonQueryParams = Depends()):
response = {"skip": commons.skip, "limit": commons.limit}
if commons.q:
response.update({"q": commons.q})
return response
This example demonstrates how to encapsulate common query parameters within a class and use an instance of this class as a dependency.
Practical Tips for Dependency Management
- Reuse dependencies: Whenever possible, create generic dependencies that can be reused across multiple endpoints. This reduces code duplication and simplifies maintenance.
- Keep dependencies lightweight: Dependencies should be fast and lightweight to avoid slowing down your request processing. Avoid including heavy computations or blocking operations in dependencies.
- Use sub-dependencies judiciously: While sub-dependencies are powerful, they can also make your code harder to follow if overused. Ensure that your dependency hierarchies remain manageable.
Conclusion
Mastering dependencies in FastAPI is crucial for building scalable, efficient, and maintainable web applications. By understanding how to effectively leverage dependencies and sub-dependencies, you can significantly enhance the modularity and reusability of your code. Remember to start with simple dependencies, gradually moving towards more complex structures as needed, and always aim to keep your dependencies organized and lightweight. With the insights and examples provided in this guide, you're now well-equipped to navigate the depths of FastAPI's dependency management system. Happy coding!
As you continue to develop your FastAPI skills, consider revisiting this guide and experimenting with different dependency patterns and structures. The flexibility and power of FastAPI's dependency system can unlock new possibilities for your web applications, making them more robust and easier to maintain.
Recent Posts
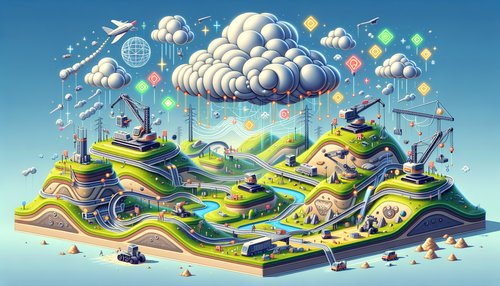
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
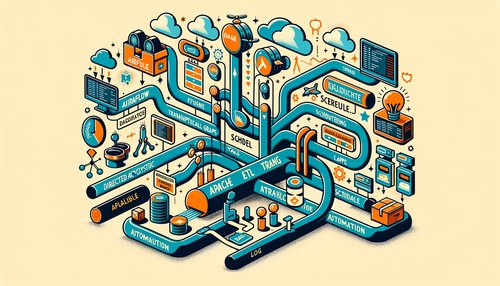
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow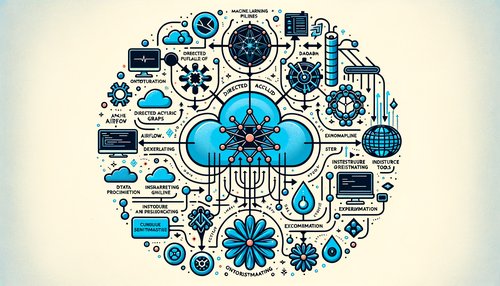
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow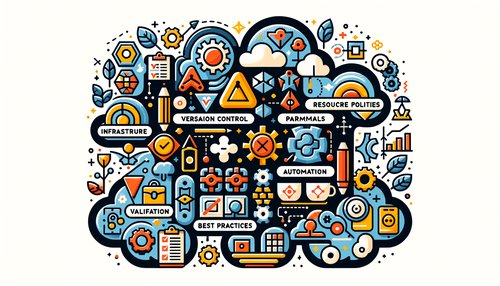
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
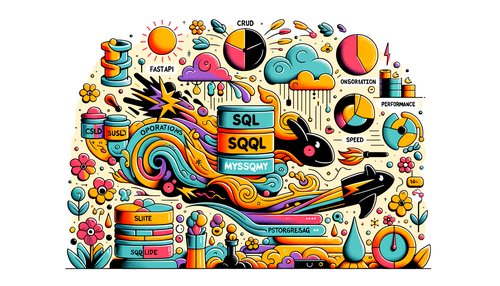