Mastering FastAPI: The Ultimate Guide to Effortlessly Handling Form Data Like a Pro!
Welcome to the ultimate guide where we dive deep into the world of FastAPI, focusing on one of its most practical and essential features: handling form data. Whether you're developing a web application that requires user input or building an API that interacts with web forms, mastering form data handling can significantly streamline your development process. In this comprehensive guide, we'll cover everything from the basics of form data handling in FastAPI to advanced techniques that will make you a pro in no time. Get ready to elevate your FastAPI skills and create more efficient, secure, and user-friendly applications.
Understanding Form Data in FastAPI
Before we jump into the technicalities, it's crucial to grasp what form data is and why it's important in web development. Form data represents the information that users input into a form on a webpage, which is then sent to the server for processing. FastAPI, being a modern and fast web framework for building APIs with Python 3.7+, provides a straightforward and efficient way to handle form data, ensuring that your applications are not only responsive but also secure.
Setting Up Your FastAPI Environment
First things first, ensure that your FastAPI environment is set up. If you haven't done so, install FastAPI and Uvicorn, an ASGI server, to run your application. This can be done using pip:
pip install fastapi uvicorn
With the installation out of the way, you're ready to start coding your app.
Handling Basic Form Data
Handling form data in FastAPI is remarkably simple thanks to its built-in form parsing capabilities. To handle form data, you can use FastAPI's Form
class. Here's a basic example of how to use it:
from fastapi import FastAPI, Form
app = FastAPI()
@app.post("/submit-form/")
async def handle_form(username: str = Form(...), password: str = Form(...)):
return {"username": username, "password": password}
This example demonstrates a simple API endpoint that processes a form with a username and password. The Form
class is used to specify that these values should be extracted from the form data.
Advanced Form Handling Techniques
While handling basic form data is straightforward, FastAPI also supports more advanced techniques that can be incredibly useful for complex applications.
Using Pydantic Models for Form Data
One powerful feature of FastAPI is its integration with Pydantic models, which can be used to define more complex data structures for your form data. This approach not only makes your code cleaner but also adds an extra layer of validation to your form data. Here's an example:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
@app.post("/items/")
async def create_item(item: Item):
return item
In this example, a Pydantic model is used to define the expected structure and types of the form data. FastAPI automatically validates the incoming data against this model.
File Uploads with Form Data
FastAPI makes handling file uploads alongside form data a breeze. To upload files, you can use FastAPI's File
and UploadFile
classes. Here's how:
from fastapi import FastAPI, File, Form, UploadFile
app = FastAPI()
@app.post("/upload/")
async def handle_upload(file: UploadFile = File(...), notes: str = Form(...)):
return {"filename": file.filename, "notes": notes}
This snippet shows an endpoint that accepts a file and additional form data (notes). FastAPI provides a convenient way to access metadata about the file (like its filename) and the content of the form fields.
Securing Form Data
While handling form data efficiently is crucial, ensuring the security of this data is equally important. FastAPI provides several tools and practices to help secure your form data, including:
- Data Validation: By using Pydantic models, you can ensure that incoming form data adheres to a specific schema and contains valid data types.
- HTTPS: Always use HTTPS to encrypt data in transit, protecting sensitive information from being intercepted.
- CSRF Protection: Consider using CSRF tokens to protect against Cross-Site Request Forgery attacks, ensuring that only legitimate requests are processed by your API.
Conclusion
Mastering form data handling in FastAPI doesn't have to be daunting. By understanding the basics, leveraging advanced features like Pydantic models and file uploads, and implementing security best practices, you can enhance the functionality and security of your web applications. FastAPI's simplicity and efficiency in handling form data allow you to focus on building feature-rich applications without getting bogged down by backend complexities. Embrace these techniques, and you'll be handling form data like a pro in no time!
Remember, the key to mastering FastAPI, or any technology, is continuous practice and exploration. Don't hesitate to experiment with different features and approaches to find what works best for your specific use case. Happy coding!
Recent Posts
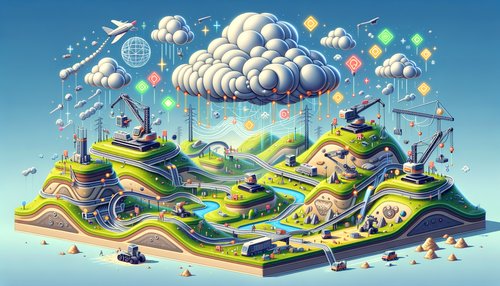
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
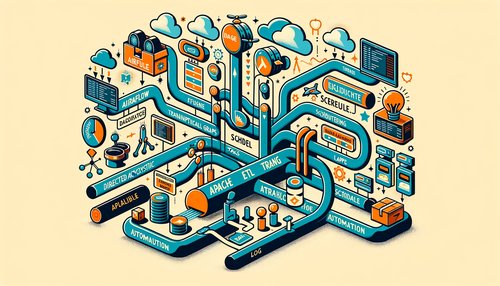
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow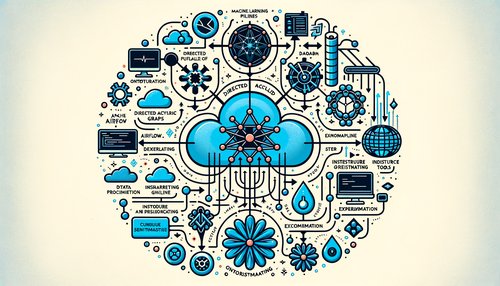
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow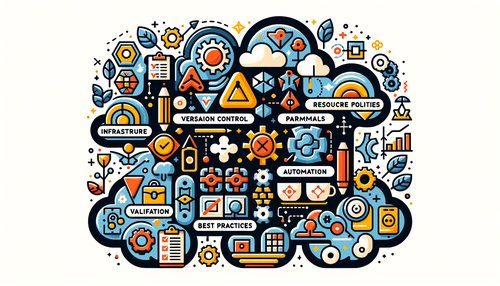
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
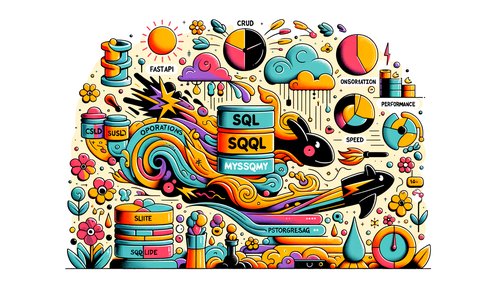